The goto Statement in JavaScript
- Understanding the Goto Statement
- Using Labels and Break Statements
- Using Functions for Control Flow
- Conclusion
- FAQ
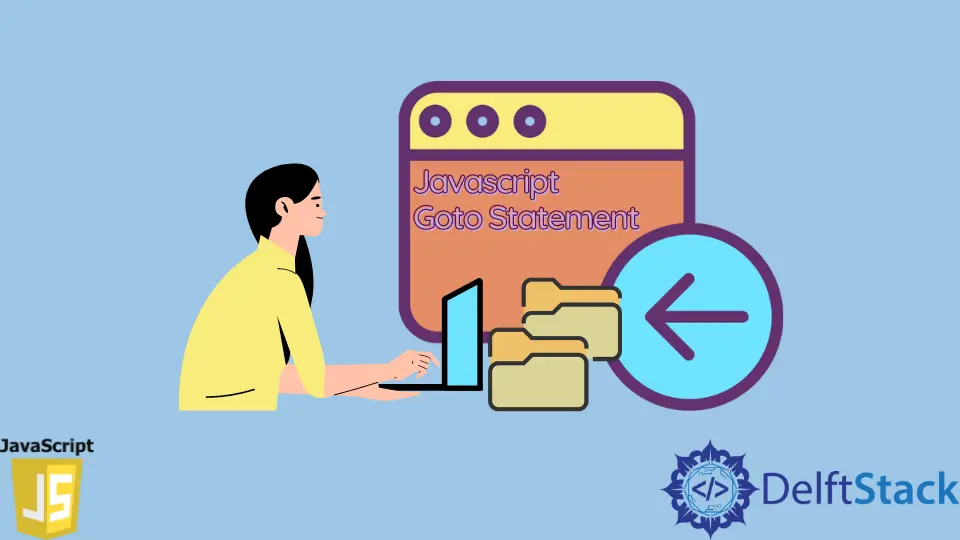
JavaScript is a versatile language, but it often raises questions about its control flow mechanisms. One such topic is the goto statement, which many programmers might find intriguing yet confusing. Although JavaScript does not support a traditional goto statement, understanding its alternatives can enhance your coding skills and improve your ability to manage program flow effectively.
In this tutorial, we will explore how to simulate a goto-like behavior in JavaScript using other control structures. By the end, you will have a clearer understanding of how to manage flow in your JavaScript applications without the need for an explicit goto statement.
Understanding the Goto Statement
The goto statement is a control flow statement that allows you to jump to a specific point in your program. While this may sound convenient, it can lead to code that is difficult to read and maintain. Many programming languages, including JavaScript, have moved away from using goto due to these concerns. Instead, developers use loops, functions, and conditionals to control the flow of their programs.
In JavaScript, we can achieve similar behavior by using constructs like loops, labels, and functions. This approach not only keeps your code cleaner but also makes it easier to understand. Let’s dive into some practical ways to implement goto-like functionality in JavaScript.
Using Labels and Break Statements
Labels in JavaScript can be used in conjunction with break statements to create a flow that resembles a goto statement. This method allows you to jump out of nested loops or switch statements efficiently. Here’s how it works:
outerLoop: for (let i = 0; i < 3; i++) {
for (let j = 0; j < 3; j++) {
if (i === 1 && j === 1) {
break outerLoop;
}
console.log(`i = ${i}, j = ${j}`);
}
}
Output:
i = 0, j = 0
i = 0, j = 1
i = 0, j = 2
i = 1, j = 0
In this example, we have an outer loop labeled outerLoop
and an inner loop. When the condition i === 1 && j === 1
is met, the break statement exits both loops. This effectively simulates a goto statement by jumping to a specific point in the code, allowing you to manage the flow without losing clarity.
This technique is useful when you need to exit multiple levels of loops based on certain conditions. However, it’s essential to use labels judiciously, as overusing them can lead to less readable code.
Using Functions for Control Flow
Another effective way to manage flow in JavaScript is by using functions. Functions allow you to encapsulate logic and control execution based on specific conditions. Here’s a simple example:
function checkCondition(i, j) {
if (i === 1 && j === 1) {
return true;
}
return false;
}
for (let i = 0; i < 3; i++) {
for (let j = 0; j < 3; j++) {
if (checkCondition(i, j)) {
console.log("Condition met, exiting loops.");
return;
}
console.log(`i = ${i}, j = ${j}`);
}
}
Output:
i = 0, j = 0
i = 0, j = 1
i = 0, j = 2
i = 1, j = 0
In this code, we define a function called checkCondition
that checks if the current values of i
and j
meet a specific condition. If they do, we exit the loops using the return statement. This method keeps your code organized and allows for easier debugging and testing.
Using functions for control flow not only simulates the goto behavior but also promotes reusability. By encapsulating logic within functions, you can keep your code modular and maintainable.
Conclusion
While JavaScript does not include a traditional goto statement, you can achieve similar flow control using labels, break statements, and functions. These alternatives help maintain clean and readable code while allowing you to manage complex control flows effectively. By understanding these techniques, you can improve your JavaScript skills and write more efficient programs.
In summary, mastering control flow in JavaScript is crucial for any developer. By leveraging the power of labels and functions, you can simulate the behavior of a goto statement without sacrificing code quality.
FAQ
-
What is the goto statement in programming?
The goto statement is a control flow statement that allows jumping to a specific point in the code. However, it is often discouraged due to potential readability issues. -
Does JavaScript support the goto statement?
No, JavaScript does not support a traditional goto statement, but you can use labels and break statements to achieve similar functionality. -
Why is using goto considered bad practice?
Using goto can lead to “spaghetti code,” making it difficult to read and maintain. Structured programming encourages using loops and functions instead. -
How can I exit multiple loops in JavaScript?
You can use labeled statements with break or return to exit multiple loops efficiently. -
Are there alternatives to using goto in JavaScript?
Yes, you can use functions, loops, and conditionals to control the flow of your program without needing a goto statement.