How to Get Element Based on the Class Name in JavaScript
-
Get an Element Based on Its CSS Class Name With Javascript
.getElementsByClassName()
Function -
Get Element Based on CSS Class Name With Javascript
.querySelector()
Function -
Get Multiple HTML Elements Based on CSS Class Name With the
.querySelectorAll()
Function
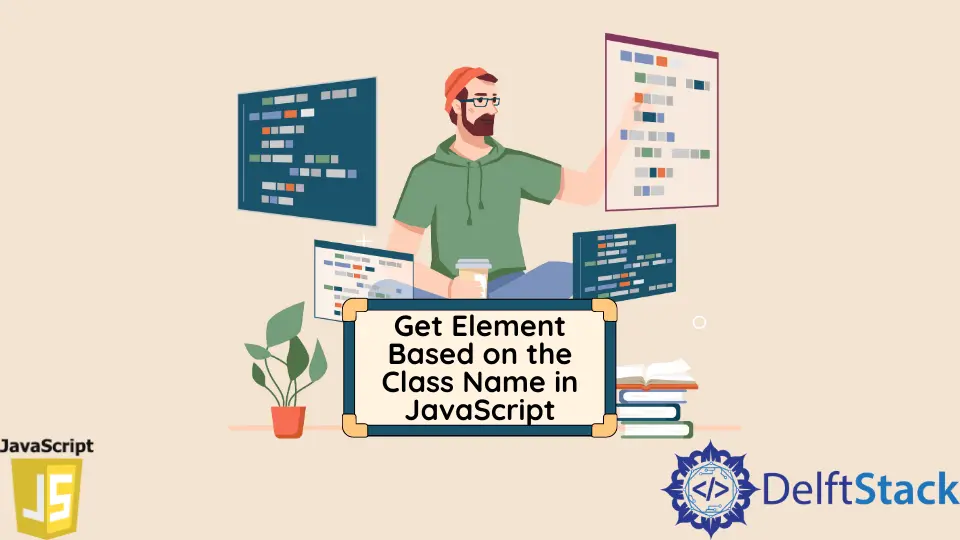
We may need to query an element from the DOM to change its style dynamically at the run time. Javascript has various inbuilt functions that can serve our purpose. The famous one out of these is the .getElementById()
. But .getElementById()
returns only one element, the first element, whose HTML id
matches the parameter passed into the function. The .getElementById()
function approach may be cumbersome if we are to change styles, of multiple elements, at the same time. It will involve adding multiple ids to the HTML elements and querying them one at a time. Can we do it in a better way? The following section elaborates on ways to get elements from the DOM based on its CSS class name.
Get an Element Based on Its CSS Class Name With Javascript .getElementsByClassName()
Function
Javascript has an inbuilt method .getElementsByClassName()
which enables us to query for more than one HTML elements based on their CSS class name. It is a method available in the Document
interface and is usually used on the it like document.getElementsByClassName()
. The function will search the document (the DOM) for all elements with the CSS class name specified in the function parameters.
As per the MDN Docs, the function returns the live collection of HTML elements. It means that the elements returned by the getElementsByClassName()
function may be modified at run time if there are pieces of code modifying the DOM elements directly.
getElementsByClassName
Syntax
let el = document.getElementsByClassName(cssClassName);
The cssClassName
should be a string representing the desired CSS class. If there are multiple CSS classes, we can mention them separated by spaces (like css-class1 css-class2
etc.)
getElementsByClassName
Arguments
The function takes one parameter, the CSS class name. In some cases, we may require a combination of CSS class names to select an element. In such scenarios, we can pass multiple CSS class names separated by spaces.
Example
Let us refer to the following piece of code to understand the functioning.
<div class="bold">Element 1: Bolder text</div>
<div class="green">Green text</div>
<div class="bold">Element 2: Bolder text</div>
window.onload = function() {
let els = document.getElementsByClassName('bold');
console.log(els);
}
Output:
HTMLCollection(2) [div.bold, div.bold]
The above code will log a collection of HTML elements in the console. The Google chrome console will show an output as shown above. Writing the document.getElementsByClassName("bold")
code inside the window.onload
ensures that the code runs only once the HTML has been rendered. Note that unlike .getElementById()
function, the getElementsByClassName()
function retruns an array of HTML elements. We cannot directly work on the element, output by the getElementsByClassName()
as we do for .getElementById()
. If we are to change the bold
style in the above code to normal for the first element, we can use the following code.
let els = document.getElementsByClassName('bold');
els[0].style.fontWeight = '100';
Get Element Based on CSS Class Name With Javascript .querySelector()
Function
getElementsByClassName()
is a widely used method and is restricted to selecting HTML elements based on their CSS class name. Javascript has another inbuilt function available in Document
interface, the querySelector()
, which is more generic in nature. It can be used for querying element based on its CSS class name. This functionality is just a subset of the actual capabilities of the querySelector()
. The Javascript querySelector()
function is comparable to .getElementById()
as it returns the first element that satisfies the selection parameter passed in its arguments.
.querySelector()
Syntax
The syntax is simillar to that of .getElementsByClassName()
.
let el = document.querySelector('.cssQuerySelector');
.querySelector()
Parameters
The querySelector()
accepts a string data type as a parameter representing the css query selector
. The same way that we use the CSS selectors to apply particular styles to the selected elements. It includes #<id>
to select a HTML element based on its id, .<css-class-name>
to select an element based on its CSS style class name and even a combination of the two, like #<id>.<css-class-name>
, can be used to select an element.
Example
The following code explains the usage of .querySelector()
.
<div class="bold">Element 1: Bolder text</div>
<div class="green">Green text</div>
<div class="bold">Element 2: Bolder text</div>
window.onload = function() {
let els = document.querySelector('.bold');
console.log(els);
}
Output:
<div class="bold">Element 1: Bolder text</div>
The output is as it appears in the google chrome browser console. Note that the .querySelector()
function returns just one HTML element unlike the .getElementsByClassName()
which returns a collection of them. Hence, if we are to process it, we can directly apply the changes to it. For instance, if we are to change the font of the selected element to normal, we can achieve it using the following code.
window.onload = function() {
let els = document.querySelector('.bold');
els.style.fontWeight = '100';
}
Get Multiple HTML Elements Based on CSS Class Name With the .querySelectorAll()
Function
As the name hints, the .querySelectorAll()
function in Javascript returns a collection of HTML elements that satisfy the selection criteria. Just like the .querySelector()
, the querySelectorAll()
accepts the CSS query selector string as its parameter. It returns a static list of HTML elements, which are not live. It behaves similar to .getElementsByClassName()
as it returns multiple HTML elements queried based on it, but is not limited to it. Just like the querySelector()
, we can use the .querySelectorAll()
to get elements based on their ids or the CSS class names. Just like we select elements with the Query Selectors in the CSS style sheets.
.querySelectorAll()
Syntax
document.querySelectorAll('<css-selector-string>');
.querySelectorAll()
Parameters
The function accepts a CSS query string as its only input parameter. To select elements based on id, we must use the parameter #<id>
. And for selecting based on CSS class name, it should be .<css-class-name>
. For combination of the two we can use #<id>.<css-class-name>
format.
Example
<div class="bold">Element 1: Bolder text</div>
<div class="green">Green text</div>
<div class="bold">Element 2: Bolder text</div>
let els = document.querySelectorAll('.bold');
console.log(els);
Output:
NodeList(2)
0: div.bold
1: div.bold
The output is an array of type node list
. We can process these nodes further by selecting them from the array and changing their CSS class values as per the requirement. An example is as depicted by the following code. The line els[0].style.fontWeight = "100";
will make the first selected HTML node to normal font-weight.
<div class="bold">Element 1: Bolder text</div>
<div class="green">Green text</div>
<div class="bold">Element 2: Bolder text</div>
let els = document.querySelectorAll('.bold');
els[0].style.fontWeight = '100';
console.log(els);
Output:
NodeList(2)
0: div.bold
1: div.bold