JavaScript Annotation: How Decorators Will Add Annotation Syntax to Classes
- Annotations Before Decorators Proposal
- a Primer on Decorators
- Syntax of a Decorator
- the Evaluation of a Decorator
- Calling Decorators
- an Example of Decorators
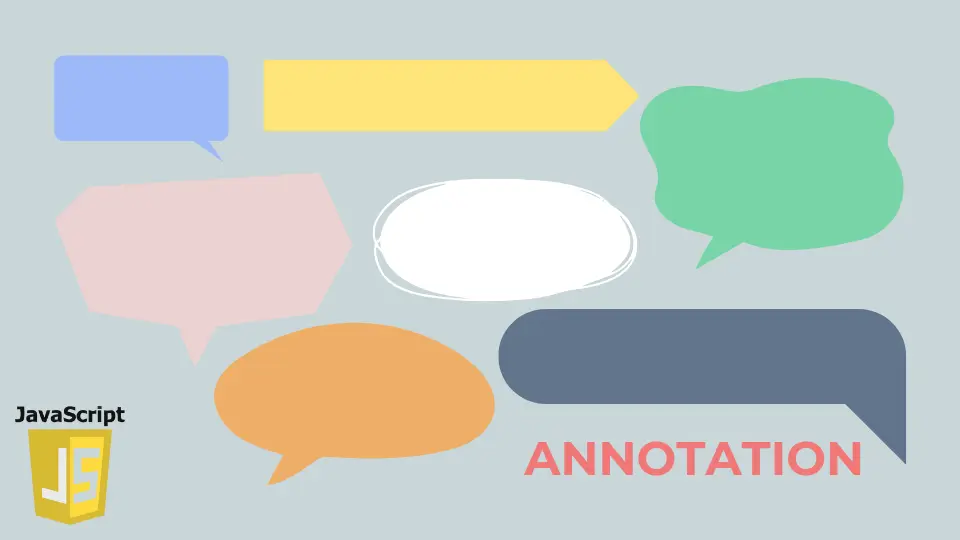
This article explains what annotations look like in current JavaScript. We say annotations because they are not like classical annotations in a language like Java.
However, as of April 24, 2022, Decorators is still a Stage 3 proposal. This means Decorators are not yet available in JavaScript.
However, when they become available, they’ll provide a way to add annotation syntax for class declarations.
Annotations Before Decorators Proposal
Before the proposals for Decorators in JavaScript, the thing closest to an annotation is the "use script"
directive. For example:
(function() {
'use strict';
// code in strict mode
})();
In the code above, the "use strict"
directive causes strict mode execution within the function.
a Primer on Decorators
From the Decorators proposal, decorators are functions that you can use for metaprogramming and adding functionality to a value without changing its external behavior. They’ll work on the following.
- Classes
- Class methods
- Class fields
- Class accessors
- Class auto accessors
After calling a decorator on these elements, a Decorator can do the following:
- A decorator can replace a method with another method or a class with another class.
- Decorators can provide access to the value decorated via accessor functions.
- Decorators can initialize the value that you want to decorate.
Syntax of a Decorator
The Decorators proposal is currently at Stage 3; however, it uses the syntax from Stage 2. Therefore, the following applies to the syntax of a decorator.
-
Their expressions are restricted to a chain of variables. Also, you do property access with dot notation and not square bracket notation.
However, you can use
@(expression)
if you’d like an arbitrary expression. -
You can decorate class expressions and class declarations.
-
Class decorators come after
export
anddefault
.
the Evaluation of a Decorator
The evaluation of a decorator follows the following steps.
- Decorator expressions are evaluated with computed property names.
- Decorators are called functions during class definition. This happens after evaluating the methods but before the prototype and constructor.
- Decorators mutate the constructor and prototypes at once.
Calling Decorators
When you call decorators, they receive two parameters. These parameters are:
- The value you want to decorate. This value is
undefined
in the case of class fields. - A context object that contains information about the value being decorated.
an Example of Decorators
The following is a sample code inspired by a similar code from the Decorator proposal.
export function log(target) {
if (typeof target.descriptor.value === 'function') {
const original = target.descriptor.value;
target.descriptor.value = function(...args) {
console.log('arguments: ', args);
const result = original.apply(this, args);
console.log('result: ', result);
return result;
}
}
return target;
}
Afterward, the Decorator will work as such:
import {log} from './decorators.js';
export default class DogSounds {
@log
barkSound() {
console.log('woof');
}
@log
say(to_say) {
console.log(to_say)
return 'The dog said ' + to_say
}
}
From the code above, you can tell that the application of the Decorator via the @
symbol is similar to annotation from a language like Java.
-
Can we use Decorators in JavaScript?
No. Because as of April 2022, it’s still a Stage 3 ECMA proposal.
-
When can we use Decorators in JavaScript?
You can use Decorators in JavaScript when the Decorators proposal reaches Stage 4 and is included in the next edition of ECMAScript.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn