What Is an Attribute in Java
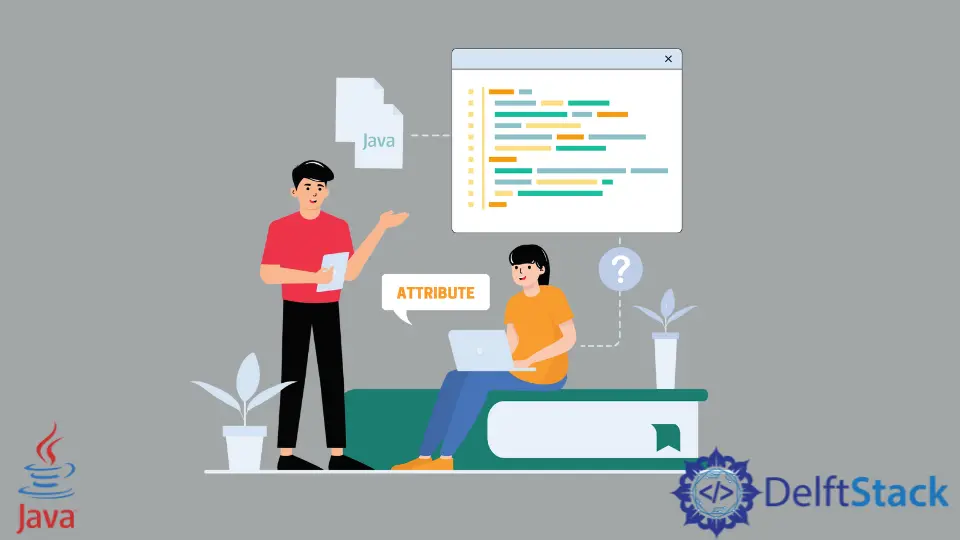
When creating a Class in Java, it contains behaviors and attributes of its own, which helps differentiate one class from another. We will learn more about attributes in this article.
Attributes in a Class in Java
Let us take a person as an example; the Person
class has attributes and behaviors. The Person
class attributes include name, gender, height, weight, and age.
These attributes are characteristics of the Person
class. Behaviors are the tasks that the Person
class can perform.
For example, if the person can speak, eat, dance, sing and, sleep these are the person’s behaviors. In other words, attributes are fields declared inside an object.
These variables belong to an object and are represented with different data types.
As seen in the code below, we have a Person
class with member variables name
and gender
of String
type, age
of int
type, height
and weight
of Double
type. We called the default parameterized constructor to initialize the fields
of this class.
We created a Person
class object using the new
keyword. In Java, we use the dot notation to access an object’s attribute.
For example, to access the name
attribute of the Person
class, we use person.name
. Here, the person is an instance to the class Person
, and name
is an attribute of this class.
We can also use the getter
methods of the class to access the class’s attributes. We have used person.getGender()
to access the gender attribute.
The getter
method returns the value of the attributes in a class.
class Person {
String name;
int age;
String gender;
Double height;
Double weight;
Person(String name, int age, String gender, Double height, Double weight) {
this.name = name;
this.age = age;
this.gender = gender;
this.height = height;
this.weight = weight;
}
public String getName() {
return name;
}
public String getGender() {
return gender;
}
public int getAge() {
return age;
}
public Double getWeight() {
return weight;
}
public Double getHeight() {
return height;
}
public void eat() {
System.out.println(name + " can eat");
}
public void speak() {
System.out.println("Person can talk");
}
public void sleep() {
System.out.println("Person can sleep");
}
}
public class TestClass {
public static void main(String[] args) {
Person person = new Person("John", 30, "male", 158.0, 70.8);
person.eat();
System.out.println("John is a " + person.age + " years old " + person.getGender() + ".");
}
}
Output:
John can eat
John is a 30 years old male.
In Java, there are built-in classes; their attributes are accessible. For example, Array
is a class that has a public attribute length
.
Here, in the code, we have created and initialized an array of int
types with an initial capacity of 4 elements. We can access or get the length of an array using the array.length
.
public class TestLength {
public static void main(String[] args) {
int[] array = new int[4];
System.out.println(array.length);
}
}
Output:
4
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn