How to Call Python From JavaScript
- Method 1: Using HTTP Requests with Flask
- Method 2: Using Child Processes in Node.js
- Method 3: Using WebSocket for Real-Time Communication
- Conclusion
- FAQ
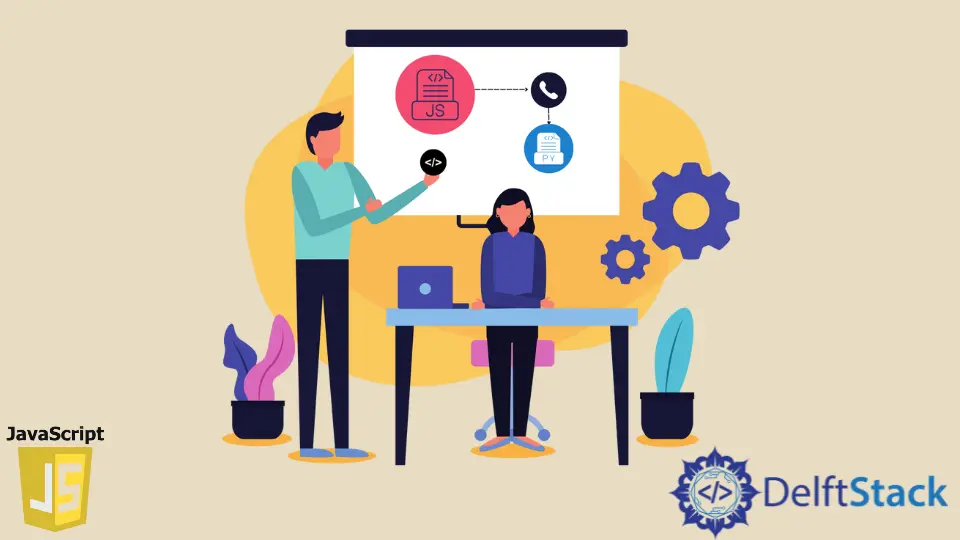
In today’s post, we’ll explore the intriguing intersection of Python and JavaScript. As developers, we often find ourselves needing to leverage the strengths of both languages. Whether you’re building a web application or automating tasks, knowing how to call Python from JavaScript can significantly enhance your projects. In this guide, we will delve into effective methods for invoking Python scripts from JavaScript, ensuring you have the tools you need to integrate these two powerful languages seamlessly. Let’s get started and unlock the potential of your applications!
Method 1: Using HTTP Requests with Flask
One of the most popular ways to call Python from JavaScript is by setting up a simple web server using Flask. Flask is a lightweight web framework for Python that allows you to create a RESTful API. This method involves creating an endpoint in Python that JavaScript can call using HTTP requests.
Setting Up Flask
First, you need to install Flask if you haven’t already. You can do this using pip:
pip install Flask
Next, create a simple Flask app that listens for incoming requests. Here’s an example:
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route('/api/data', methods=['GET'])
def get_data():
return jsonify({"message": "Hello from Python!"})
if __name__ == '__main__':
app.run(debug=True)
When you run this script, Flask will start a local server.
Output:
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
Calling the Flask API from JavaScript
Now that your Flask server is running, you can call this API from your JavaScript code using the Fetch API. Here’s how:
fetch('http://127.0.0.1:5000/api/data')
.then(response => response.json())
.then(data => console.log(data.message))
.catch(error => console.error('Error:', error));
Output:
Hello from Python!
By setting up a Flask server, you create a bridge between your JavaScript front end and Python back end. This method allows you to send data back and forth easily, making it a robust solution for many web applications. Whether you need to process data or perform complex calculations, Flask can handle it efficiently.
Method 2: Using Child Processes in Node.js
Another effective way to call Python from JavaScript is by using Node.js’s built-in ability to spawn child processes. This method allows you to execute Python scripts directly from your JavaScript code. It’s particularly useful when you need to run standalone Python scripts that perform specific tasks.
Running Python Scripts
First, ensure you have Node.js installed on your machine. You can then create a simple Python script. Here’s a basic example:
# script.py
import sys
def main():
print("Hello from Python!")
sys.exit(0)
if __name__ == '__main__':
main()
With this script saved as script.py
, you can call it from your Node.js application. Here’s how to do that:
const { spawn } = require('child_process');
const pythonProcess = spawn('python', ['script.py']);
pythonProcess.stdout.on('data', (data) => {
console.log(data.toString());
});
pythonProcess.stderr.on('data', (data) => {
console.error(`Error: ${data}`);
});
Output:
Hello from Python!
Explanation
In this method, you use Node.js’s child_process
module to spawn a new Python process. The spawn
function takes the command to execute and the arguments as an array. You can listen for data emitted from the Python script using the stdout
event. This method works well for executing scripts that don’t require user interaction and can return results directly to your JavaScript code.
Method 3: Using WebSocket for Real-Time Communication
For applications that require real-time communication, using WebSocket can be an excellent approach. This allows you to establish a persistent connection between your JavaScript front end and Python back end, enabling bidirectional communication.
Setting Up WebSocket with Flask
You can use Flask-SocketIO to create a WebSocket server in Python. First, install Flask-SocketIO:
pip install flask-socketio
Then, create a simple WebSocket server:
from flask import Flask
from flask_socketio import SocketIO
app = Flask(__name__)
socketio = SocketIO(app)
@socketio.on('message')
def handle_message(msg):
print('Received message: ' + msg)
socketio.send('Hello from Python!')
if __name__ == '__main__':
socketio.run(app)
Output:
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
Connecting from JavaScript
Now, you can connect to this WebSocket server from your JavaScript code:
const socket = io('http://127.0.0.1:5000');
socket.on('connect', () => {
socket.send('Hello from JavaScript!');
});
socket.on('message', (data) => {
console.log(data);
});
Output:
Hello from Python!
Explanation
In this setup, you create a WebSocket connection between your JavaScript app and the Flask server. When JavaScript sends a message, the Python server receives it and can respond in real-time. This method is particularly beneficial for applications like chat apps or live data feeds, where you need instant updates without constantly polling the server.
Conclusion
Integrating Python with JavaScript can open up a world of possibilities for your projects. Whether you choose to use Flask for RESTful APIs, Node.js for executing scripts, or WebSocket for real-time communication, each method has its own strengths. By mastering these techniques, you can create more dynamic and powerful applications that leverage the best features of both languages. Start experimenting with these methods today, and see how they can enhance your development workflow!
FAQ
-
Can I call a Python script from a browser?
You cannot directly call a Python script from a browser. Instead, you set up a server (like Flask) that runs the script and communicates with your JavaScript code via HTTP requests. -
Is Flask the only option for calling Python from JavaScript?
No, while Flask is popular, you can use other frameworks like Django or FastAPI to achieve similar results. -
What are the limitations of using child processes in Node.js?
Child processes are suitable for running scripts but may not be the best choice for long-running applications, as they can consume more resources. -
How do I handle errors when calling Python from JavaScript?
You can handle errors using try-catch blocks in Python and by listening for error events in JavaScript. -
Can I use WebSocket with other programming languages?
Yes, WebSocket is a protocol that can be implemented in various programming languages, allowing real-time communication between clients and servers.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn