How to Draw Circle in HTML5 Canvas Using JavaScript
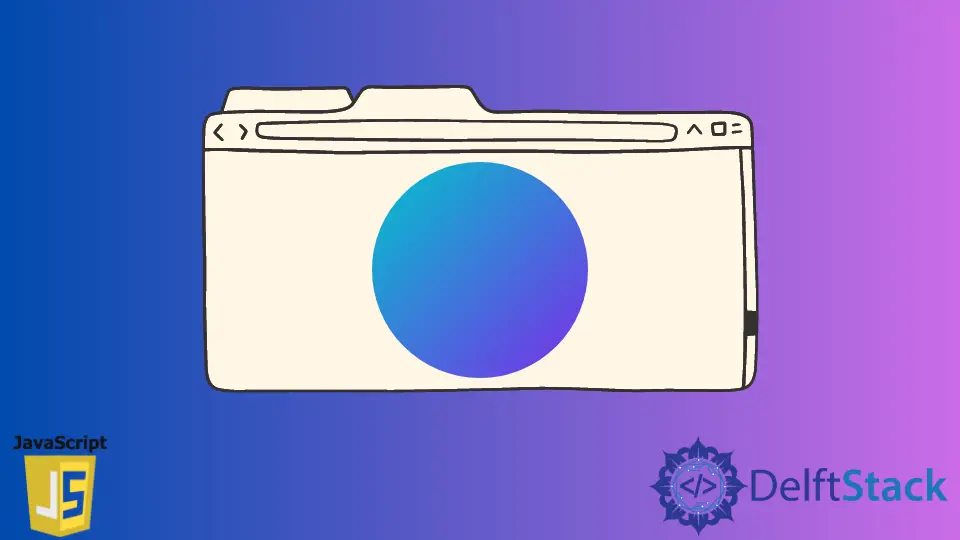
Graphics are an important part of any web application. HTML provides two ways to create graphics, the first is canvas
and another one is SVG
. In today’s post, we are going to learn how to create graphics particularly circles (2 dimensional) in HTML using canvas and JavaScript.
Draw Circle by canvas
in HTML Using JavaScript
Canvas is a default element provided by HTML which is used to draw graphics on web applications. It is nothing but a rectangle area on the page with no border and content. Users can use this rectangle area to draw graphics.
Graphics rendered in canvas are different than regular HTML and CSS styling. The entire canvas with all the graphics it contains is treated as a single dom element.
Methods of canvas
in HTML
getContext()
: It is an in-built method provided bycanvas
that returns the drawing context on canvas depending on thecontextType
. If context identifier is not supported or is already set it will returnnull
. Supported context types are2d
,webgl
,webgl2
, andbitmaprenderer
.beginPath()
: It is an in-built method provided bycanvas
that begins the path or resets the already existing path to draw the graphics.arc()
: It is an in-built method provided bycanvas
that is used to create an arc of the circle on the current path based on the input parameters.fill()
: It is an in-built method provided bycanvas
that is used to fill the current path with the specified color. Users can also specify the region likenonzero
andevenodd
.stroke()
: It is an in-built method provided bycanvas
that is used to outline the current path with the given stroke style.
Syntax of arc
context.arc(
$centerX, $centerY, $radius, $startAngle, $endAngle, $counterclockwise);
Parameters
$centerX
: It is a mandatory parameter that specifies theX
or horizontal co-ordinate/center point of the circle.$centerY
: It is a mandatory parameter that specifies theY
or verticle co-ordinate/center point of the circle.$radius
: It is a mandatory parameter that specifies the radius of the circle. This must be positive.$startAngle
: It is a mandatory parameter that specifies starting angle of an arc in radians measured from the positive x-axis.$endAngle
: It is a mandatory parameter that specifies the ending angle of an arc in radians measured from the positive x-axis. For eg,2 * Math.PI
for a full circle.$counterclockwise
: It is an optional parameter that specifies a boolean value which indicates how a circle will be drawn clockwise or counterclockwise. The default value isfalse
.
Steps to Draw a Circle Using JavaScript Canvas
-
Get the context of the Canvas.
-
Declare the X, Y points & Radius.
-
Set the color & width of a line.
-
Draw the circle.
Example code:
<canvas id="myCanvas" width="500" height="200"></canvas>
<script>
const canvas = document.getElementById('myCanvas');
const context = canvas.getContext('2d');
const centerX = canvas.width / 2;
const centerY = canvas.height / 2;
const radius = 50;
context.beginPath();
context.fillStyle = '#0077aa';
context.strokeStyle = '#0077aa47';
context.lineWidth = 2;
context.arc(centerX, centerY, radius, 0, 2 * Math.PI, false);
context.fill();
context.stroke();
</script>
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn