How to Extract the First Child of an Element in JavaScript
-
Extract the First Child of an Element in JavaScript Using
Node.firstChild
-
Extract the First Child of an Element in JavaScript Using
Node.childNodes
-
Extract the First Child of an Element in JavaScript Using
Element.children
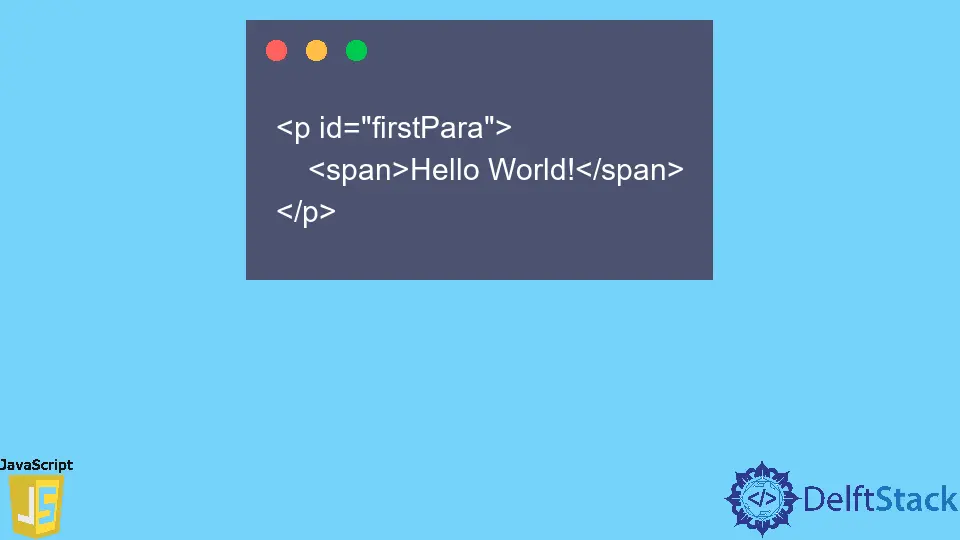
In today’s post, we’ll learn to extract the first child of an element in JavaScript.
Extract the First Child of an Element in JavaScript Using Node.firstChild
Node.firstChild
returns the first child of the node in the tree, or null
if the node has no children. This is the read-only firstChild
property of the Node interface.
Syntax:
Node.firstChild
For example, we have a paragraph tag that shows the Hello World!
text inside it in another span tag.
HTML code:
<p id="firstPara">
<span>Hello World!</span>
</p>
JavaScript code:
const firstPara = document.getElementById('firstPara');
console.log(firstPara.firstChild);
Output:
"#text"
When you run the above code in any browser, the console of the browser displays #text
. This is because a text node is inserted, by default, to keep white space between the end-of-paragraph tag and the opening-of-span tags.
Each white space automatically creates a #text
node, from a single space to multiple spaces, newlines, tabs, etc.
To avoid the text node issue, either you can remove the white space from the source or use Element.firstElementChild
to return only the first element node.
You can find more information about the firstChild
in the documentation here.
Extract the First Child of an Element in JavaScript Using Node.childNodes
Node.childNodes
returns an active NodeList of the child nodes of the specified element, with the index 0 assigned to the first child node. This is the read-only childNodes
property of the Node interface.
Child nodes contain items, texts, and comments.
The elements of the node collection are objects, not strings. To retrieve data from node objects, use their properties.
For example, to get the name of the first child node, you can use elementNodeReference.childNodes[0].nodeName
.
ChildNodes
, by default, includes all child nodes, both elements and non-elements. It returns an active NodeList containing the children of the node.
You can find more information about childNodes
in the documentation here.
For example, we have a paragraph tag that shows Hello World!
text inside it in another span tag.
HTML code:
<p id="firstPara">
<span>Hello World!</span>
</p>
JavaScript code:
const firstPara = document.getElementById('firstPara');
console.log(firstPara.childNodes[0]);
Output:
"#text"
Similar to the previous section, by default, a text node is inserted to keep white space between the end of the paragraph tag and the opening of the span tags.
Extract the First Child of an Element in JavaScript Using Element.children
The Element.children
property returns a live HTML collection containing all the children of the element it was called on.
The sole difference between Element.children
and Node.childNodes
is that Element.children
contains only element nodes whereas Node.childNodes
get all child nodes, including non-element nodes like text and comments.
An HTML collection is an active, ordered collection of the DOM elements children of the node. You can access each child node of the collection using the item()
method.
You can find more information about the children in the documentation here.
For example, we have a paragraph tag that shows the Hello World!
text inside it in another span tag.
HTML code:
<p id="firstPara">
<span>Hello World!</span>
</p>
JavaScript code:
const firstPara = document.getElementById('firstPara');
console.log(firstPara.children[0]);
Output:
"Hello World!"
When you run the above code in any browser, the console of the browser will display "Hello World!"
. This is because this property returns only DOM elements of the calling node.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn