How to Implement Fade-In Div in JavaScript
- Understanding the Fade-In Effect
- Method 1: Simple Fade-In Using JavaScript
- Method 2: Fade-Out Effect Before Fading In
- Method 3: Continuous Fade-In and Fade-Out Loop
- Conclusion
- FAQ
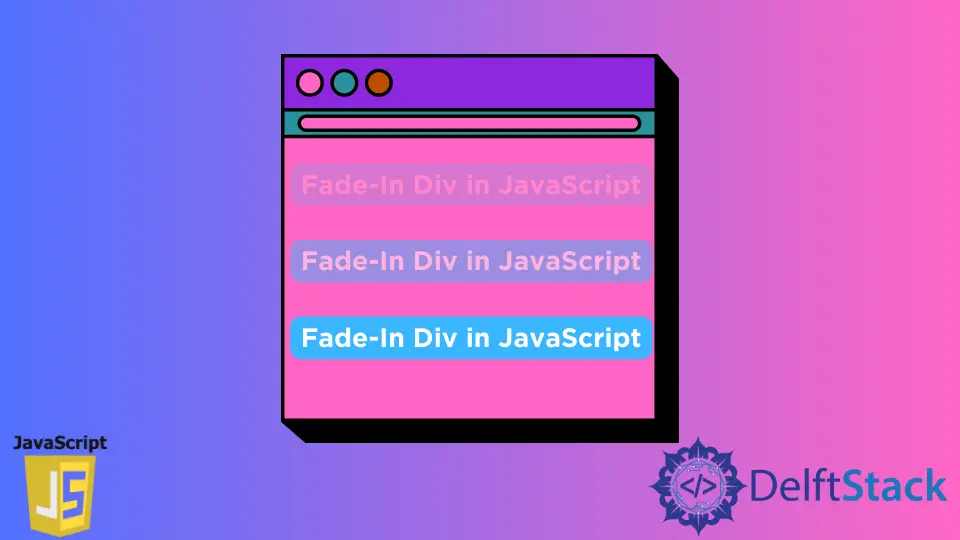
In today’s post, we’ll learn how to fade in and out a div using pure JavaScript. This technique is not only visually appealing but also enhances user experience by making transitions smoother. Whether you’re looking to improve your website’s aesthetics or simply want to add some flair to your web applications, implementing a fade-in effect is a great skill to have.
In this article, we’ll explore various methods to achieve this effect, providing you with clear examples and detailed explanations. By the end, you’ll be equipped to create engaging fade-in animations that captivate your audience.
Understanding the Fade-In Effect
Before diving into the code, it’s important to understand what a fade-in effect is. Essentially, it’s a gradual transition where an element becomes visible over a set period. This can be particularly useful when displaying content that you want to draw attention to, such as alerts, notifications, or special offers. The fade-in effect can be achieved using CSS transitions, JavaScript animations, or a combination of both. Here, we will focus on how to implement this using pure JavaScript.
Method 1: Simple Fade-In Using JavaScript
Let’s start with a straightforward approach to implement a fade-in effect using JavaScript. This method involves manipulating the element’s opacity over time.
function fadeIn(element, duration) {
element.style.opacity = 0;
element.style.display = "block";
let start = performance.now();
function animate(time) {
let elapsed = time - start;
element.style.opacity = Math.min(elapsed / duration, 1);
if (elapsed < duration) {
requestAnimationFrame(animate);
}
}
requestAnimationFrame(animate);
}
const myDiv = document.getElementById("myDiv");
fadeIn(myDiv, 1000);
Output:
The div with id "myDiv" will fade in over one second.
In this code, we define a function called fadeIn
, which takes two parameters: the element to fade in and the duration of the fade effect in milliseconds. We first set the element’s opacity to 0 and change its display property to “block” so it becomes visible. The animate
function is called using requestAnimationFrame
, which ensures smooth animations. As time progresses, we gradually increase the opacity until it reaches 1, making the element fully visible.
Method 2: Fade-Out Effect Before Fading In
Now, let’s explore how to create a fade-out effect before fading in. This can be useful when you want to transition between different pieces of content smoothly.
function fadeOut(element, duration, callback) {
element.style.opacity = 1;
let start = performance.now();
function animate(time) {
let elapsed = time - start;
element.style.opacity = Math.max(1 - elapsed / duration, 0);
if (elapsed < duration) {
requestAnimationFrame(animate);
} else {
callback();
}
}
requestAnimationFrame(animate);
}
function fadeInWithFadeOut(element, duration) {
fadeOut(element, duration, () => {
element.style.display = "block";
fadeIn(element, duration);
});
}
const myDiv = document.getElementById("myDiv");
fadeInWithFadeOut(myDiv, 1000);
Output:
The div with id "myDiv" will fade out and then fade in over one second.
In this example, we first define a fadeOut
function that reduces the opacity of the element to 0 over the specified duration. Once the fade-out is complete, we call the callback function, which is responsible for triggering the fade-in effect. The fadeInWithFadeOut
function orchestrates this process, ensuring a smooth transition between the two effects. This method creates a more dynamic experience, allowing users to focus on the changing content.
Method 3: Continuous Fade-In and Fade-Out Loop
For an engaging user interface, you might want to create a continuous fade-in and fade-out loop. This can be particularly effective for image sliders or rotating banners.
function fadeLoop(element, duration) {
fadeIn(element, duration);
setTimeout(() => {
fadeOut(element, duration, () => {
setTimeout(() => fadeLoop(element, duration), 1000);
});
}, duration);
}
const myDiv = document.getElementById("myDiv");
fadeLoop(myDiv, 1000);
Output:
The div with id "myDiv" will continuously fade in and out every three seconds.
In this implementation, we define a fadeLoop
function that first fades the element in. After the specified duration, we set a timeout to fade it out. Once the fade-out is complete, we set another timeout to restart the loop after a brief pause. This creates a seamless transition that keeps the user engaged and can be particularly useful for displaying multiple messages or images in a carousel format.
Conclusion
Implementing a fade-in effect using pure JavaScript is a valuable skill that can significantly enhance the user experience on your website. Whether you choose a simple fade-in, a combination of fade-in and fade-out, or a continuous loop, these techniques will help you create engaging and visually appealing interfaces. With a few lines of code, you can add a touch of elegance to your web applications. So go ahead, experiment with these methods, and see how they can elevate your projects!
FAQ
-
What is a fade-in effect?
A fade-in effect is a gradual transition that makes an element become visible over time. -
Can I use CSS for fade-in effects?
Yes, CSS transitions can also be used to create fade-in effects, but this article focuses on pure JavaScript methods.
-
How can I customize the duration of the fade effect?
You can change the duration parameter in the functions to control how long the fade effect lasts. -
Is it possible to create a fade-in effect for multiple elements?
Yes, you can loop through multiple elements and apply the fade-in function to each one. -
Can I combine fade-in effects with other animations?
Absolutely! You can combine fade-in effects with other CSS or JavaScript animations to create more complex interactions.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn