JavaScript 中的加法賦值或 += 運算子
Muhammad Muzammil Hussain
2023年10月12日
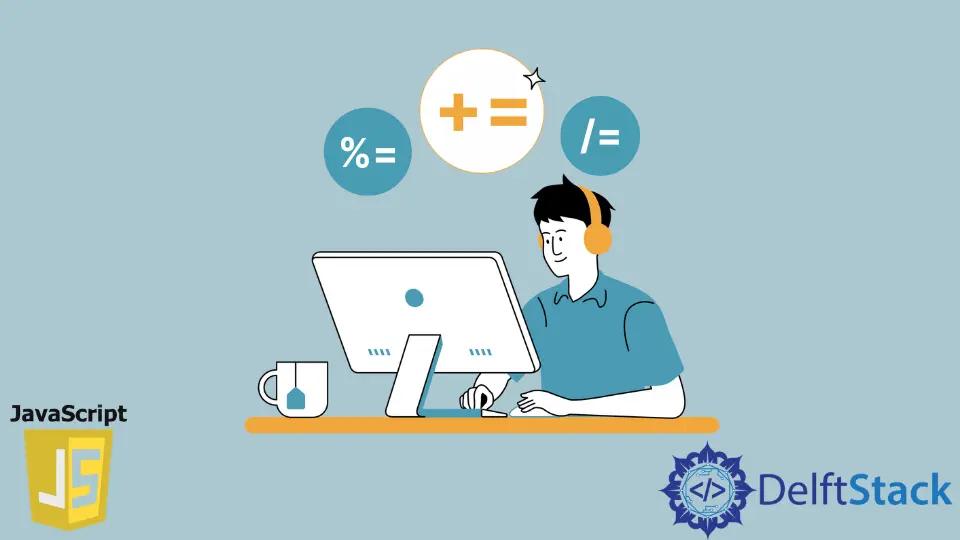
本文將向你介紹 JavaScript 的加法賦值運算子。讓我們看看它是如何工作的並閱讀其他賦值運算子。
JavaScript 中的 +=
是什麼
+=
是 JavaScript 中的加法賦值運算子。它將兩個值相加,並將結果分配給單個 JavaScript 語句中的變數。
語法:
x += y;
means x = x + y;
a += b;
means a = a + b;
加法賦值運算子(加法或連線)的行為取決於兩個運算元的型別,如以下示例中所述。
<html>
<head>
<title>
JavaScript addition assignment operator
</title>
</head>
<body>
<h3> <u> JavaScript addition assignment operator </u></h3>
<body>
<script>
var a = 3;
var b = "Delft"
document.write(a += 5); // Addition
document.write("<br>");
document.write("<br>");
document.write(b += "Stack"); // Concatenation
</script>
</html>
輸出:
JavaScript addition assignment operator
8
DelftStack
JavaScript 加法賦值運算子示例:
<html>
<head>
<title>
JavaScript addition assignment operator
</title>
</head>
<body>
<h3> <u> JavaScript addition assignment operator in different scenarios </u></h3>
<body>
<script>
var ds = 'DelftStack';
var n = 4;
var b = true;
// adding number with number results in addition
document.write(n += 5); // 9
document.write("<br>");
// adding Boolean with Number results in addition
document.write(b += 1); // 2
document.write("<br>");
// adding Boolean with Boolean results in addition
document.write(b += false); // 2
document.write("<br>");
// adding Number with String results in concatenation
document.write(n += 'Cybexo') // "9Cybexo"
document.write("<br>");
// adding String with Boolean results in concatenation
document.write(ds += false) // "DelftStackfalse"
document.write("<br>");
// adding String with String results in concatenation
document.write(ds += ' Technology') // "DelftStack Technology"
</script>
</html>
輸出:
JavaScript addition assignment operator in different scenarios
9
2
2
9Cybexo
DelftStackfalse
DelftStackfalse Technology
同樣,在 JavaScript 中,我們也有:
- 減法賦值運算子
-=
- 乘法賦值運算子
*=
- 除法賦值運算子
/=
- 模賦值運算子
%=
使用 -=
作為減法賦值運算子
減法賦值運算子用於從變數值中減去右運算元編號值,然後將結果賦給變數。
語法:
x -= y;
means x = x - y;
a -= b;
means a = a - b;
使用 *=
作為乘法賦值運算子
乘法賦值運算子將變數乘以右運算元的值,然後在單個語句中將結果賦給變數。
語法:
x *= y;
means x = x * y;
a *= b;
means a = a * b;
使用/=
作為除法分配
運算子
它將變數除以正確的運算元編號,並在單個 JavaScript 語句中將結果分配給變數。
語法:
x /= y;
means x = x / y;
a /= b;
means a = a / b;
使用 %=
作為餘數/模賦值運算子
餘數/模賦值運算子將變數除以右運算元的值,然後在單個 JavaScript 語句中將餘數分配給變數。
語法:
x %= y means x = x % y
a %= b means a = a % b