JavaScript Destructor
- Use Destructuring With Objects
- Use Destructuring With Arrays
- Use Destructuring With Arrays and Objects Simultaneously
- Conclusion
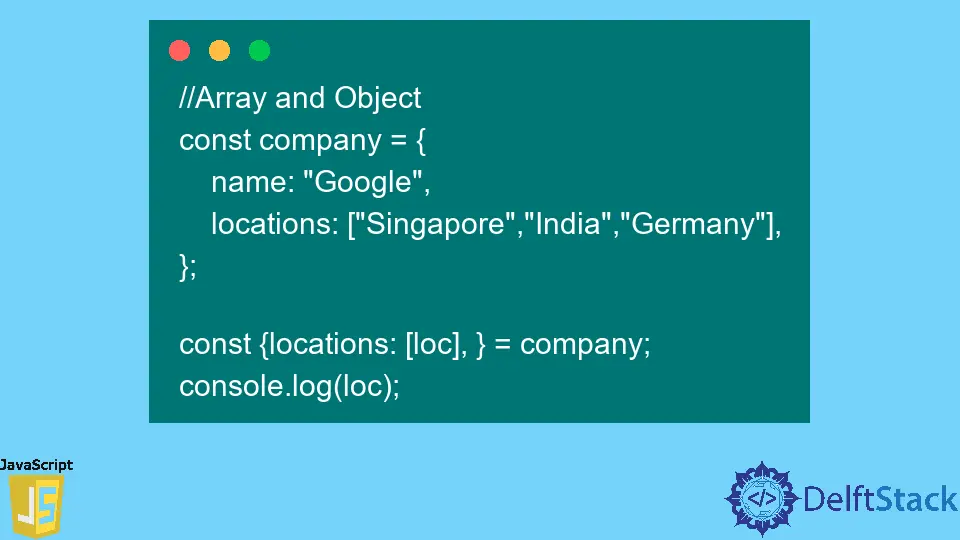
This article mainly focuses on JavaScript de-structuring, one of the best and most helpful features of ES6. De-structuring is a JavaScript statement that enables the unpacking of array items or object properties into separate variables, arrays, objects, nested objects, and assignments to variables that allow for all the extraction of data.
At the same time, we will go through some examples and see how to use de-structuring for arrays, objects, and both.
Use Destructuring With Objects
// object
const employee = {
id: 001,
name: 'John Oliver',
age: 24,
department: 'Marketing',
address: {
city: 'Colombo',
country: 'Sri Lanka',
},
};
The employee
object in the JS code mentioned earlier is visible. On the right side, this employee has basic properties like id
, name
, age
, and department
. A console with some data is displayed.
// object
const employee = {
id: 001,
name: 'John Oliver',
age: 24,
department: 'Marketing',
address: {
city: 'Colombo',
country: 'Sri Lanka',
},
};
const name = employee.name;
const age = employee.age;
console.log(name);
console.log(age);
So, we will first see how we can use the de-structuring with objects.
If we want to access the property of this employee, then what should we do? We can write a name; it changes the employee.name
.
It changes to employee.age
if we want to access the age.
Suppose we print the name and age to the console with console.log(name);
and console.log(age);
. If we run the code, you can see that we get the name
and the age
consoled on output.
Here, you can see that we have done the same thing repeatedly. We have repeated the name
twice, age
twice, and the employees.
But ES6 gives you some best features for de-structuring assignments when you don’t need to repeat yourself because it can be done in small syntax and concisely.
Let’s look at how we can fulfill this task with de-structuring. Firstly, we write a variable.
Here, this constant is equal to the employee
.
// object
const employee = {
id: 001,
name: 'John Oliver',
age: 24,
department: 'Marketing',
address: {
city: 'Colombo',
country: 'Sri Lanka',
},
};
//const name = employee.name;
//const age = employee.age;
const { name, age } = employee;
console.log(name);
console.log(age);
But on the left side, we create an object syntax then we can write property names that we want to access from the employee
object. So, we also want to access the name
and the age
.
When we execute the above code, we still receive the same output or result.
Here, we aren’t repeating employee.name
or employee.age
. We are just using those property names directly.
We can also do one more thing if you don’t want to use the name
as the property and you want to give any other name.
// object
const employee = {
id: 001,
name: 'John Oliver',
age: 24,
department: 'Marketing',
address: {
city: 'Colombo',
country: 'Sri Lanka',
},
};
//const name = employee.name;
//const age = employee.age;
const { name: fullName, age } = employee;
console.log(fullName);
console.log(age);
Then, we can write a colon and use fullName
, and we will still get the same result. And one cool thing about this de-structuring is that you can use the default parameter here.
// object
const employee = {
id: 001,
age: 24,
department: 'Marketing',
address: {
city: 'Colombo',
country: 'Sri Lanka',
},
};
// const name = employee.name;
// const age = employee.age;
const {name: fullName = 'Lora Noah ', age} = employee;
console.log(fullName);
console.log(age);
Suppose we want to use a default parameter of Lora Noah
. You can see that now we have used the default parameter.
Let’s look at this employee
object that doesn’t contain the property name. In that case, it will use the default value, Lora Noah.
Suppose we want to de-structure a nested object like the employee
. We have to know the city where the particular employee resides and add to the address which we currently know.
Then, we can also do that. We need to have an address first.
If we console this address, it gives us an object. Let’s try running the code.
// object
const employee = {
id: 001,
age: 24,
department: 'Marketing',
address: {
city: 'Colombo',
country: 'Sri Lanka',
},
};
// const name = employee.name;
// const age = employee.age;
const {address} = employee;
console.log(address);
The output will be received like the one shown below. But we only want the city of it.
We don’t want the complete object here because we can change it. What can we de-structure? We can write about the city
.
Remove the address, and then use the city
for the console.
// object
const employee = {
id: 001,
age: 24,
department: 'Marketing',
address: {
city: 'Colombo',
country: 'Sri Lanka',
},
};
// const name = employee.name;
// const age = employee.age;
const {address: {city}} = employee;
console.log(city);
Here is the output we get.
Then, we get Colombo
as the output when you can use the de-structuring in functions. The function we build should be called displayEmployee
.
That function takes an employee
and then needs to console.log()
. We use a string literal for the console.
We write the employee name as ${name}
, and the age is ${age};
for the console.
// object
const employee = {
id: 001,
age: 24,
department: 'Marketing',
address: {
city: 'Colombo',
country: 'Sri Lanka',
},
};
function displayEmployee(employee) {
console.log(`The employee name is ${name} and age is ${age}`);
}
displayEmployee(employee);
If we want to print those names and then usually what to do? The employee.name
and employee.age
of the console.
// object
const employee = {
id: 001,
age: 24,
department: 'Marketing',
address: {
city: 'Colombo',
country: 'Sri Lanka',
},
};
function displayEmployee(employee) {
console.log(
`The employee name is ${employee.name} and age is ${employee.age}`);
}
displayEmployee(employee);
In this code run, you can see that we get the employee.name
as undefined.
That’s why we removed the name
property from the object of an employee
. Now, we can get the name John and the age 24
.
The code and the expected output after executing the code are shown below.
// object
const employee = {
id: 001,
name: 'John',
age: 24,
department: 'Marketing',
address: {
city: 'Colombo',
country: 'Sri Lanka',
},
};
function displayEmployee(employee) {
console.log(
`The employee name is ${employee.name} and age is ${employee.age}`);
}
displayEmployee(employee);
Output:
But we can also do the function’s de-structure; therefore, we require the age to de-structure this function. We need to have the name.
You don’t need to maintain any sequence here. If explained in other words, the name
and the age
can be written.
Since there is no set order, we can alter it as necessary.
// object
const employee = {
id: 001,
name: 'John',
age: 24,
department: 'Marketing',
address: {
city: 'Colombo',
country: 'Sri Lanka',
},
};
function displayEmployee(age, name) {
console.log(
`The employee name is ${employee.name} and age is ${employee.age}`);
}
displayEmployee(employee);
If we run this code, then we still get the same output.
Use Destructuring With Arrays
We have to create an array. We want to have access to any of the array’s values.
The words apple
, mango
, banana
, and pineapple
are written in an array. We use the variable fruits to represent that array.
The first element, apple
, is written at index 0, and the second element, mango
, is used at index 1.
And we display the console.log(apple)
and console.log(mango)
.
// Array
const fruits = ['apple', 'mango', 'banana', 'pineapple'];
const apple = fruits[0];
const mango = fruits[1];
console.log(apple);
console.log(mango);
The output is shown below.
We get apple
and mango
as a result. But if we want to de-structuring the array, we put it in writing.
To de-structuring arrays, this constant will be set to be similar to the fruits array.
Here, an array is required, and the values are used for the array. If we enter fruit1
and fruit2
, the apple
of the first index is to be the output.
// Array
const fruits = ['apple', 'mango', 'banana', 'pineapple'];
// const apple = fruits[0];
// const mango = fruits[1];
const [fruit1, fruit2] = fruits;
console.log(fruit1);
console.log(fruit2);
Therefore, the apple
will be assigned the value of fruit1
, and the mango
can be assigned the value of fruit2
. The term fruit1
above relates to the array’s first value.
The second value of the array is called fruit2
. which is the array’s second value, mango
.
If you want to skip mango, You need the third value, banana. We will thus execute an empty.
We won’t have any reference then it skips the second value of the array. It gives us the third value.
// Array
const fruits = ['apple', 'mango', 'banana', 'pineapple'];
// const apple = fruits[0];
// const mango = fruits[1];
const [fruit1, , fruit2] = fruits;
console.log(fruit1);
console.log(fruit2);
Then, we can get apple
and banana
as output.
Use the Rest Operator for Destructuring With Arrays
You can also use the rest operator for de-structuring. The rest operator can be used if you want the array’s first three items and the remaining values.
We can enter the rest operator and run a console.log()
to obtain the remaining array that contains pineapple
.
In the code, we can see the operator’s behavior.
// Array
const fruits = ['apple', 'mango', 'banana', 'pineapple'];
// const apple = fruits[0];
// const mango = fruits[1];
const [fruit1, , fruit2, ...rest] = fruits;
console.log(fruit1);
console.log(fruit2);
console.log(rest);
This is the output.
Here is how you will do the de-structuring part in the arrays.
Use Destructuring With Arrays and Objects Simultaneously
Firstly, we can write an array and object. This array of groceries can be seen in the below code.
// Array and Object
const groceryList = [
{item: 'Apples', price: 25, category: 'fruits'},
{item: 'Mangoes', price: 35, category: 'fruits'},
{item: 'Tomatoes', price: 15, category: 'vege'},
{item: 'Milk', price: 20, category: 'misc'},
{item: 'Bread', price: 12, category: 'misc'},
{item: 'Eggs', price: 18, category: 'misc'},
];
console.log(groceryList[0].item);
If you want to access the Apples
, usually you will do a console.log(groceryList[0].item);
. Then you can see the Apples
as the output.
Using de-structuring, we can write this. We write constant and write Apples
in it.
These Apples
will be equal to the groceryList
. First, we have an array if we want to go through the first element of the array.
// Array and Object
const groceryList = [
{item: 'Apples', price: 25, category: 'fruits'},
{item: 'Mangoes', price: 35, category: 'fruits'},
{item: 'Tomatoes', price: 15, category: 'vege'},
{item: 'Milk', price: 20, category: 'misc'},
{item: 'Bread', price: 12, category: 'misc'},
{item: 'Eggs', price: 18, category: 'misc'},
];
// console.log(groceryList[0].item);
const [apple] = groceryList;
console.log(apple);
Here’s the output.
The array is complete here, but the object is not needed. We only want the item in the object for de-structuring.
If we de-structure the object, it’s always taken with the property. And there is no property as the apple.
Therefore, we need to assign the property this way. Look at the code below.
You can try different components for the variable as the apple. But if you use it directly as an item, you can write it.
// Array and Object
const groceryList = [
{item: 'Apples', price: 25, category: 'fruits'},
{item: 'Mangoes', price: 35, category: 'fruits'},
{item: 'Tomatoes', price: 15, category: 'vege'},
{item: 'Milk', price: 20, category: 'misc'},
{item: 'Bread', price: 12, category: 'misc'},
{item: 'Eggs', price: 18, category: 'misc'},
];
// console.log(groceryList[0].item);
const [{item}] = groceryList;
console.log(item);
The output says Apples
.
If you want the output to be Tomatoes
, skip the first two steps, and then there is nothing to worry about. You can see the Tomatoes
in the array as the third.
// Array and Object
const groceryList = [
{item: 'Apples', price: 25, category: 'fruits'},
{item: 'Mangoes', price: 35, category: 'fruits'},
{item: 'Tomatoes', price: 15, category: 'vege'},
{item: 'Milk', price: 20, category: 'misc'},
{item: 'Bread', price: 12, category: 'misc'},
{item: 'Eggs', price: 18, category: 'misc'},
];
// console.log(groceryList[0].item);
const [, , {item}] = groceryList;
console.log(item);
This is the output of the above-implemented code chunk.
Suppose we also want the other items after receiving the Tomatoes
. To do that, we must apply the same rest operator and add a console.log()
to the console.
Once we have that, we can retrieve the remaining array of objects.
// Array and Object
const groceryList = [
{item: 'Apples', price: 25, category: 'fruits'},
{item: 'Mangoes', price: 35, category: 'fruits'},
{item: 'Tomatoes', price: 15, category: 'vege'},
{item: 'Milk', price: 20, category: 'misc'},
{item: 'Bread', price: 12, category: 'misc'},
{item: 'Eggs', price: 18, category: 'misc'},
];
// console.log(groceryList[0].item);
const [, , {item}, ...rest] = groceryList;
console.log(item);
console.log(rest);
The output can be seen below.
That’s how powerful the de-structuring is. Let’s examine an illustration of a different approach where an object contains an array.
We want the location Singapore
to pick. So to get the location of Singapore, we can do console.log(company.(locations[0]))
.
// Array and Object
const company = {
name: 'Google',
locations: ['Singapore', 'India', 'Germany'],
};
console.log(company.locations[0]);
We can get the value/output as Singapore. See it below.
We want to achieve this using de-structuring. We can write a constant that’s equal to the object that is a company.
To do a de-structuring for an object, we use curly braces. In curly braces, we use the property name as the location.
From the locations, we must access the first value of the index 0. Therefore, we can write an array here and use loc
for location.
// Array and Object
const company = {
name: 'Google',
locations: ['Singapore', 'India', 'Germany'],
};
const {
locations: [loc],
} = company;
console.log(loc);
And we want to print this loc
; therefore, we use console.log(loc)
. Then, we can get Singapore
as the output.
Suppose we want the result as India
; therefore, we skip the first value. Then, we can get output that value (India).
// Array and Object
const company = {
name: 'Google',
locations: ['Singapore', 'India', 'Germany'],
};
const {
locations: [, loc],
} = company;
console.log(loc);
Here, you can see the output.
There is the last use case in which we discuss the de-structuring. Here, we use a constant as new Users
.
const Users = [
['John', 'Oliver'],
['James', 'Smith'],
['Mary', 'Elizabeth'],
];
[{
firstName: 'John',
lastName: 'Oliver',
},
{
firstName: 'James',
lastName: 'Smith',
},
{
firstName: 'Mary',
lastName: 'Elizabeth',
},
];
These Users
have nesting arrays. If we want the result to be the output below, we can see which contains the objects and inside the object.
We want a key-value pair: firstName as John
and lastName as Oliver
. Using the de-structuring, we can do it very easily.
We use a higher-order array function here, which is the map. So, we create a constant with the Users
object, which takes the Users
.
It will give you the first user. With the first user, you can return the object you want; here, we will do firstName
.
This firstName will equal the user index of zero, and then for the lastName, you will do a user index of 1.
After that console, the Users
object using console.log(UsersObj)
;.
const Users = [
['John', 'Oliver'],
['James', 'Smith'],
['Mary', 'Elizabeth'],
];
[{
firstName: 'John',
lastName: 'Oliver',
},
{
firstName: 'James',
lastName: 'Smith',
},
{
firstName: 'Mary',
lastName: 'Elizabeth',
},
];
const UserObj = Users.map((User) => {
return { firstName: User[0], lastName: User[1] };
});
console.log(UserObj);
Here, we get the firstName
and lastName
of the three objects.
Regarding the de-structuring, what can we do here? From the array, we can assign the first value as the firstName
and the second value as the lastName
.
const Users = [
['John', 'Oliver'],
['James', 'Smith'],
['Mary', 'Elizabeth'],
];
[{
firstName: 'John',
lastName: 'Oliver',
},
{
firstName: 'James',
lastName: 'Smith',
},
{
firstName: 'Mary',
lastName: 'Elizabeth',
},
];
const UserObj = Users.map(([firstName, lastName]) => {
return { firstName: firstName, lastName: lastName };
});
console.log(UserObj);
Here also, the same result comes as the output above.
But we can do one more thing as ES6 has enhanced object literals. We can do that for the key, and the value is the same.
We can remove it. However, with what remained, we can still get the same results.
const Users = [
['John', 'Oliver'],
['James', 'Smith'],
['Mary', 'Elizabeth'],
];
[{
firstName: 'John',
lastName: 'Oliver',
},
{
firstName: 'James',
lastName: 'Smith',
},
{
firstName: 'Mary',
lastName: 'Elizabeth',
},
];
const UserObj = Users.map(([firstName, lastName]) => {
return { firstName, lastName };
});
console.log(UserObj);
That’s how we can use the de-structuring.
Conclusion
In this article, we have discussed what de-structuring is and how to perform it in JavaScript. It’s useful when you work with languages like React.
Even if you don’t work with React, you can still write clean code.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.