How to Detect Clipboard Data in JavaScript
- Understanding Clipboard Events
- Accessing Clipboard Data
- Handling Cross-Browser Compatibility
- Best Practices for Clipboard Data Handling
- Conclusion
- FAQ
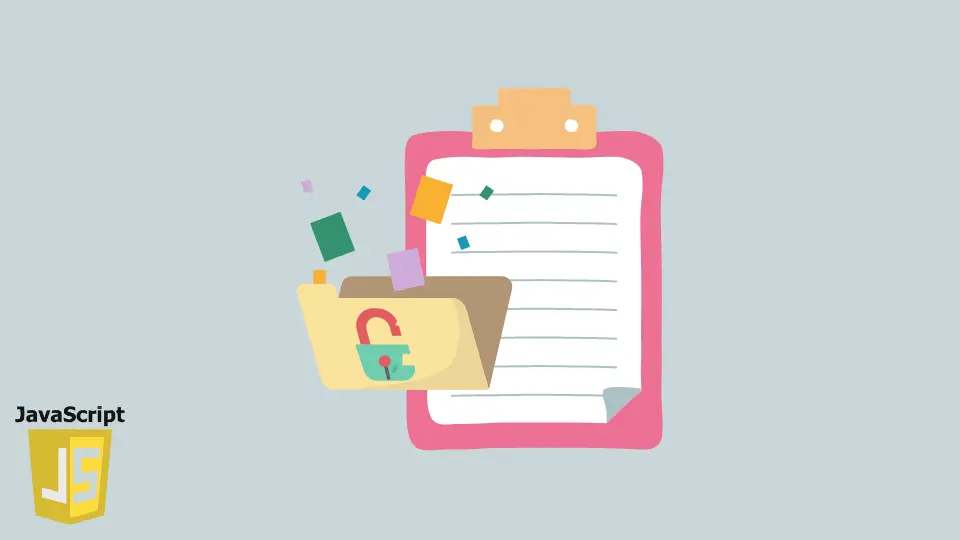
In today’s digital landscape, clipboard interactions have become a common part of user experience on the web. Whether you’re building a text editor, a form, or a dynamic application, detecting clipboard data during paste events can enhance functionality significantly.
In this article, we’ll explore how to effectively detect clipboard data in JavaScript when a user pastes content into a web application. We’ll cover the necessary event listeners, how to access clipboard data, and best practices to ensure a smooth user experience. Let’s dive into the world of clipboard events and enhance our JavaScript applications!
Understanding Clipboard Events
Clipboard events in JavaScript are triggered when a user interacts with the clipboard, typically through actions like copy, cut, and paste. The most relevant event for our purpose is the paste
event. This event is fired when the user pastes content into an editable element, such as a text input or a content-editable div.
To start detecting clipboard data, you need to add an event listener for the paste
event. This can be done using JavaScript’s addEventListener
method. Here’s a simple example:
document.getElementById('myInput').addEventListener('paste', function(event) {
const clipboardData = event.clipboardData || window.clipboardData;
const pastedData = clipboardData.getData('Text');
console.log('Pasted data: ', pastedData);
});
In this code, we attach a paste
event listener to an input field with the ID myInput
. When the user pastes data, we retrieve the clipboard data using event.clipboardData
and log the pasted text to the console. This approach is straightforward and effectively captures the clipboard data.
Output:
Pasted data: <the text that was pasted>
By utilizing clipboard events, you can create applications that respond dynamically to user input, enhancing interactivity and user engagement.
Accessing Clipboard Data
Once you’ve set up the event listener, the next step is to access the clipboard data effectively. The ClipboardEvent
provides a clipboardData
property, which contains all the data that was copied to the clipboard. You can extract various types of data, including text, images, and HTML content.
Here’s how you can access and display different types of clipboard data:
document.getElementById('myInput').addEventListener('paste', function(event) {
const clipboardData = event.clipboardData || window.clipboardData;
const pastedText = clipboardData.getData('text/plain');
const pastedHTML = clipboardData.getData('text/html');
console.log('Pasted Text: ', pastedText);
console.log('Pasted HTML: ', pastedHTML);
});
In this example, we retrieve both plain text and HTML content from the clipboard. The getData
method takes a MIME type as an argument, allowing you to specify which type of data you want to access. This flexibility is essential for applications that need to handle rich content.
Output:
Pasted Text: <the plain text that was pasted>
Pasted HTML: <the HTML content that was pasted>
By capturing different formats, you can build more sophisticated applications that handle various data types seamlessly, improving the user experience.
Handling Cross-Browser Compatibility
While modern browsers support clipboard events, it’s essential to ensure that your application works consistently across different environments. Older browsers may not fully support the clipboardData
API, so it’s crucial to implement fallback mechanisms.
Here’s an example that checks for compatibility and provides a fallback:
document.getElementById('myInput').addEventListener('paste', function(event) {
let pastedData;
if (event.clipboardData) {
pastedData = event.clipboardData.getData('text/plain');
} else {
pastedData = window.clipboardData.getData('Text');
}
console.log('Pasted data: ', pastedData);
});
In this code, we first check if event.clipboardData
is available. If not, we fall back to using window.clipboardData
, which is an older method primarily supported in Internet Explorer. This ensures that your application remains functional for users on legacy browsers.
Output:
Pasted data: <the pasted data>
By implementing cross-browser compatibility, you can ensure a broader reach and a better user experience, regardless of the browser being used.
Best Practices for Clipboard Data Handling
When working with clipboard data, adhering to best practices is crucial for maintaining a smooth user experience. Here are some important guidelines:
-
User Feedback: Always provide feedback to users when they paste data. This could be in the form of a message or visual cues indicating that the paste was successful.
-
Data Validation: Validate the pasted data to ensure it meets your application’s requirements. For instance, if you’re expecting a specific format, check for that before processing the data.
-
Security Considerations: Be cautious when handling clipboard data, especially if your application processes sensitive information. Always sanitize input to prevent potential security vulnerabilities.
-
Accessibility: Ensure that your clipboard interactions are accessible. Consider users who may rely on keyboard shortcuts or screen readers.
By following these best practices, you can create a more robust and user-friendly application that effectively handles clipboard interactions.
Conclusion
Detecting clipboard data in JavaScript is a powerful feature that can significantly enhance user interaction within your web applications. By leveraging the paste
event, accessing various data formats, ensuring cross-browser compatibility, and adhering to best practices, you can build applications that respond intelligently to user input. As you implement these techniques, remember to test your application thoroughly to ensure a seamless experience for all users. Happy coding!
FAQ
-
How can I detect clipboard data in JavaScript?
You can detect clipboard data by adding an event listener for thepaste
event and accessing the clipboard data throughevent.clipboardData
. -
What types of data can I retrieve from the clipboard?
You can retrieve plain text, HTML content, and other types of data using thegetData
method on the clipboard data object. -
How do I ensure compatibility across different browsers?
Check for the availability ofevent.clipboardData
, and if it’s not available, usewindow.clipboardData
as a fallback for older browsers. -
What should I do if the pasted data doesn’t meet my requirements?
Implement data validation to ensure the pasted content matches your application’s expected format before further processing. -
Are there any security concerns with clipboard data?
Yes, always sanitize and validate clipboard data to prevent potential security vulnerabilities, especially when handling sensitive information.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn