How to Do Unit Testing in Java
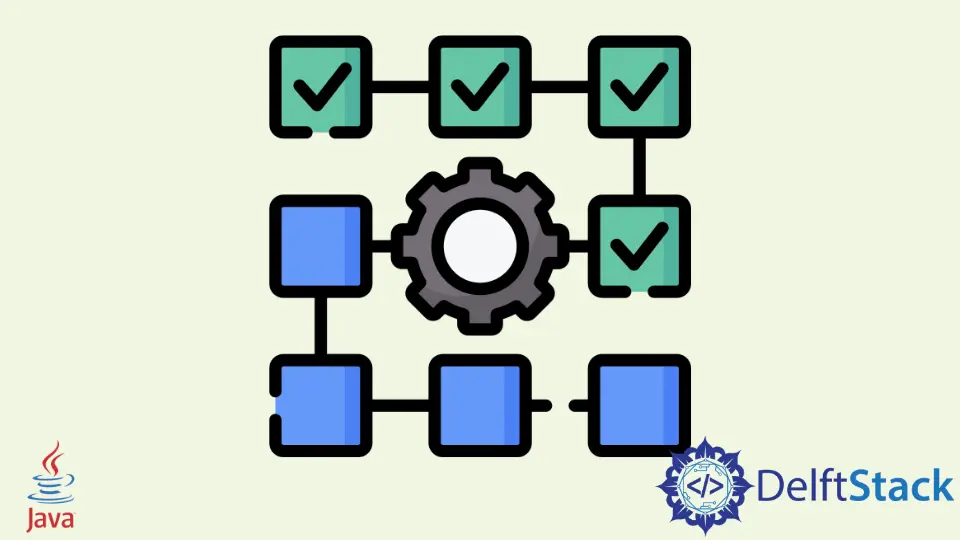
This article will discuss unit testing in Java. There are several types of testing, and unit testing is one of them.
A unit test involves the testing of isolated components like classes and methods. It is effective because we have great control over specific parts of the whole program.
Use JUnit Testing Framework to Unit Test in Java
The following example has a simple program with a calculateOccurrences()
method that returns the total number of occurrences of the specified character in a string.
The method calculateOccurrences()
receives two parameters: the stringToUse
and the second parameter is the characterToFind
.
In the function, we loop through the string and check if any character in the string matches with the characterToFind
and if it does, then increment the charCount
. We call the main()
function with proper arguments.
We need to test it to know that it works properly; we will do that in the next section of this tutorial.
public class JavaTestExample {
public static void main(String[] args) {
int getTotalOccurrences = calculateOccurrences("pepper", 'p');
System.out.println(getTotalOccurrences);
}
public static int calculateOccurrences(String stringToUse, char characterToFind) {
int charCount = 0;
for (int i = 0; i < stringToUse.length(); i++) {
if (stringToUse.charAt(i) == characterToFind) {
charCount++;
}
}
return charCount;
}
}
Output:
3
To unit test the Java program, we use the JUnit testing framework specially made for unit testing in Java. We import it using the following maven dependency.
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
</dependency>
To test different scenarios, we create test cases and then check if the tests pass the given condition or not.
For this example, we use the Intellij IDEA IDE that can be downloaded from https://www.jetbrains.com/idea/
.
We keep the main Java and test files in the same package to save against any access error.
Below is the first test case; we annotate the method with @Test
to make it a test case. JUnit provides us with several assertion methods that help us write the tests.
To test if the returned value is equal to our desire, we use the assertEquals()
method.
The assertEquals()
method that we use in this program takes two arguments; the first is the expected result where we pass 1.
The second argument is the actual returned value by the calculateOccurrences()
method when passing the this is a java example
string and i
as the character to find.
We run the test, and the Run
window pops up in the IDE. Notice that the windows’ title in the output says Tests failed: 1 of 1 test
.
Further, we get the message with AssertionError
, and the expected value to be 1, and the actual value is 2.
The test failed because we expected the result to be 1, which is the number of i’s in the this is a java example
string, but actually, there are two occurrences of i’s in it, so the test failed.
Test Case 1:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class JavaTest {
@Test
public void testCheckChar() {
assertEquals(1, JavaTestExample.calculateOccurrences("this is a java example", 'i'));
}
}
Output:
In the second test case, we use 2 as the expected result with the same string, and in the output, we get a green tick with the message Test Passed:1 of 1 test
. It happens because the expected value is equal to the actual value.
Test Case 2:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class JavaTest {
@Test
public void testCheckChar() {
assertEquals(2, JavaTestExample.calculateOccurrences("this is an java example", 'i'));
}
}
Output:
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn