Advanced Encryption Standard in JavaScript
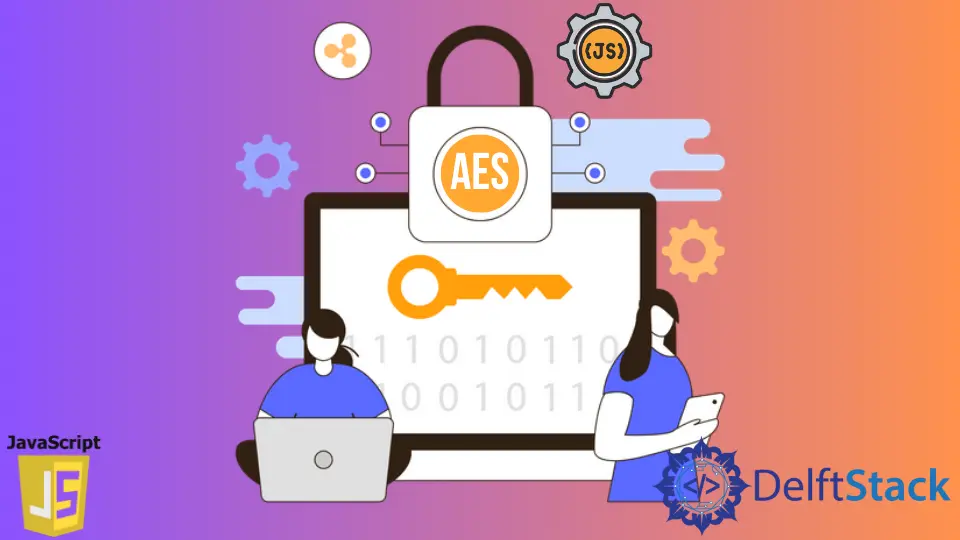
AES stands for Advanced Encryption Standard
; it encrypts sensitive data transfer as the name suggests. Like many organization needs security at a top-level priority.
Advanced Encryption Standard in JavaScript
AES is an algorithm developed for the encryption of data using some standards. It uses the same key to encrypt and decrypt data, called symmetric encryption.
These algorithms are used in different communication apps such as WhatsApp
, Signal
, etc. The flow is the message we write when sent is encrypted to pass through the internet so that no one can hack our message.
If they even hack it, they will not be able to decrypt the message, and when the message is reached to the destination receiver endpoint, it is decrypted using the same key as the sender have. Those keys are provided by the application to both the sender and receiver.
A practical example is given below using a third-party library, JSAES. That only takes two arguments as a parameter, the first one for the text to encrypt and the second for the password.
These two parameters are used both for encryption and decryption methods. Moreover, this example has nothing fancy; go for it and practice according to your needs.
<!DOCTYPE html>
<html>
<head>
<title>AES</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/crypto-js/3.1.2/rollups/aes.js"></script>
<script>
function encrypt() {
var encrypted = CryptoJS.AES.encrypt(
document.getElementById("text").value,
document.getElementById("password").value
);
document.getElementById("EncryptedValue").innerHTML = encrypted;
document.getElementById("decrypted").innerHTML = "";
}
function decrypt() {
var decrypted = CryptoJS.AES.decrypt(
document.getElementById("EncryptedValue").innerHTML,
document.getElementById("password").value
).toString(CryptoJS.enc.Utf8);
document.getElementById("decrypted").innerHTML = decrypted;
document.getElementById("EncryptedValue").innerHTML = "";
}
</script>
</head>
<body>
<h1>This is a Heading</h1>
<br />Data to encrypt:
<input id="text" type="text" placeholder="Enter text to encrypt" />
<br />password: <input id="password" type="text" value="cool" />
<br /><button onclick="encrypt()">encrypt</button>
<br />Encrypted Value:<br /><span id="EncryptedValue"></span>
<br />
<button onclick="decrypt()">decrypt</button>
<br />Decrypted Value: <span id="decrypted"></span>
</body>
</html>
Output:
Decrypt Data:
AES executes operations on bytes of data instead of bits. The cipher processes 128 bits (16 bytes) of the input data at a time since the block size is 128 bits.
The receiver and sender will have the same secret key (symmetric) to encrypt and decrypt.