WebSocket Client in Java
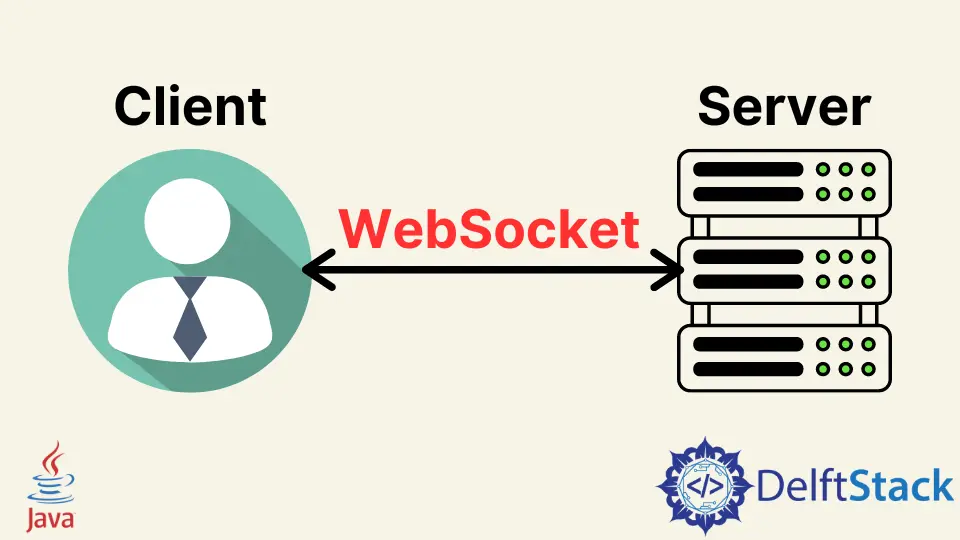
This tutorial demonstrates how to create a WebSocket client in Java.
WebSocket Client in Java
A WebSocket can be used to create a communication channel between the client and server. The WebSocket protocol is compatible with HTTP used for web communication.
The WebSocket protocol has two improvements to HTTP: first, it has a lower overhead than HTTP, and the second is the bi-directional web communication.
Client and server are the parts of the WebSocket communication where the client is used to send and receive data, and the server is used for communication.
Let’s try to create a client using the WebSocket:
-
First, our class needs to be annotated as a
ClientEndpoint
. -
Then, we need to create a
ClientManager
and ask it to connect to the annotated endpoint, which is our client. The URI will specify the server. -
When the connection is established, the logic will be similar to the server.
-
When a method opens, a message is received, or a connection closes the method,
onOpen()
,onMessage()
, oronClose()
will be called automaticaly.
Here is an example of the client using the above points:
package delftstack;
import java.io.*;
import java.net.URI;
import javax.websocket.*;
@ClientEndpoint
public class Client_Endpoint {
@OnOpen
public void onOpen(Session Client_Session) {
System.out.println("--- Connection Successful " + Client_Session.getId());
try {
Client_Session.getBasicRemote().sendText("Start");
} catch (IOException e) {
e.printStackTrace();
}
}
@OnMessage
public String onMessage(String Client_Message, Session Client_Session) {
BufferedReader Buffered_Reader = new BufferedReader(new InputStreamReader(System.in));
try {
System.out.println("--- Message Received " + Client_Message);
String User_Input = Buffered_Reader.readLine();
return User_Input;
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@OnClose
public void onClose(Session Client_Session, CloseReason Close_Reason) {
System.out.println("--- Session ID: " + Client_Session.getId());
System.out.println("--- Closing Reason: " + Close_Reason);
}
public static void main(String[] args) {
ClientManager Client_Manager = ClientManager.createClient();
try {
URI uri = new URI("ws://localhost:8080");
Client_Manager.connectToServer(MyClientEndpoint.class, uri);
while (true) {
}
} catch (DeploymentException | URISyntaxException | InterruptedException e) {
e.printStackTrace();
}
}
}
The code implements a client for a chat-based system. When the connection opens the client will send a message to the server using Client_Session.getBasicRemote().sendText()
.
The server will receive the message and will answer with the same string. This will make the method onMessage()
run in the client.
The onMessage()
method will read the message from user input in this system.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook