Working of StdOut in Java
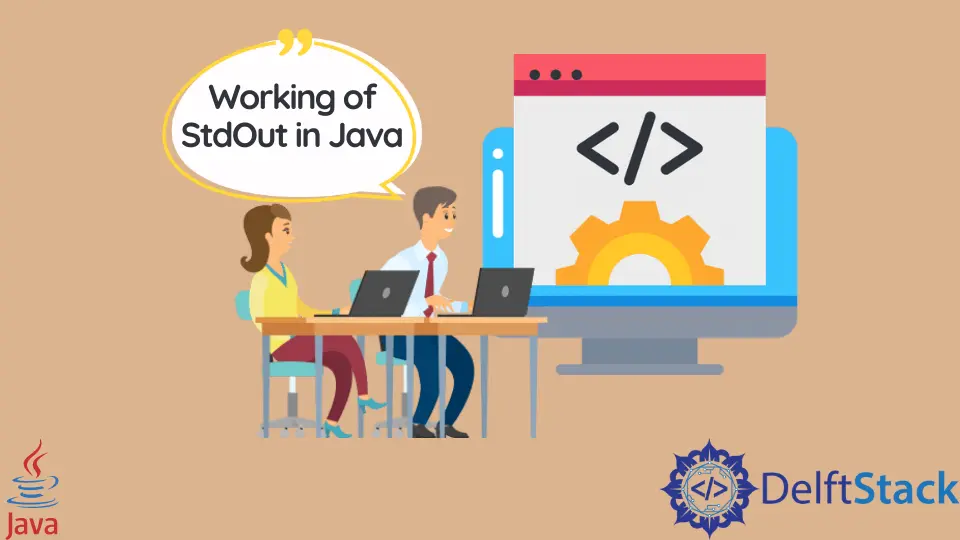
This tutorial describes the StdOut
class and demonstrates the working of StdOut
in Java.
StdOut
in Java
The StdOut
class is used to print the standard output. This class provides methods to print numbers and strings to standard output. The StdOut
class must be in the classpath
if we want to use it in our code.
Most of the time, the class is included in the auto-installer, and if it is not, the jar
file for StdOut
can be downloaded and added to the classpath
. We can also add the StdOut.Java
in the workplace.
Here is a simple example for StdOut
in Java:
package delftstack;
public class Example {
public static void main(String[] args) {
int Number1 = 14;
int Number2 = 277;
int Number3 = 126;
int Calculation = Number1 + Number2 - Number3;
StdOut.println("Hello, This is delftstack.com");
StdOut.printf("%d + %d - %d = %d\n", Number1, Number2, Number3, Calculation);
}
}
The code above uses StdOut.println
and StdOut.printf
methods to print the standard outputs. The printf
method takes parameters that will be printed in a standard way. See the following result.
OUTPUT:
Hello, This is delftstack.com
14 + 277 - 126 = 165
The StdOut
has different methods to print different standard outputs. See the table below:
Method | Description |
---|---|
print() |
Prints standard output. |
print(boolean x) |
Prints flush the Booleans in standard output. |
print(byte x) |
Prints a byte into the standard output. |
print(char x) |
Prints a char into the standard output. |
print(double x) |
Prints a double into the standard output. |
print(float x) |
Prints a float into the standard output. |
print(int x) |
Prints an integer into the standard output. |
print(long x) |
Prints along into the standard output. |
print(Object x) |
Prints an object into the standard output. |
print(short x) |
Prints a short into the standard output. |
println() |
Prints the line separator string and terminates the current line. |
println(boolean x) |
Prints the Boolean into the standard output and terminates the current line. |
println(byte x) |
Prints the byte into the standard output and terminates the current line. |
println(char x) |
Prints the char into the standard output and terminates the current line. |
println(double x) |
Prints the double into the standard output and terminates the current line. |
println(float x) |
Prints the float into the standard output and terminates the current line. |
println(int x) |
Prints the integer into the standard output and terminates the current line. |
println(long x) |
Prints the long into the standard output and terminates the current line. |
println(Object x) |
Prints the object to this output stream and terminates the current line. |
println(short x) |
Prints the short into the standard output and terminates the current line. |
printf(Locale locale, String format, Object... args) |
Prints a formatted string to the standard output. It takes parameters to print them with the specified standard output. |
printf(String format, Object... args) |
Prints a formatted string to the standard output. It also takes parameters to print them with the standard output. |
main(String[] args) |
Used to unit test some of the methods in StdOut. |
You might wonder if System.out
also do the same work; what is the difference? Here are the differences between StdOut
and System.out
:
- The behaviour of
System.out
andStdOut
is the same, butStdOut
has a few technical differences. - The
StdOut
will flush the output, and the outcome will immediately be seen in the terminal. - The
StdOut
forces the character encoding toUTF-8
to make it standard. - The
StdOut
forces the locale toLocale.US
for consistency and floating point values.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook