How to Get Selected Radio Button Value in JavaScript
-
Get Radio Element Value Using
document.querySelector()
in JavaScript -
Get Radio Element Value by
getElementsByName()
in JavaScript
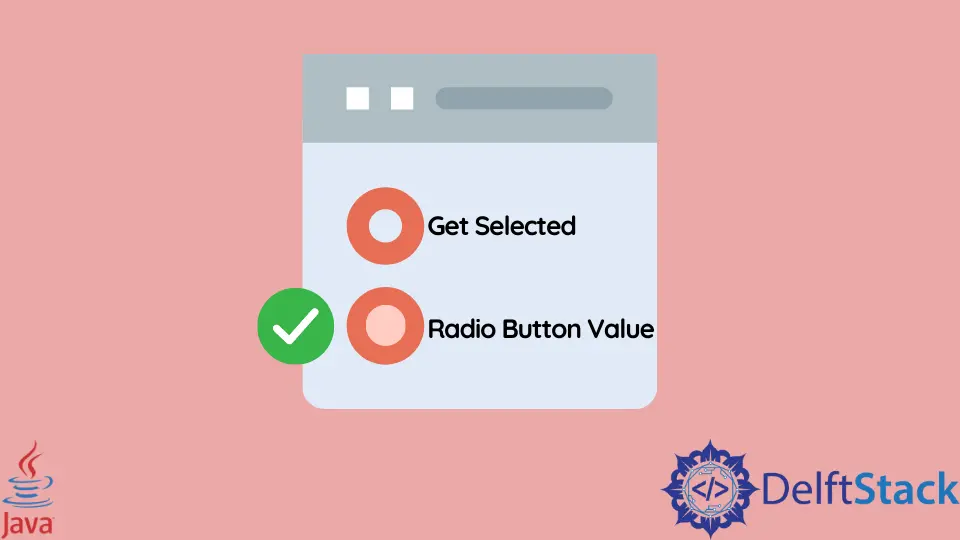
A radio button is an HTML input element that specifies a wide variety of options from which the user can choose any option.
In this article, we will learn how to get the value of this HTML element into JavaScript.
Get Radio Element Value Using document.querySelector()
in JavaScript
It is a basic document
technique provided by JavaScript and returns the Objects/NodeList part whose selector
matches the desired selector
. The only difference between querySelector()
and querySelectorAll()
is that the single-matching item object is returned first, then all of the matching item’s objects. If an invalid selector
is passed, a SyntaxError
is returned.
Syntax:
document.querySelector($selector);
The selector
is a required parameter that specifies the class’s match condition or identifier. This string must be a valid CSS select string. It returns the first matching element object that satisfies the selector
condition in the DOM.
For more information, read the documentation of the querySelector
method.
Example code:
<label for="gender">Gender: </label>
<input type="radio" name="gender" value="Male">Male
<input type="radio" name="gender" value="Female" checked="checked">Female
<button type="button" onclick="console.log(document.querySelector('input[name=gender]:checked').value)">
Click to check
</button>
In the code above, we have defined two input elements with the values Male
and Female
. When we access the document with querySelector()
, it finds all nodes whose name attribute matches gender and whose checked attribute is set to true. Since this is an object, we can directly extract the value. If you need to find more than one matching element, you can use querySelectorAll()
and iterate through the array, and print all the values. The output of the code above will look like this:
Output:
Female
Get Radio Element Value by getElementsByName()
in JavaScript
This is a basic document
technique provided by JavaScript and returns the Objects/NodeList part whose name
attribute matches the desired name. This NodeList represents an array of nodes accessible using an index, and this index starts with 0
like any other array.
Syntax
document.getElementsByName(name);
name
is a required parameter that specifies the name
of an HTML attribute that must match. If any matching elements are found, it returns the object of the matching DOM element; otherwise, it returns null
.
The only difference between getElementsByName
and querySelectorAll
is that getElementsByName
first selects only those elements whose specified name attribute matches the given name. In contrast, querySelectorAll
can use any selector, which provides it with much more flexibility.
For more information, read the documentation of the getElementById
method.
Example code:
<label for="gender">Gender: </label>
<input type="radio" name="gender" value="Male" checked="checked">Male
<input type="radio" name="gender" value="Female">Female
const radios = document.getElementsByName('gender');
for (var i = 0; i < radios.length; i++) {
if (radios[i].checked) {
console.log(radios[i].value);
break;
}
}
In the code above, we have defined two input elements with the values Male
and Female
. When we access the document with getElementsByName()
, it finds all the nodes whose name attribute matches the name and returns NodeList. Since this is an array, we can extract the first matching element with [0]
. If more than one matching item is found, you can browse the array and print all the values. The output of the code above will look like this:
Output:
Male
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn