How to Change CSS Property Using JavaScript
-
Change CSS Property With
getElementsByClassName
in JavaScript -
Change CSS Property With
getElementById
in JavaScript -
Change CSS Property With
querySelector()
in JavaScript - Conclusion
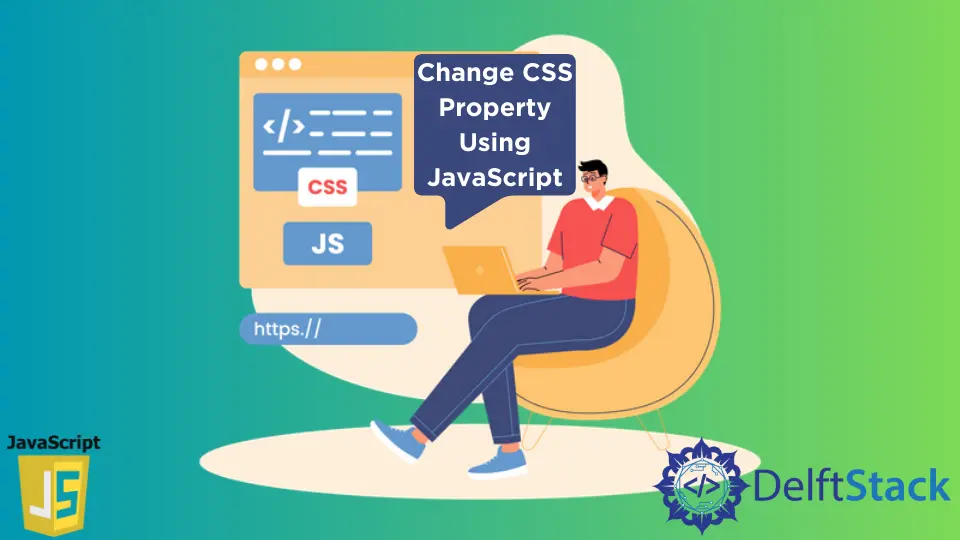
HyperText Markup Language (HTML) is static and usually dumb. It means it does not have the capabilities to execute pieces of code based on dynamic conditions. It does not have provisions for if
condition blocks to show-hide certain HTML elements or tags if a condition is satisfied. For such scenarios, we may need to rely on JavaScript or jQuery for changing the CSS styles of an HTML element.
To change an HTML element’s style, say a <div>
, we should select the <div>
or that particular HTML element uniquely. Now, we can do this with the following functions of the Document Interface.
Change CSS Property With getElementsByClassName
in JavaScript
getElementsByClassName
searches the entire HTML document and returns an array of all HTML elements having the class name as passed in the parameters of this function. We can also use it on an element to find the sub-elements with the specified CSS class name. The syntax for getElementByClassName
is as follows.
document.getElementsByClassName('green-class'));
Set Style Using element.style
Once we have identified the element uniquely, we can use the .style
or .className
methods to change its CSS styles. Refer to the following examples.
<div class="col-md-12">
<div class="p-3">
<label>Input String:</label><br>
<input type="text" class="input-blue-border" id="b1" value="120">
<button class="ml-3" onclick="changeStyle()">Change Border</button>
</div>
</div>
function changeStyle() {
document.getElementsByClassName('input-blue-border')[0].style.borderColor =
'red';
}
Note that in the changeStyle()
method we query the input element with the document.getElementsByClassName("input-blue-border")
method. It returns an array with selected elements. We select the first element of the array and change its bordercolor with .style.borderColor = "red"
.
Set Style Using element.className
One can use element.className
to change various style parameters of an HTML element by clubbing those as a class and assigning the class name to the selected element with element.className
. This method is helpful, especially in scenarios where we need to display an error in an input field. In that case, we need to change the border color of the input field to red color and the inner text of the input to red color. Hence we can club these styles as a class and assign them to the element using the element.className
attribute. The following code illustrates error handling.
<div class="col-md-12">
<div class="p-3">
<label>Input String:</label><br>
<input type="text" class="input-blue-border" id="b1" value="120">
<button class="ml-3" onclick="changeClass()">Change Border</button>
</div>
</div>
function changeClass() {
document.getElementsByClassName('input-blue-border')[0].className =
'input-error';
}
The input-error
class has a couple of attributes that set the border colour and the font colour of the input field to red.
Remarks
getElementByClassName()
method returns an array of elements that qualify the class name value passed as arguments.getElementByClassName()
can be used while applying style changes to more than one element by iterating over the array returned by this method.- Once the element is selected,
element.style.<style attribute>
sets the particular style attribute to the selected element. - Similarly,
element.className
helps set more than one style changes to the selected HTML element by clubbing those style attributes as a CSS class.
Change CSS Property With getElementById
in JavaScript
If we have a unique id assigned to an HTML element, we can query it and change its style with the getElementById()
function of the Document
interface. It is the most widely used method by web developers. Mostly the ids assigned to a div will be kept unique so that while executing the getElementById()
function, other HTML elements do not get selected. The syntax for getElementById()
is as depicted below.
document.getElementById("parent-1"));
As we select a unique element, in this case, the style changes are easy to do. The following codes depict the ways. It is similar to those mentioned previously, with a difference being how we query for an element. Here, we uniquely identify HTML nodes with the element id.
document.getElementById('b1').style.borderColor = 'red';
document.getElementById('b1').className = 'input-error';
Remarks
- Unlike the
getElementByClassName()
, thegetElementById()
method returns only one object which is the node element selected by the query. - The intended HTML needs to have an id attribute for
getElementById()
method to work. - If more than one HTML node has the same id, then the
getElementById()
method will return the first element having the specified id. - Unlike
getElementByClassName()
, the style changes can be applied directly on the object returned by thegetElementById()
function as it returns an object instead of an array.
Change CSS Property With querySelector()
in JavaScript
querySelector()
method is a superset of features offered by the element selection mechanisms. It has the combined power of both getElementsByClassName()
and getElementById()
methods. With this method, we can select the HTML element the same way while writing CSS classes. It can work for selecting an element by id, by class and even by the HTML tag. It behaves similar to the getElementById()
method in terms of the return type. querySelector()
returns only the first HTML node element that satisfies the criteria mentioned in the parameters. The syntax used for querySelector()
is as follows:
document.querySelector('#<id of div>');
document.querySelector('.<css class name>');
document.querySelector('<HTML tag eg: div>');
Hence, here too the style can be applied to the selected HTML element by adding .style.borderColor
or .className
. If we use the same HTML structure and change the onclick
method to changeStyle()
, we can achieve bringing the new style dynamically by adding the code as described below in the JavaScript:
<button class="ml-3" onclick="changeStyle()">Change Border</button>
function changeStyle() {
document.querySelector('.input-blue-border').style.borderColor = 'red';
}
Similarly, if we are to change the CSS class of the input, instead of just a style change, we need to use the .className()
attribute instead of .style.borderColor
in the above example.
<button class="ml-3" onclick="changeClass()">Change Border</button>
function changeClass() {
document.querySelector('.input-blue-border').className = 'input-error';
}
Remarks
- It is worthy of having an eye on the return type of
document.querySelector()
function. Instead of returning all the HTML objects satisfying the query, it returns the first element that fits the query condition. - The beauty of using the
querySelector()
is that we can use it in various circumstances, may it be querying based on the id of the HTML element or be its CSS class or even with the HTML tag name. - Dynamically change styles of more than one divs satisfying the query criteria may not be possible with
querySelector()
as it returns an object instead of an array of HTML elements passing the query terms.
Conclusion
For changing the style of an HTML element at run time, we can make use of .style
or .className
attributes of the selected HTML element. The challenges are faced while selecting the target HTML node. There are various ways to select HTML nodes uniquely. We can use the getElementsByClassName()
method, which uses the name of a CSS class to query the HTML node. Or uniquely select an element by assigning an id to it and querying it with .getElementById()
or using the multipurpose querySelector()
method that can fit all circumstances understanding the query based on the parameters passed to it.