How to Convert Seconds to Minutes in JavaScript
- Basic Conversion of Seconds to Minutes in JavaScript
-
Use
toString()
andpadStart()
Methods to Format inMM:SS
in JavaScript
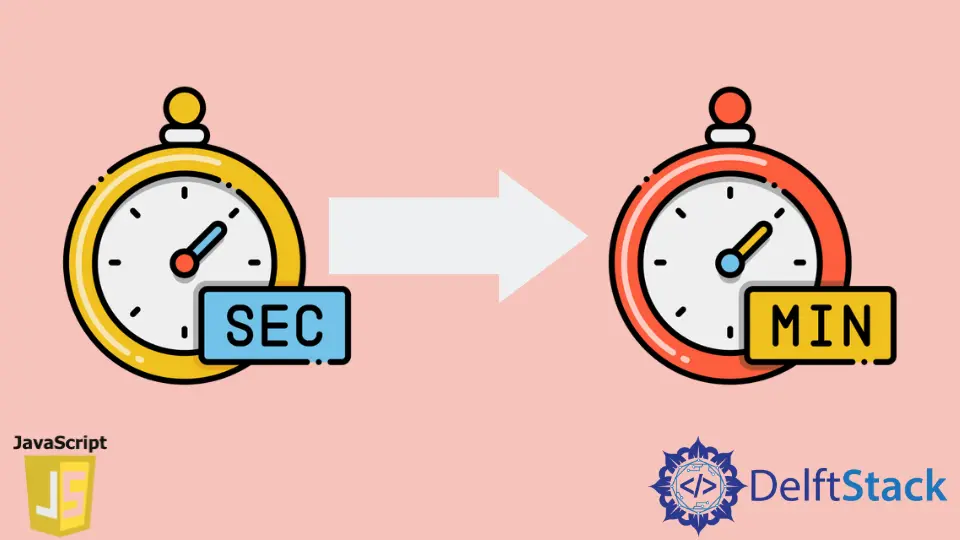
We will consider a fundamental way of applying mathematical expression for converting seconds into minutes.
In our drive, we will initially divide the given value (in seconds) by 60
as 1 minute = 60 seconds
. With this basic concept, we will be retrieving the corresponding minutes for the stated seconds.
Here, specifically, we will see an example with elementary math. The other one will have the output in a beautified format where we will be using the toString()
method and padStart()
method.
Let’s hop on to the code fences to preview the solution better.
Basic Conversion of Seconds to Minutes in JavaScript
We will set a suitable number (considering the value in seconds). We will divide the number by 60
and take the floor
value as the rounded minute value.
For calculating the rest seconds that couldn’t fit into a full minute, we will perform the mod
of the whole second
number (var = time_s
) by 60
. So, by dividing time_s
by 60
, we will get the full minutes, and the remainder will be calculated by doing time_s mod(%) 60
.
Code Snippet:
var time_s = 462;
var minute = Math.floor(time_s / 60);
var rest_seconds = time_s % 60;
console.log(minute + ' minutes ' + rest_seconds + ' seconds.')
Output:
We had 462 seconds and divided it by 60
. After executing this expression, we received the value 7.7
and picked the floor
value 7
.
The remainder here is 42
, and this is the remaining seconds that couldn’t reach a full minute. So, finally, the output is 7 minutes 42 seconds
.
Use toString()
and padStart()
Methods to Format in MM:SS
in JavaScript
The padStart()
method works on strings, and so we will convert our number elements to a string via the toString()
method. We have similar mathematical expressions to convert the seconds into minutes.
But to be more specific, if we convert a single-valued minute or second, the padStart()
method will make it a 2-digit string with the starting digit as 0
. Let’s visualize the code lines and the output.
Code Snippet:
var time_s = 462;
var minute = Math.floor(time_s / 60);
var rest_seconds = time_s % 60;
console.log(
minute.toString().padStart(2, '0') + ':' +
rest_seconds.toString().padStart(2, '0'));
Output: