Fastest Factorial Program in JavaScript
- Factorial Program Using Recursion in JavaScript
- Factorial Program Using Iteration in JavaScript
- Fastest Factorial Program in JavaScript
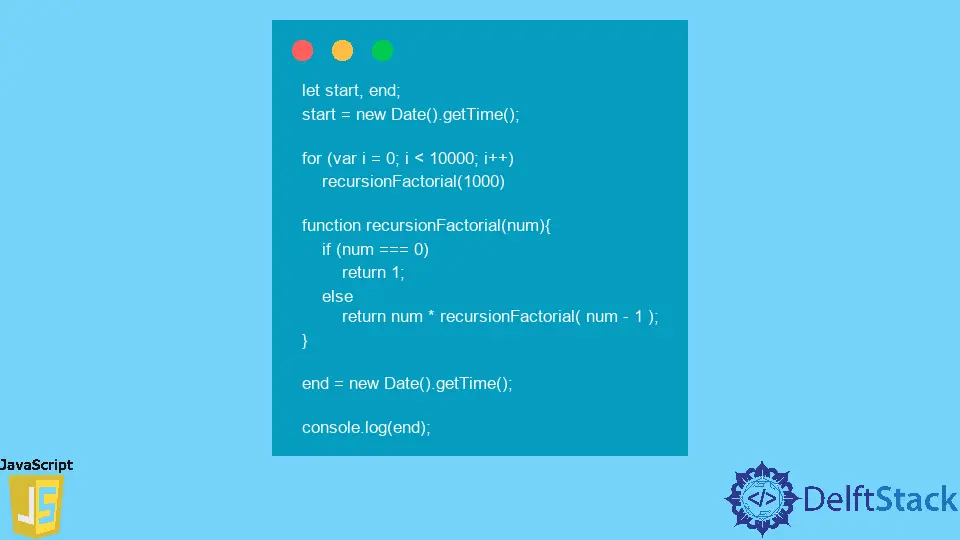
The factorial program is used to find the factors of a given number. For example, the factors of the number 8 are 1, 2, 4, and 8. The factors of both the numbers 0 and 1 are always 1. Similarly, every number has some factorial numbers.
There are two ways of writing a factorial program in JavaScript, one way is by using recursion, and another way is by using iteration. We will call both of these programs 1000 times using the for()
loop, and every time we call this program, we will find the factorial of the number 1000.
Let’s see both the ways in detail, and later we will see which one is the fastest factorial program.
Factorial Program Using Recursion in JavaScript
The recursive method is one way of writing the factorial program. In recursion, we call the same function again and again with some base condition. The base condition makes sure that we don’t get into an infinite loop.
To check the time it takes to execute the recursive program, we use the getItem()
method of the Date
object. The start
variable will store the time when the program starts its execution, and the end
will store the time when the program completes its execution.
As we know, the factorial of a number zero is 1
. So, we will make this our base condition, and as soon as a number becomes zero, it will return 1
. Else we will keep finding the factorial of that number by calling the same factorial function again and again till we reach 0
. The implementation of the factorial program using recursion is as shown below.
let start, end;
start = new Date().getTime();
for (var i = 0; i < 10000; i++) recursionFactorial(1000)
function recursionFactorial(num) {
if (num === 0)
return 1;
else
return num * recursionFactorial(num - 1);
}
end = new Date().getTime();
console.log(end);
Output:
1627808767415
The output shown above is the time when the recursive program completes its execution which is also stored in the end
variable. The time is in milliseconds and is printed in the console window of the browser.
Factorial Program Using Iteration in JavaScript
Another way of writing a factorial program is by using iteration. In iteration, we use loops such as for
, while
, or do-while
loops.
Here also we will be checking the time it takes to execute the iterative program by using the getItem()
method of the Date
object. The start2
variable will store the time when the program starts its execution, and the end2
will store the time when the iterative program completes its execution.
In the iterationFactorial()
function, we initially set the counter to 1
and then use a for loop to find the factors by incrementing the counter
variable. In the end, we return the value of the counter
variable.
let start2, end2;
start2 = new Date().getTime();
for (var i = 0; i < 10000; i++) iterationFactorial(1000);
function iterationFactorial(num) {
var counter = 1;
for (var i = 2; i <= num; i++) counter = counter * i;
return counter;
}
end2 = new Date().getTime();
console.log(end2);
Output:
1627808727136
The output shown above is the time when the program ends the iteration program completes its execution which is also stored in the end2
variable. The time is in milliseconds and is printed in the console window of the browser.
Fastest Factorial Program in JavaScript
Now that we have seen both the recursion and iteration program for finding factorial of a number. Let’s now check the final result (i.e, time taken to run both of these programs) to see which one of these two factorial programs is faster.
We have already stored the start and end time for the recursion program in the start
and end
variables and the time for the iteration program inside the start2
and end2
variables. Now we have to subtract the end time from the start time to get the resultant time in milliseconds.
let res1 = end - start;
let res2 = end2 - start2;
console.log('res1: ' + res1 + ' res2: ' + res2)
Output:
res1: 1626 res2: 27
The final results show that the recursion program takes much more time than the iteration program. Therefore, In JavaScript, the iteration factorial program is the fastest. This output may vary depending upon the system on which you will be executing this program.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn