How to Append HTML Content to the Existing HTML Element Using JavaScript
-
Drawback of Using
InnerHTML
to Add a New HTML Node - Adding HTML Content Without Replacing the DOM Elements
-
Using
appendChild()
to Append HTML Content in JavaScript -
Using
insertAdjacentHTML()
to Add HTML Node
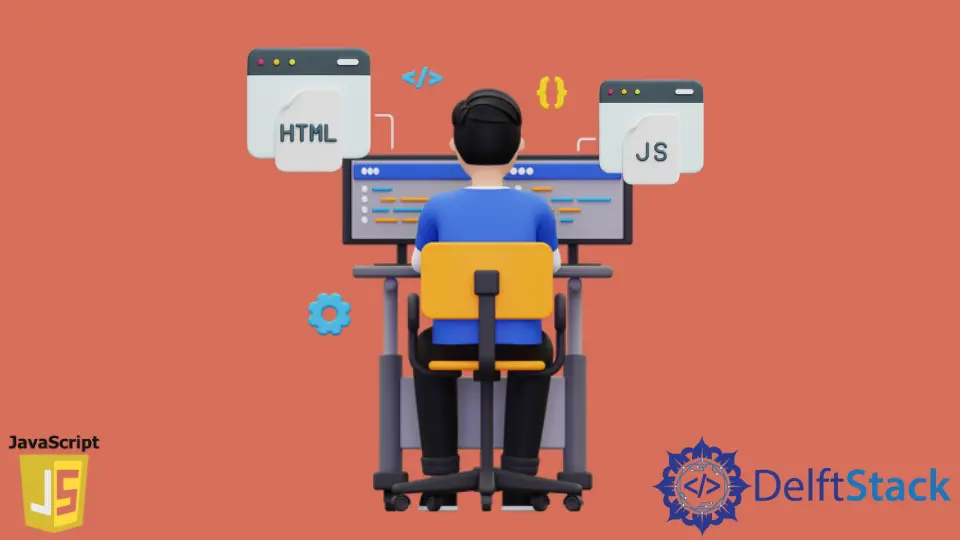
Adding HTML content to a div or another HTML element with javascript is easy. We can set the HTMLElement.innerHTML
property of the element with the new HTML content. But during the process, it will erase off all the dynamic attributes added to it.
Drawback of Using InnerHTML
to Add a New HTML Node
- The
HTMLElement.innerHTML
works by deleting the HTML content inside the element and then adding them back again with the new piece of HTML included in it. In essence, it replaces the HTML content rather than just adding a new one. - When we add a new HTML element to the DOM with the
innerHTML
property, it will remove all the descendant nodes too and then replace them with the new nodes built by parsing the new HTML content (the HTML string that is assigned toinnerHTML
property) and render the entire HTML afresh. - It is not much known that while using
innerHTML
in a project which undergoes a cybersecurity review, the code can get rejected. It is advisable not to useinnerHTMl
directly as it may have a potential security risk (for further details, refer to the MDN link).
Adding HTML Content Without Replacing the DOM Elements
To add a piece of HTML code to our existing DOM, we can use the following inbuilt functions of javascript without having the fear of losing any dynamic content already attached with them.
appendChild()
insertAdjacentHTML()
Using appendChild()
to Append HTML Content in JavaScript
appendChild()
serves the same purpose as that of the innerHTML
. We can use both of these methods to add HTML content to a node. The difference between the two approaches is that the appendChild()
is a function, whereas innerHTML
is a property. appendChild()
will add a node to the existing DOM element instead of replacing the content of DOM as done by the innerHTML
property.
<div>
<div>User Details</div>
<div>
<label>Name:</label>
<input type="text" id="name" placeholder="Name">
</div>
<div id="adress">
<label>Address:</label>
<input type="text" id="contact" placeholder="Address">
</div>
<button id="add_address" onclick="addAddressLine()">Add Address Line</button>
</div>
var lineCount = 0;
addAddressLine = function() {
var i = document.createElement('input');
i.setAttribute('type', 'text');
i.setAttribute('placeholder', 'Address Line ' + ++lineCount);
var addressContainer = document.getElementById('adress');
addressContainer.appendChild(i);
}
We have created a form to capture the name
and address
of the user using the above code. The address usually has more than one line. Hence, we have created a button that adds new input fields when clicked to capture these additional address lines. Following is the process followed for creating a new input field.
- We call the
addAddressLine
function on the click event of theAdd Address Line
button. - In the function, we create HTML
input
dynamically with thedocument.createElement('input')
function. It returns the createdinput
element which stored in thei
variable. - Next we set the
type
andplaceholder
attributes to the createdinput
variable with thesetAttribute()
method. - We have the
input
element ready to be added to the existingdiv
structure. Hence, we query for theaddress
div
using thedocuemnt.getElementById()
which returns the div structure as a whole and then we use theappendChild(i)
function of JavaScript. - The
appendChild(i)
function adds the dynamically createdinput
element held by the variablei
and places it at the end of theaddress
div
. We use thelineCount
to increment the placeholder value each time we add a new input field.
Note
- We can also use the newer method
append()
for appending the created element to the DOM. The javascript methodappend
allows us to use DOMString (simple string texts) as an argument and does not return any value. Beware that the Internet Explorer browser does not support the newerappend()
method. - All the modern web browsers, including the Internet Explorer, support the older
appendChild()
function.
Using insertAdjacentHTML()
to Add HTML Node
The appendChild()
and even the append()
functions are capable of adding new elements to the given HTML node. Both these methods insert the element after the last child. It may not be useful for all scenarios. Especially if we wish to add the HTML node at a specified position in the HTML structure. For such cases insertAdjacentHTML()
function can be used. It takes a couple of parameters, the position and the text in that position.
-
Position
:insertAdjacentHTML
supports four values in the position parameter.beforebegin
: To insert the HTML node before the start of the element.afterbegin
: As the name suggests, this option inserts the element just after the opening tag of the selected node and places it before the first child.beforeend
: This one is similar to theappendChild()
. As the name suggests, thebeforeend
position places the element just after the last child.afterend
: Refers to the position after the target HTML node tag closes.
-
String
: We can insert the HTML in the string format as a second parameter. For example:<div> Hello World!</div>
.
At a glance, the representation is as follows.
<!-- beforebegin -->
<div>
<!-- afterbegin -->
<p>
<!-- beforeend -->
</div>
<!-- afterend -->
Let us look at the same example as we discussed for appendChild()
with the insertAdjacentHTML()
function of JavaScript.
<div>
<div>User Details</div>
<div>
<label>Name:</label>
<input type="text" id="name" placeholder="Name">
</div>
<div id="address">
<label>Address:</label>
<input type="text" id="contact" placeholder="Address">
</div>
<button id="add_address" onclick="addAddressLine()">Add Address Line</button>
</div>
var lineCount = 0;
addAddressLine = function() {
var i = document.createElement('input');
i.setAttribute('type', 'text');
i.setAttribute('placeholder', 'Address Line ' + ++lineCount);
var addressContainer = document.getElementById('adress');
addressContainer.insertAdjacentElement('beforeend', i);
}
In this code, we have used the beforeend
option in the parameter to append the dynamically created input element at the end of the address
div. You can try playing around with the other attributes like the afterend
, beforebegin
, and afterbegin
parameters.