What Does Instantiate Mean in Java
- Understanding Instantiation in Java
- The Role of Constructors in Instantiation
- Instance Variables and Methods
- Conclusion
- FAQ
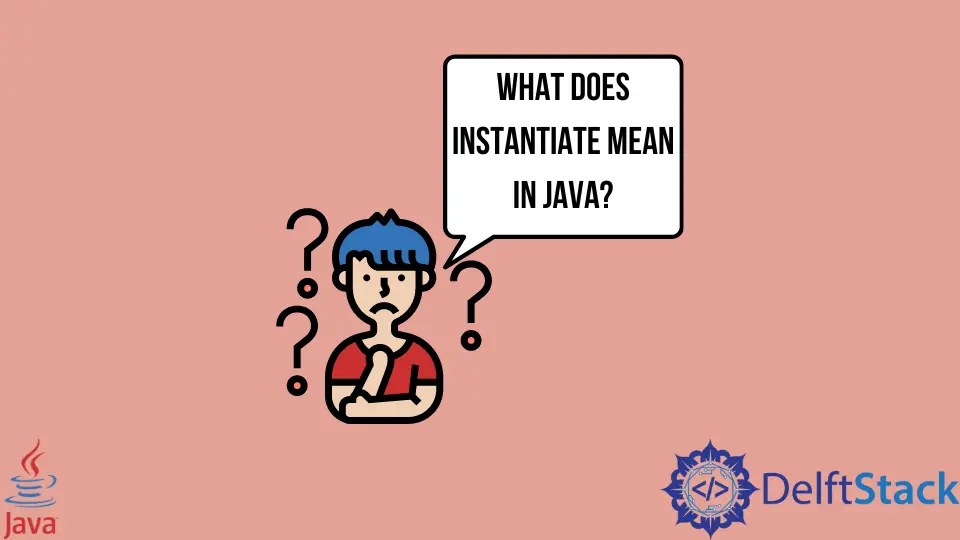
In the world of Java programming, the term “instantiate” is a fundamental concept that every developer should understand. Instantiation refers to the process of creating an instance of a class, which is a blueprint for objects in Java. When you instantiate a class, you are essentially creating a specific object that contains its own unique state and behavior defined by that class.
This article will delve into the meaning of instantiation in Java, how it works, and why it is crucial for effective programming. Whether you are a beginner or looking to brush up on your Java skills, this guide will provide you with the insights you need to grasp this essential concept.
Understanding Instantiation in Java
Instantiation in Java occurs when you use the new
keyword to create an object from a class. When you define a class, you are essentially creating a template that outlines the properties and methods that the objects of that class will have. However, until you create an object from this class, it remains just a blueprint.
Here’s a simple example to illustrate this:
class Car {
String color;
String model;
void display() {
System.out.println("Car model: " + model + ", Color: " + color);
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car();
myCar.color = "Red";
myCar.model = "Toyota";
myCar.display();
}
}
Output:
Car model: Toyota, Color: Red
In this example, we define a Car
class with properties color
and model
, along with a method display()
. In the Main
class, we instantiate the Car
class using the new
keyword, creating an object called myCar
. We then set the properties of myCar
and call the display()
method to show the car’s details. This demonstrates how instantiation allows us to create and manipulate objects based on the class definition.
The Role of Constructors in Instantiation
When you instantiate a class in Java, constructors come into play. A constructor is a special type of method that is called when an object is created. It initializes the new object and can set default values for its attributes. If you don’t define a constructor, Java provides a default constructor that initializes member variables to their default values (e.g., null
for objects, 0
for integers).
Here’s an example that highlights the use of constructors:
class Dog {
String breed;
int age;
Dog(String b, int a) {
breed = b;
age = a;
}
void display() {
System.out.println("Breed: " + breed + ", Age: " + age);
}
}
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog("Labrador", 3);
myDog.display();
}
}
Output:
Breed: Labrador, Age: 3
In this example, the Dog
class has a constructor that takes parameters to set the breed
and age
of the dog. When we create an instance of Dog
, we pass the values “Labrador” and 3 to the constructor. This way, the object is initialized with specific values right from the moment it is instantiated, showcasing the importance of constructors in the process.
Instance Variables and Methods
When you instantiate a class, the object you create can have its own instance variables and methods. Instance variables are specific to each object and hold the state of that object. Methods, on the other hand, define the behavior of the object. Each instance of a class can have different values for its instance variables, but they share the same methods.
Consider the following example:
class Book {
String title;
String author;
Book(String t, String a) {
title = t;
author = a;
}
void read() {
System.out.println("Reading: " + title + " by " + author);
}
}
public class Main {
public static void main(String[] args) {
Book book1 = new Book("1984", "George Orwell");
Book book2 = new Book("To Kill a Mockingbird", "Harper Lee");
book1.read();
book2.read();
}
}
Output:
Reading: 1984 by George Orwell
Reading: To Kill a Mockingbird by Harper Lee
Here, we have two instances of the Book
class: book1
and book2
. Each object has its own title
and author
values. When we call the read()
method on each instance, it displays the respective book details. This demonstrates how instantiation allows you to create multiple objects with unique states while sharing the same functionality.
Conclusion
Understanding instantiation in Java is crucial for any programmer looking to create efficient and effective applications. By grasping how to create objects from classes and the role of constructors, instance variables, and methods, you can harness the full power of object-oriented programming. Whether you are building a simple application or a complex system, instantiation is a key concept that will enhance your coding skills and improve your software design.
FAQ
-
What is the difference between a class and an object in Java?
A class is a blueprint for creating objects, while an object is an instance of a class that contains specific values and behaviors defined by that class. -
Can you instantiate an abstract class in Java?
No, you cannot instantiate an abstract class directly. You must create a subclass that implements the abstract methods and then instantiate that subclass. -
What happens if you don’t use a constructor in Java?
If you don’t define a constructor in your class, Java provides a default constructor that initializes instance variables to their default values. -
How do you create multiple instances of a class in Java?
You can create multiple instances by using thenew
keyword for each object you want to create, allowing each instance to have its own state. -
Why are constructors important in Java?
Constructors are important because they allow you to initialize objects with specific values at the time of instantiation, ensuring that the object is in a valid state.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn