Caesar Cipher in JavaScript
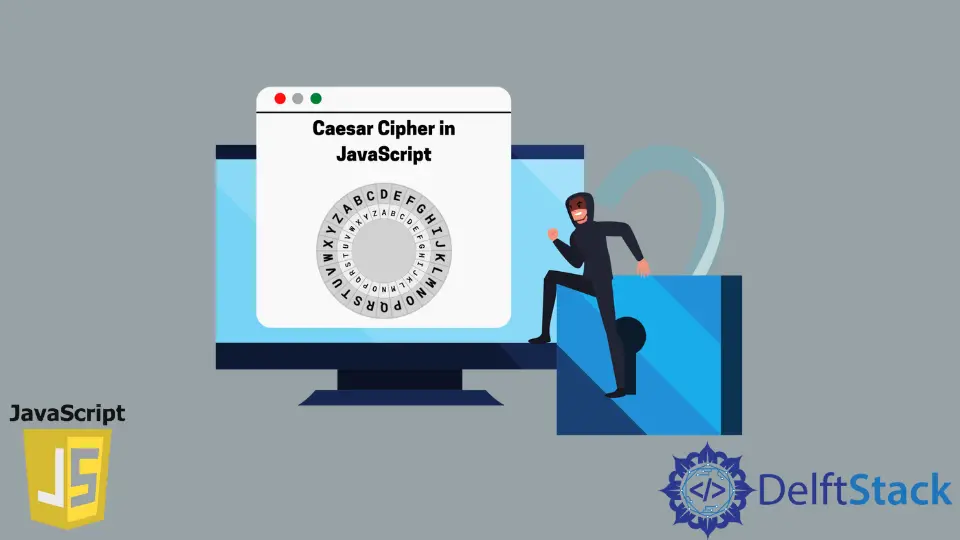
In this article, we will learn and use Caesar Cipher in JavaScript source code. The Caesar Cipher is a technique to encrypt and decrypt the string in programming.
Caesar Cipher
It is one of the easiest and most advanced techniques for encrypting data. In the provided string text, we replace and change each letter with the letter, which is a fixed number downwards or upwards in the alphabet.
For example, if we define upward fixed number 3, "A"
in a string will be replaced with "D"
, and "B"
will be replaced with "E"
, and so on.
Let’s suppose we defined upward fixed number 3 to shift a letter; our encryption will provide a result, as shown below.
original string = "hello world"
result string = "khoor zruog"
Algorithm of Caesar Cipher
A string that needs to be encrypted is called text; first, we need to define fix number between 0 to 25 as we know the total alphabets are 26; then, we need to traverse the text provided one character at a time.
For each index, convert each character as per the rule of downwards increment or upwards increment, which we have decided already. Finally, we need to generate the resultant string.
Caesar Cipher in JavaScript
In JavaScript, developers mostly used built-in or custom-created encryption techniques to secure data during the interaction with the server. In a JavaScript web application, we need to encrypt our data most of the time before uploading it to our database.
We will create an example of the JavaScript function below that will help us to encrypt our string in the Caesar Cipher technique.
Example:
const org = 'hello world';
const createMAp = (alphabets, shift) => {
return alphabets.reduce((charsMap, currentChar, charIndex) => {
const copy = {...charsMap};
let ind = (charIndex + shift) % alphabets.length;
if (ind < 0) {
ind += alphabets.length;
};
copy[currentChar] = alphabets[ind];
return copy;
}, {});
};
const encrypt = (org, shift = 0) => {
const alphabets = 'abcdefghijklmnopqrstuvwxyz'.split('');
const map = createMAp(alphabets, shift);
return org.toLowerCase().split('').map(char => map[char] || char).join('');
};
console.log('original string : ' + org)
console.log('result string :' + encrypt(org, 3))
Output:
"original string : hello world"
"result string :khoor zruog"
In the above JavaScript source, we have created the encrypt
function in which we are passing string values and fixed numbers as an argument. We have defined all alphabets and generated map objects using the createMap()
function.
In the createMap()
function, we used the reduce
method to generate the object. We have used the toLowerCase()
and split()
methods to avoid and generate new strings avoiding special characters with the fixed number 3.