How to Addition Assignment or += Operator in JavaScript
-
What Is
+=
in JavaScript -
Use the
-=
as a Subtraction Assignment Operator -
Use the
*=
as a Multiplication Assignment Operator -
Use the
/=
as aDivision Assignment
Operator -
Use the
%=
as a Remainder/Modulus Assignment Operator
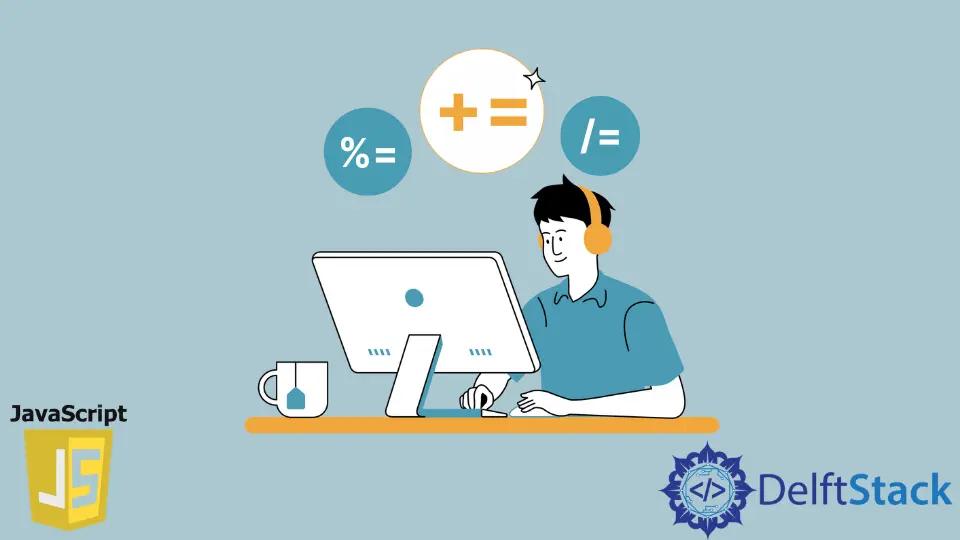
This article will inform you about JavaScript’s addition assignment operator. Let’s look at how it works and read the other assignment operators.
What Is +=
in JavaScript
The +=
is the addition assignment operator in JavaScript. It adds two values together and assigns the result to a variable in a single JavaScript statement.
Syntax:
x += y;
means x = x + y;
a += b;
means a = a + b;
The behavior of the addition assignment operator, either addition or concatenation, depends on the types of two operands, as explained in the following example.
<html>
<head>
<title>
JavaScript addition assignment operator
</title>
</head>
<body>
<h3> <u> JavaScript addition assignment operator </u></h3>
<body>
<script>
var a = 3;
var b = "Delft"
document.write(a += 5); // Addition
document.write("<br>");
document.write("<br>");
document.write(b += "Stack"); // Concatenation
</script>
</html>
Output:
JavaScript addition assignment operator
8
DelftStack
Example of JavaScript addition assignment operator:
<html>
<head>
<title>
JavaScript addition assignment operator
</title>
</head>
<body>
<h3> <u> JavaScript addition assignment operator in different scenarios </u></h3>
<body>
<script>
var ds = 'DelftStack';
var n = 4;
var b = true;
// adding number with number results in addition
document.write(n += 5); // 9
document.write("<br>");
// adding Boolean with Number results in addition
document.write(b += 1); // 2
document.write("<br>");
// adding Boolean with Boolean results in addition
document.write(b += false); // 2
document.write("<br>");
// adding Number with String results in concatenation
document.write(n += 'Cybexo') // "9Cybexo"
document.write("<br>");
// adding String with Boolean results in concatenation
document.write(ds += false) // "DelftStackfalse"
document.write("<br>");
// adding String with String results in concatenation
document.write(ds += ' Technology') // "DelftStack Technology"
</script>
</html>
Output:
JavaScript addition assignment operator in different scenarios
9
2
2
9Cybexo
DelftStackfalse
DelftStackfalse Technology
Similarly, in JavaScript, we also have:
- Subtraction assignment operator
-=
- Multiplication assignment operator
*=
- Division assignment operator
/=
- Modulus assignment operator
%=
Use the -=
as a Subtraction Assignment Operator
The subtraction assignment operator is used to subtract the right operand number value from a variable value and then assign the result to the variable.
Syntax:
x -= y;
means x = x - y;
a -= b;
means a = a - b;
Use the *=
as a Multiplication Assignment Operator
The multiplication assignment operator multiplies a variable by the value of the right operand and then assigns the result to the variable in a single statement.
Syntax:
x *= y;
means x = x * y;
a *= b;
means a = a * b;
Use the /=
as a Division Assignment
Operator
It divides the variable by the right operand number and assigns the result to the variable in a single JavaScript statement.
Syntax:
x /= y;
means x = x / y;
a /= b;
means a = a / b;
Use the %=
as a Remainder/Modulus Assignment Operator
The remainder/modulus assignment operator divides a variable by the value of the right operand and then assigns the remainder to the variable in a single JavaScript statement.
Syntax:
x %= y means x = x % y
a %= b means a = a % b