How to Change CSS Classes in JavaScript
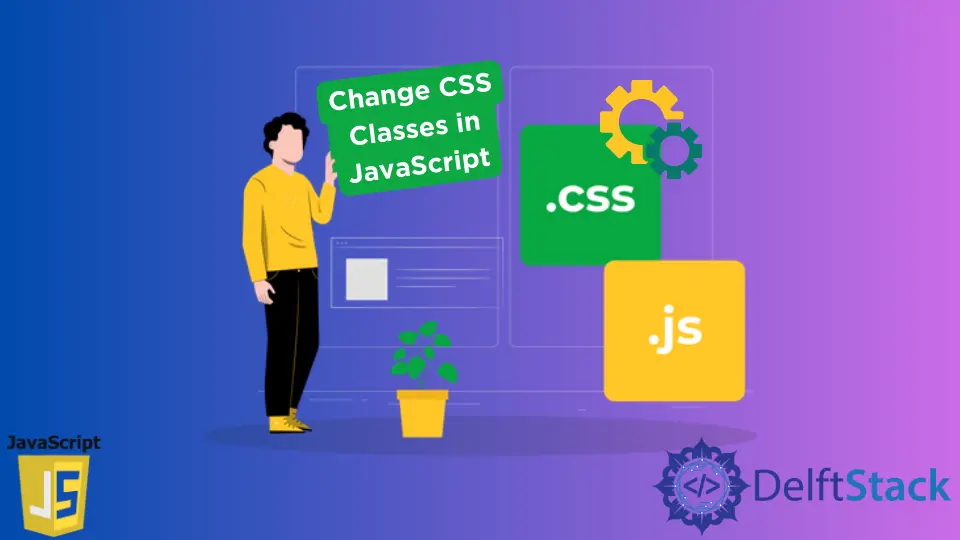
Whenever we are working with the DOM elements inside the JavaScript, there are times and situations where we need to provide some interactions to the HTML elements. For Example, changing the background colors of an element on user click, hiding an element, modifying an element’s appearance, etc. This can most of the time be done by changing the classes.
The JavaScript language provides you with different ways of doing all of this. Let’s see various methods using which we can change the CSS classes from the HTML elements.
Various Ways of Changing CSS Class in JavaScript
Below we have an HTML document that consists of a single div
element inside the body
tag. We also have a style
tag that contains one id and two classes.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
<style>
#dimensions{
width: 500px;
height: 500px;
}
.bg_1{
background-color: crimson;
}
.bg_2{
background-color: teal;
}
</style>
</head>
<body>
<div id="dimensions" class="dimensions bg_1">
<script>
let myDiv = document.getElementById('dimensions');
myDiv.addEventListener("click", function(e) {
});
</script>
</body>
</html>
The id with the name dimensions
contains the width and height. The bg_1
and bg_2
are two classes that have a property of background-color
with crimson
and teal
colors, respectively. The dimensions
and bg_1
are applied to the HTML element inside the body
tag.
From the code, you can see that we have already stored the div
element inside the myDiv
variable by using the document.getElementById()
method and passing that elements id (in this case dimensions
) as a string inside it.
Our aim here is to change the background color of the div
element from crimson to teal by changing the class bg_1
with bg_2
. This will be done only when the user will on the div
element. For this, we have also added the onClick
event using addEventListener()
method on the myDiv
variable inside the JavaScript.
We will be following various ways to achieve this as shown below. All the code which we will write below will go inside the addEventListener()
method under the script
tag.
Using the className
Method
The easiest way of changing a CSS class in JavaScript is by using the className
method. Using this method, you can replace any existing classes already present on the HTML element with some other classes. You can specify all the new classes that you want to add as a string with space separation.
myDiv.className = 'bg_2';
Notice that this will replace all the existing classes present on the element with the new classes.
Using classList.remove
and classList.add
Methods
All the classes which are present on the HTML element can be accessed with the help of the classList
method. Using classList.remove
, you can remove a specific class, and using classList.add
, you can add new classes to the element. In our example, we will first remove the bg_1
class using classList.remove
and then add new class i.e bg_2
using classList.add
as shown below.
myDiv.classList.remove("bg_1");
myDiv.classList.add("bg_2");
This is how we change CSS classes using these methods.
Using the classList.replace()
Method
Another way of changing the CSS class is by using the classList.replace()
method. This method takes two arguments, the first is the existing class that is already present on the element which is want to replace (In this case bg_1
), and the second is the new class that you want to add to the element (In this case bg_2
).
myDiv.classList.replace('bg_1', 'bg_2');
These are some of the methods using which we can change the CSS classes in JavaScript.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn