How to Fix Void Type Not Allowed Here Error in Java
-
What Is the
void type not allowed here
Error? -
Fix Void Type Not Allowed Here Error in Java - Don’t Print in
main()
Method -
Fix Void Type Not Allowed Here Error in Java - Return a String Instead of Printing in
printMessage1()
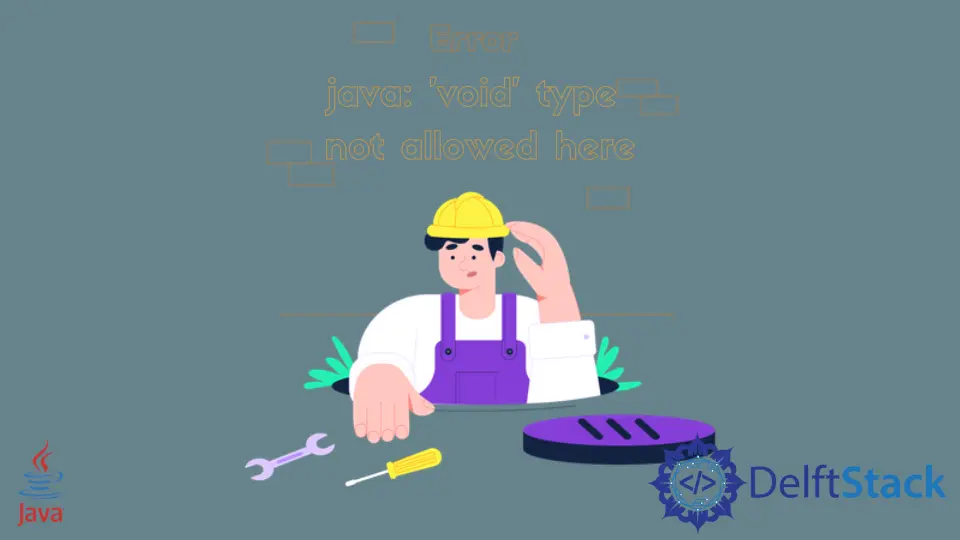
We use many functions when creating big programs in Java, and sometimes errors might appear. One of the errors that the compiler can throw is the void type not allowed here
error discussed in this article.
What Is the void type not allowed here
Error?
We create a function in Java by writing the access modifier, a return type, a function name with parentheses, and the function body is enclosed with curly braces. We can return several types of data from a function, but when we don’t want to return any, we use the keyword void
to tell the compiler that we don’t want to return anything from the method.
In the program below, we have a class JavaExample
that contains two methods, first is the main()
function, and the second is the printMessage1()
that has a print statement System.out.println()
that prints a message that printMessage1()
receives as a parameter.
The function printMessage1()
doesn’t return anything and just prints a message; we use void
type as the return type. We use another print statement but in the main()
method and call the printMessage1()
function inside it with String 1
as an argument.
When we run the code, the output throws an error, void type not allowed here
. It happens because printMessage1()
already has a print statement that prints the value
, and it doesn’t return anything when we call the function in a print statement; there’s nothing to print in the main
method.
public class JavaExample {
public static void main(String[] args) {
System.out.println(printMessage1("String 1"));
}
static void printMessage1(String value) {
System.out.println(value);
}
}
Output:
java: 'void' type not allowed here
Fix Void Type Not Allowed Here Error in Java - Don’t Print in main()
Method
The first solution to this error is that we do not call the function printMessage1()
in a print statement because there is already a System.out.println()
statement in the method itself, and it does not return anything.
In this code, we write the printMessage1()
function’s body as a println()
statement. We call the printMessage1()
method in main()
with a string as an argument.
public class JavaExample {
public static void main(String[] args) {
printMessage1("String 1");
}
static void printMessage1(String value) {
System.out.println(value);
}
}
Output:
String 1
Fix Void Type Not Allowed Here Error in Java - Return a String Instead of Printing in printMessage1()
The second solution is to specify a return type in the function, return a value, and print it wherever we call the function.
We write the method printMessage1()
but with a return type String
. Inside the method’s body, we use the return
keyword with the value
we want to return when called. In the main()
method, we call the function printMessage1()
into a print statement, but there will be no error as the method returns a value.
public class JavaExample {
public static void main(String[] args) {
System.out.println(printMessage1("How are you doing today?"));
System.out.println(printMessage1("String 2"));
}
static String printMessage1(String value) {
return value;
}
}
Output:
How are you doing today?
String 2
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn