How to Use cURL in Java
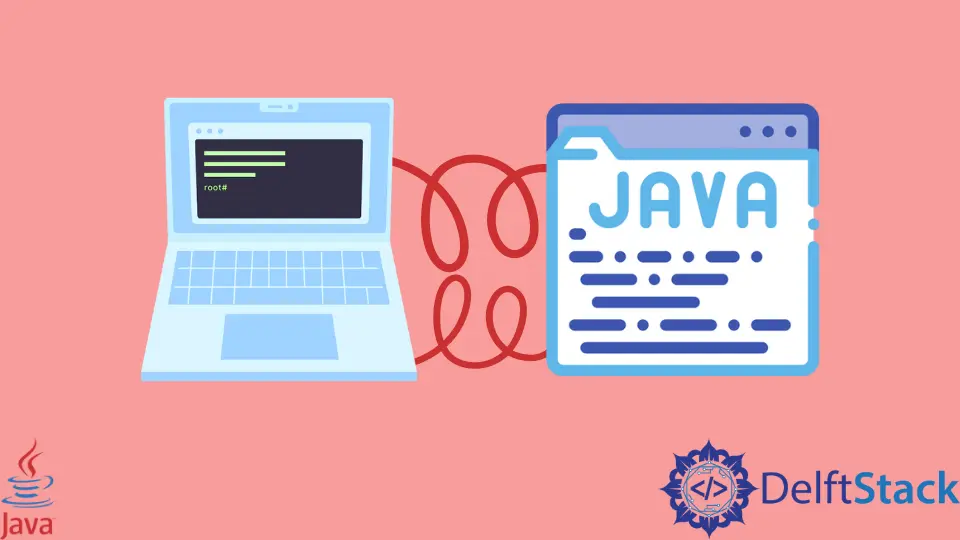
Today, we will learn about cURL
in Java. We will also learn how to use cURL
with ProcessBuilder
and Runtime.getRuntime
.
cURL
in Java
The cURL
is a networking tool that transfers the data between the cURL
client and server. The cURL
uses the protocols like HTTP
, FTP
, TELNET
, and SCP
.
The cURL
works similar to the core java’s java.net.URLConnection
package or Apache’s ApacheHttpClient
. However, the cURL
works in commands, so we may need to use ProcessBuilder
or Runtime.getRuntime()
in Java.
Different Ways to Use cURL
in Java
First, make sure the cURL
is installed on your system. Then, download the cURL
installer from these links and install it on your system.
Method 1: Use cURL
With ProcessBuilder
Once cURL
is installed on the system, we can use it in Java. Here is a simple example of how to use cURL
in Java:
String Demo_Command = "curl -X GET https://localhost/get?delft1=stack1&delft2=stack2";
ProcessBuilder Process_Builder = new ProcessBuilder(Demo_Command.split(" "));
The above code executes the cURL
command with a get request on the given server link. We also need to provide the system path
for cURL
; otherwise, it will not work.
String Demo_Command = "curl -X GET https://localhost/get?delft1=stack1&delft2=stack2";
ProcessBuilder Process_Builder = new ProcessBuilder(Demo_Command.split(" "));
Process_Builder.directory(new File("/home/"));
Process Demo_process = Process_Builder.start();
Now we can get the input stream from the process, and after the processing is complete, we can get the exit code and destroy the process instance.
String Demo_Command = "curl -X GET https://localhost/get?delft1=stack1&delft2=stack2";
ProcessBuilder Process_Builder = new ProcessBuilder(Demo_Command.split(" "));
Process_Builder.directory(new File("/home/"));
Process Demo_process = Process_Builder.start();
InputStream Input_Stream = Demo_process.getInputStream();
int Exit_Code = Demo_process.exitValue();
System.out.println(Exit_Code);
Demo_process.destroy();
For additional cURL
commands, we can reuse the ProcessBuilder
or pass the commands as an array.
Method 2: Use cURL
With Runtime.getRuntime
Another method to run commands in Java is Runtime.getRuntime()
to get the instance of the process class. The Runtime.getRuntime()
is good alternative to use cURL
in Java.
Let’s try to execute cURL
using Runtime.getRuntime
in Java:
String Demo_Command = "curl -X POST https://localhost/post --data delft1=stack1&delft2=stack2";
Process Demo_process = Runtime.getRuntime().exec(Demo_Command);
The code above sends a post request using the cURL
and Runtime.getRuntime
methods. Now we can get the InputStream
with the same method described above.
String Demo_Command = "curl -X POST https://localhost/post --data delft1=stack1&delft2=stack2";
Process Demo_process = Runtime.getRuntime().exec(Demo_Command);
InputStream Input_Stream = Demo_process.getInputStream();
Demo_process.destroy();
Remember, if the instances are no longer required, the system resources should be released using the destroy()
method on the process instances.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook