How to Check if Element Exists in DOM in JavaScript
-
Use the
getElementById()
to Check the Existence of Element inDOM
-
Use the
getElementsByClassName
to Check the Existence of an Element inDOM
-
Use the
getElementsByName
to Check the Existence of an Element inDOM
-
Use the
getElementsByTagName
to Check the Existence of an Element inDOM
-
Use the
contains()
to Check the Existence of an Element in the VisibleDOM
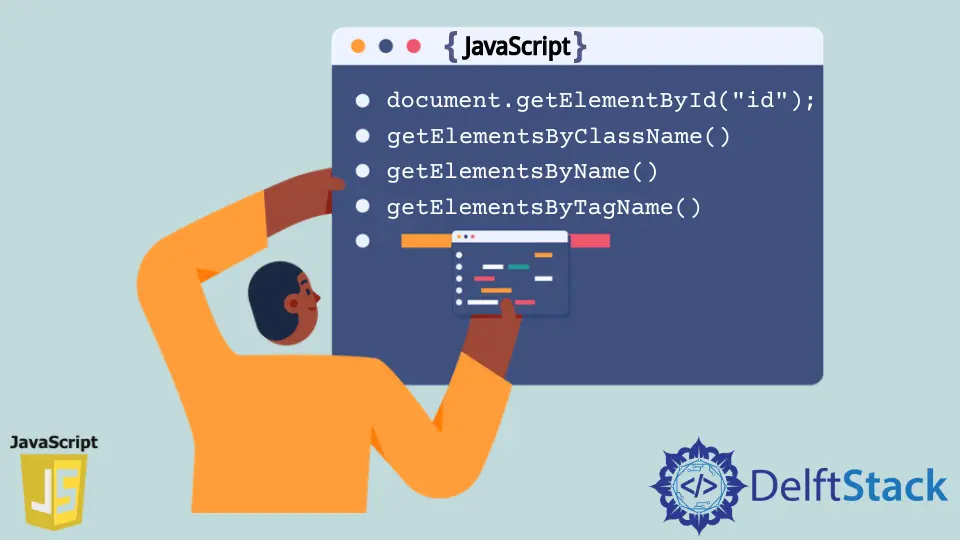
This article will explain how we can check the existence of an element in the DOM
with different methods.
Use the getElementById()
to Check the Existence of Element in DOM
We can use the function getElementById
to verify if an element exists in DOM
using the element’s Id
. In the following example we will verify that the element <a id="Anchor Id" href="#">Click Here</a>
exists in DOM
.
<html>
<body>
<a id="Anchor Id" href="#">Click Here</a>
<script>
var testData = document.getElementById("Anchor Id");
console.log(testData);
</script>
</body>
</html>
Output:
<a id="Anchor Id" href="#">Click Here</a>
If we want to return the value as boolean instead of the returned element we can add a !!
before our document.getElementById("Anchor Id");
.
<html>
<body>
<a id="Anchor Id" href="#">Click Here</a>
<script>
var testData = !!document.getElementById("Anchor Id");
console.log("Is Not null?",testData);
</script>
</body>
</html>
Output:
Is Not null? true
Use the getElementsByClassName
to Check the Existence of an Element in DOM
Similar to that we use the getElementById()
function to find the element using its Id
, we also have many other functions that perform the same operation by with deffrernt criteria like getElementsByClassName()
, getElementsByName()
and getElementsByTagName()
.
The Function getElementsByClassName()
is used to find the element in the DOM
using its class name. An example to the class name value is ClassExample
in element <a class="ClassExample"></a>
. It will return one or more elements if any element is found or null
if the element does not exist.
Let’s see the following example of the getElementByClassName()
function:
<html>
<body>
<a class="ClassExample" href="#">Click Here for the class example </a>
<script>
var classname = document.getElementsByClassName("ClassExample");
var classnameExists = !!document.getElementsByClassName("ClassExample");
console.log("Element :",classname);
console.log("Is Not null ? ",classnameExists);
</script>
</body>
</html>
Output:
Element : HTMLCollection [a.ClassExample]
Is Not null ? true
We can also use the sign !!
before the function getElementsByClassName()
to type cast the result to boolean value, where it will return true
if it have any value, and false
if it will return null
.
Use the getElementsByName
to Check the Existence of an Element in DOM
The function getElementsByName()
is used to find any element in DOM
by its name, The Name
of the element has value of ElementNameHolder
in the element <a name="ElementNameHolder"></a>
. Let’s see the following example:
<html>
<body>
<a class="ClassExample" name="ElementNameHolder" href="#">Click Here for the class example </a>
<script>
var EleName = document.getElementsByName("ElementNameHolder");
var elementNameExists = !!document.getElementsByName("ElementNameHolder");
console.log("Element :",EleName);
console.log("Is Not null ? ",elementNameExists);
</script>
</body>
</html>
Output:
Element : NodeList [a]
Is Not null ? true
Use the getElementsByTagName
to Check the Existence of an Element in DOM
The function getElementsByTagName()
can return all elements with the specified tagName
in DOM
. The return of the function can be one or more elements or null
if no element is found.
The following example shows how to check if the required element exists in DOM
or not.
<html>
<body>
<exampleTag>
<a name="ElementNameHolder1" href="#">Click Here for the tag name example 1 </a>
</exampleTag>
<exampleTag>
<a name="ElementNameHolder2" href="#">Click Here for the tag name example 2 </a>
</exampleTag>
<script>
var EleTagName = document.getElementsByTagName("exampleTag");
console.log("Element 1 :",EleTagName[0].innerHTML);
console.log("Element 2 :",EleTagName[1].innerHTML);
</script>
</body>
</html>
Output:
Element 1 :
<a name="ElementNameHolder1" href="#">Click Here for the tag name example 1 </a>
Element 2 :
<a name="ElementNameHolder2" href="#">Click Here for the tag name example 2 </a>
Use the contains()
to Check the Existence of an Element in the Visible DOM
If we want to check the existence of an element in the visible DOM
, we can use document.body.contains()
that will search in the visible DOM
for the element we send in its first argument.
The function contains()
recieves only one node, so when we retrieve nodes using any of the functions document.getElementsByTagName
or document.getElementsByName
, we need to select only one element to send, as those functions return all elements found that match the selection criteria.
<html>
<body>
<exampleTag>
<a name="ElementNameHolder2" href="#">Tag, Name, Id in visible DOM example 1</a>
<a id="ElementId1" href="#">Tag, Name, Id in visible DOM example 2</a>
</exampleTag>
<exampleTag>
<a name="ElementNameHolder2" href="#">Tag, Name, Id in visible DOM example 3</a>
<a id="ElementId2" href="#">Tag, Name, Id in visible DOM example 4</a>
</exampleTag>
<script>
var EleTagName = document.getElementsByTagName("exampleTag");
let myVar1 = document.body.contains(document.getElementsByTagName("exampleTag")[0]);
let myVar2 = document.body.contains(document.getElementsByName("ElementNameHolder2")[0]);
let myVar3 = document.body.contains(document.getElementById("ElementId2"));
console.log("Element 1 :",EleTagName[0].innerHTML);
console.log("Element 2 :",EleTagName[1].innerHTML);
</script>
</body>
</html>
Element 1 :
<a name="ElementNameHolder2" href="#">Tag, Name, Id in visible DOM example 1</a>
<a id="ElementId1" href="#">Tag, Name, Id in visible DOM example 2</a>
Element 2 :
<a name="ElementNameHolder2" href="#">Tag, Name, Id in visible DOM example 3</a>
<a id="ElementId2" href="#">Tag, Name, Id in visible DOM example 4</a>