How to Get Client IP Address in JavaScript
-
Use
Ipify
Application to Get Client IP Address in JavaScript -
Use
Ipinfo
Application to Get Client IP Address in JavaScript -
Use
Abstract
Application to Get Client IP Address in JavaScript
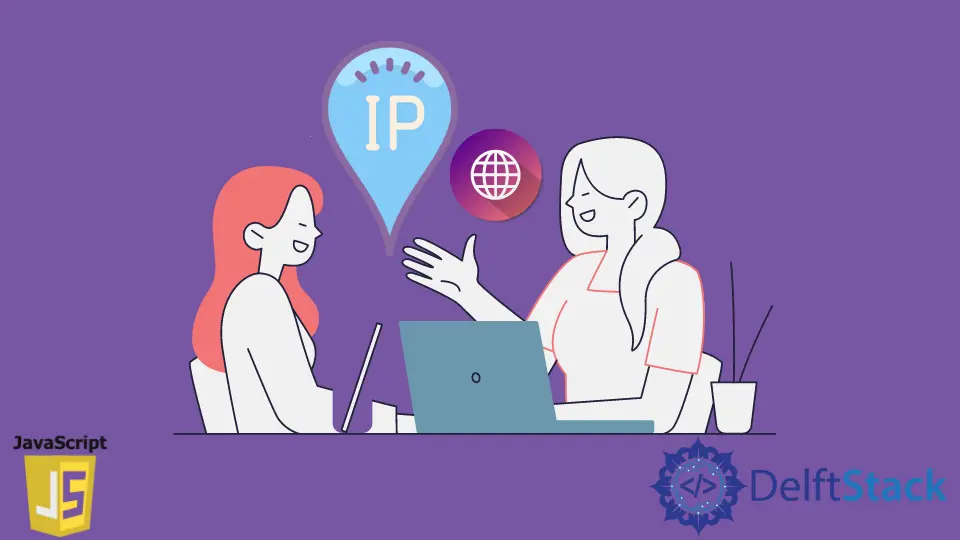
The IP address is a combination of numbers that gives a unique address for your network hardware. it is like a fingerprint, or just like a person has an email address to receive emails. It connects your computer to the other devices in the network, the web, and all over the world.
JavaScript gets a public IP address that belongs to a client using third-party applications. The third-party application fetches the user IP address and returns it in three formats - plain text, JSON, and JSONP format. There are dozens of such applications available on the internet.
In this article, we will introduce the three most popular tools to fetch IP address in JavaScript - ipify
, ipinfo
, and Abstract
.
Use Ipify
Application to Get Client IP Address in JavaScript
ipify
is an open-source application. You can get the codes in the GitHub repository. There is no limitation, even if you’re making millions of requests per minute.
Example:
Please install Axios
(HTTP client for the browser and node.js) or include CDN for it.
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<script>
async function getIpClient() {
try {
const response = await axios.get('https://api.ipify.org?format=json');
console.log(response);
} catch (error) {
console.error(error);
}
}
getIpClient();
</script>
Output:
data: {ip: "198.16.76.28"}
Use Ipinfo
Application to Get Client IP Address in JavaScript
Ipinfo
application has a usage limitation. You can make 1,000 requests per day for free or register with a free plan to get 50,000 free requests per month.
Example:
async function getIpClient() {
try {
const response = await axios.get('https://ipinfo.io/json');
console.log(response);
} catch (error) {
console.error(error);
}
}
getIpClient();
Output:
{
city: "Amsterdam"
country: "NL"
ip: "198.16.76.28"
loc: "52.3740,4.8897"
org: "AS174 Cogent Communications"
postal: "1012"
readme: "https://ipinfo.io/missingauth"
region: "North Holland"
timezone: "Europe/Amsterdam"
}
Use Abstract
Application to Get Client IP Address in JavaScript
Abstract
application also has a limitation of 20,000 free requests per month. And it requires registration to get your API key even with a free plan.
Example:
async function getIpClient() {
try {
const response = await axios.get(
'https://ipgeolocation.abstractapi.com/v1/?api_key=<your_api_key>');
console.log(response);
} catch (error) {
console.error(error);
}
}
getIpClient();
Please visit Abstract
website to preview the output of the above function.