How to Get MAC Address in JavaScript
- The MAC Address
- Format of MAC Address
-
Use the
ActiveX
Object to Get MAC Address in JavaScript - Retrieve MAC Addresses via Node.js
- Obtain MAC Address via Network Information API (Browser Support Limited)
- Use External Services or APIs
- Conclusion
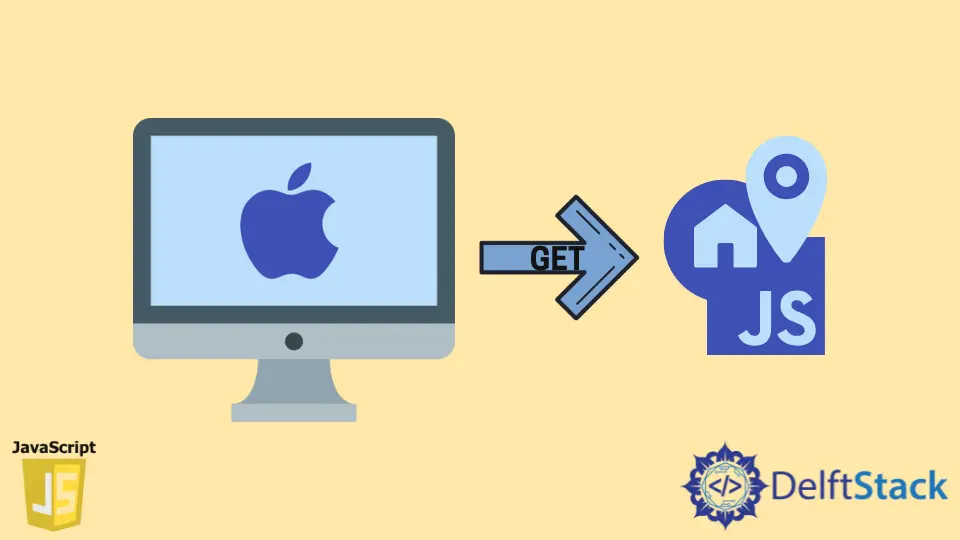
Retrieving a device’s MAC address via JavaScript isn’t directly accessible due to security and privacy concerns. However, this post can explain alternative approaches to achieve similar functionalities using JavaScript.
A MAC (Media Access Control) address is a unique identifier assigned to computers and network interfaces for communication on the physical network segment. JavaScript does not have direct access to MAC addresses due to browser security restrictions.
The MAC Address
MAC (Media Access Control) is a 48-bit unique identifier assigned to the Network Interface Controller. It is a physical address associated with every networking device.
The Data Link Layer uses a MAC address to route a data packet from a source point to its destination. It is separated into two sublayers in the IEEE 802 standard:
- Logical Link Control (LLC) Sublayer
- Media Access Control (MAC) Sublayer
Because millions of network devices exist and we need to identify each uniquely, the MAC address is unique worldwide.
Format of MAC Address
The MAC Address is a hexadecimal number with 12 digits (6-byte binary number) commonly written in Colon-Hexadecimal notation. The OUI (Organizational Unique Identifier) is the first 6-digits of the MAC Address (for example, 00:40:96).
The IEEE Registration Authority Committee assigns these MAC prefixes to its registered suppliers. Here are some OUI of well-known manufacturers:
CC:46:D6 - Cisco
3C:5A:B4 - Google, Inc.
3C:D9:2B - Hewlett Packard
00:9A:CD - HUAWEI TECHNOLOGIES CO.,LTD
The manufacturer allocates the rightmost six digits to the Network Interface Controller.
As mentioned above, the MAC address is written in a Colon-Hexadecimal format, but this is merely a conversion and not a necessity. Any of the following formats can be used to represent a MAC address:
Use the ActiveX
Object to Get MAC Address in JavaScript
We can only get the MAC address in JavaScript using the ActiveX
object control for Microsoft. It will only work on Internet Explorer as the ActiveX
object is unavailable with any other browser.
To enable the ActiveX
object in Internet Explorer, we will go to Tools
and select Internet Options
. Then, on the Security
tab, we will click on Custom Level
.
We will go down until we see Initialize
and the script ActiveX controls not marked as safe
. We will enable it, then click Ok
.
<script type="text/javascript">
var macAddress = "";
var computerName = "";
var wmi = GetObject("winmgmts:{impersonationLevel=impersonate}");
e = new Enumerator(wmi.ExecQuery("SELECT * FROM Win32_NetworkAdapterConfiguration WHERE IPEnabled = True"));
for(; !e.atEnd(); e.moveNext()) {
var s = e.item();
macAddress = s.MACAddress;
computerName = s.DNSHostName;
}
</script>
Now, we go to the coding part. We can add the source code above anywhere on our HTML head or body, but if we choose to put it in the body, place it above the code below.
Here, we will access Win32_NetworkAdapterConfiguration
to read network-related details like the MAC Address, IP Address, and the computer name. Then, we can use text boxes to display that information or whatever we like.
<script type="text/javascript">
<input type="text" id="txtMACAdress" />
<input type="text" id="txtComputerName" />
<script type="text/javascript">
document.getElementById("txtMACAdress").value = unescape(macAddress);
document.getElementById("txtComputerName").value = unescape(computerName);
</script>
We must make sure that we place the declarations above this code to display the values correctly. Now, the client can see their own MAC address displayed on the screen.
You can find the complete source code for this problem in this link. We have also provided a screenshot below.
The output on this screenshot has been blurred out for obvious reasons.
Retrieve MAC Addresses via Node.js
In networking, a MAC (Media Access Control) address serves as a unique identifier assigned to network interfaces. While retrieving this address programmatically using Node.js is possible, it’s important to note that this information is sensitive and generally not exposed directly through JavaScript in web browsers due to security and privacy concerns.
In Node.js, the os
module provides a powerful method, os.networkInterfaces()
, enabling developers to access detailed network interface information, including MAC addresses.
Using os.networkInterfaces()
Method
Node.js’ os.networkInterfaces()
function allows retrieving detailed information about network interfaces on the system. The following code demonstrates how to use this function to obtain MAC addresses:
const os = require('os');
const networkInterfaces = os.networkInterfaces();
const macAddresses = {};
Object.keys(networkInterfaces).forEach((key) => {
const interfaces = networkInterfaces[key];
macAddresses[key] = interfaces
.filter((details) => details.mac && details.mac !== '00:00:00:00:00:00')
.map((detail) => detail.mac);
});
console.log('MAC Addresses:', macAddresses);
This code snippet retrieves and organizes MAC addresses by interface type (e.g., 'Ethernet'
, 'Wi-Fi'
, 'Loopback'
) into an object (macAddresses
).
Overall, this code snippet retrieves and organizes MAC addresses based on their associated network interface types using Node.js’ os.networkInterfaces()
function and JavaScript array manipulation functions like filter()
and map()
.
Obtain MAC Address via Network Information API (Browser Support Limited)
The Network Information API, although providing insights into network-related details, intentionally withholds direct access to MAC addresses.
The Network Information API, primarily designed to provide network-related data to web app, offers limited details about the user or machine’s network connection. However, it doesn’t expose MAC addresses to JavaScript due to browser security restrictions.
The following code demonstrates attempting to retrieve the MAC address using the Network Information API:
let macAddress;
if (navigator.userAgent.toLowerCase().indexOf('firefox') > -1) {
macAddress = 'Not accessible in Firefox';
} else {
macAddress = 'Not accessible in this browser';
}
console.log('MAC Address:', macAddress);
The navigator.userAgent
retrieves information about the user’s browser. The indexOf('firefox')
checks if the user agent string contains 'firefox'
, indicating the browser being used.
The conditional statement provides a general approach to handle browser-specific scenarios. Finally, display a message indicating that the MAC address is inaccessible due to browser limitations.
Use External Services or APIs
This approach involves making requests to external services or APIs that offer MAC address lookups based on provided IP addresses. It’s essential to note that this method might not always yield accurate answers or reliable results and might be subject to restrictions or limitations imposed by the external service.
Source code:
const ipAddress = '192.168.1.1'; // Replace with the target IP address
const apiUrl = 'https://example.com/api/getmac'; // Replace with actual API endpoint
fetch(`${apiUrl}?ip=${ipAddress}`)
.then(response => response.json())
.then(data => {
const macAddress = data.macAddress;
console.log('MAC Address:', macAddress);
})
.catch(error => {
console.error('Error:', error);
});
First, define the IP address and API endpoint by replacing the ipAddress
variable with the target IP address and apiUrl
with the actual API endpoint offering MAC address lookup services.
The fetch
function sends a GET
request to the specified API endpoint, providing the IP address as a parameter. Once the response is received, it’s parsed as JSON (response.json()
), and the retrieved MAC address is extracted from the data and logged into the console.
Conclusion
In this post, we have learned that retrieving a device’s MAC address directly through JavaScript within browsers is restricted due to security measures. This unique identifier for network interfaces isn’t directly accessible due to privacy concerns.
Techniques like ActiveX (limited to Internet Explorer) and the Network Information API in browsers don’t grant access to MAC addresses. Alternatives exist: Node.js provides limited access in a server-side environment, and external services or APIs offer indirect methods using IP addresses.
However, these indirect approaches may lack reliability and security vulnerability and raise privacy considerations. Ultimately, respecting user privacy and adhering to security restrictions remain crucial when handling sensitive network information in web app.
Another post that answers how to get a client IP address in JavaScript is in this link.