Undefined Value in Java
- What is Undefined in Java
- What is Null in Java
- Code to Assign a Null Value to a Variable in Java
- What Separates Undefined from Null in Java
- Code to Use a Null Value in Java
- What is the Null Exception Error in Java
- Undefined in Java Generics
- Conclusion
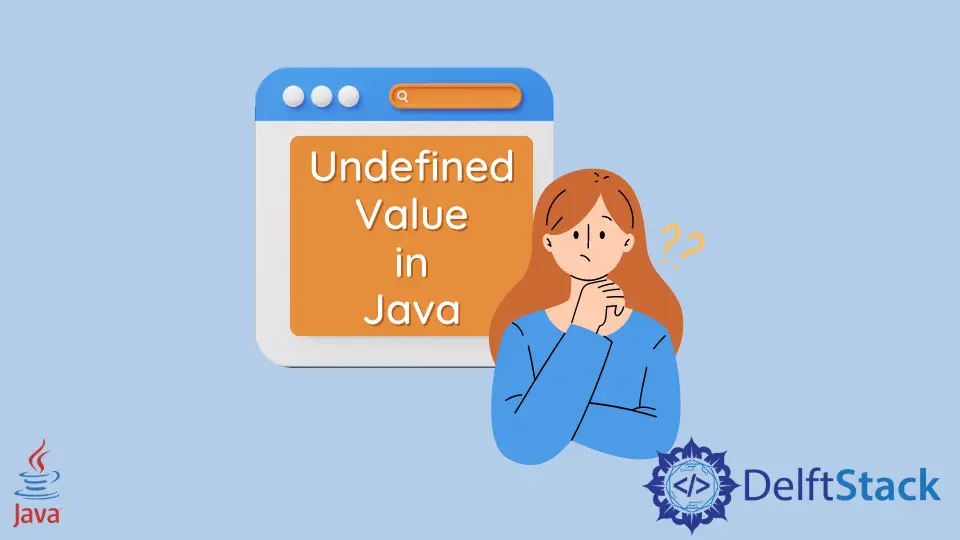
This article aims at highlighting datatypes like undefined and null objects. In the further section of the article, emphasis will be put upon understanding undefined in Java generics and null in Java, with their respective codes and functions.
What is Undefined in Java
An undefined is a form of an empty variable with no defined data type or which is defined but not have been used yet.
undefined
is a datatype in JavaScript and shares many similarities withnull
in Java. In Java, the termundefined
does not exist.- Unknown objects are also identified as undefined in many cases.
Aspects of both will be discussed later in this article.
Let’s start by learning how to initialize undefined variables and then check if it is of undefined data type.
- Create a class
check_null
having a static variable nameda
. - It can be seen that variable
a
does not have any data type defined, so theif-else
condition throws the first condition and prints:Resultant is a null
.
This interprets that by default, variables are designed to get assigned null values when the declaration of a datatype is not done.
public class check_null {
static check_null a;
public static void main(String args[]) throws Exception {
if (a == null) {
System.out.println("Resultant is a null");
} else {
System.out.println("Resultant is not a null");
}
}
}
Output:
Resultant is a null
A lot of programmers get confused between undefined and null. Although they are very different data items, they serve very similar purposes, so we must understand null.
What is Null in Java
Null stands for an absence in value, which means programmers intentionally put this absence in a variable to serve a purpose. It accepts null as a value, meaning it does not store any other value.
For primitive operations, the compiler assigns a zero to it to avoid compilation errors.
Code to Assign a Null Value to a Variable in Java
-
Create a class named
assign_null
. -
In the main method, two string variables are initialized:
str_s1
andstr_s2
. Here, the initialization of the second variable is optional, and a single variable would also do the job. -
The null is stored in the variable
str_s1
and passed tostr_s2
. When both the variables are printed, the resultant output is null for both.Although it may represent an absence of value, Java treats null as a value during operations.
public class assign_null {
public static void main(String[] arg) {
String str_s1 = null;
String str_s2 = str_s1;
System.out.println(str_s1);
System.out.println(str_s2);
}
}
Output:
null
null
What Separates Undefined from Null in Java
Undefined is a data type assigned to those variables where “which datatype to use” is in question. It is left as a placeholder, giving retrospective flexibility with data types.
Null is an intentional absence of value assigned to a variable. The datatype found in this case is undefined, and no other value can be inserted in this datatype except null.
In a real-life example, null can be used to define the emptiness of an object. A printer having no pages can be denoted as null, while it can be defined as empty if there are blank pages.
If there are printed pages, then it can be defined as non-empty.
Code to Use a Null Value in Java
In the below program, different values are assigned to a String
variable, and it is checked which values throw a null, using a checking method.
- A
FindifNull
method is created, whose purpose is to find if our passed value matches with the conditions. - The variable’s contents are checked, finding whether it is empty, filled, or null.
- Then, we take 3 different string inputs in our main function and call our
FindifNull
method to pass the result to the main function.
class useNull {
public static void main(String[] args) {
String er1 = null;
String er2 = "";
String er3 = " ";
System.out.println("\n"
+ "Resultant:" + FindifNull(er1));
System.out.println("\nResultant:" + FindifNull(er2));
System.out.println("\nResultant:" + FindifNull(er3));
}
public static String FindifNull(String str) {
if (str == null) {
return "Null";
} else if (str.isEmpty()) {
return "Not filled";
} else {
return "place filled";
}
}
}
Output:
Resultant:Null
Resultant:Not filled
Resultant:place filled
What is the Null Exception Error in Java
Null provides flexibility with objects in most programming languages, as they can be defined without providing a reference type. These languages use null as a default value to avoid errors while referencing.
But this can cause major errors when more than one object wants to get referenced to the same data storage.
This can be understood better with an illustration of function overloading in Java. Here, two overloaded functions are defined with the same name.
void test(Integer x)
void test(String soap)
As both integer and string are primitive data types, the compiler gets confused and cannot identify the variable it should pass the value to when the object is called.
The main
class creates the object and passes the null to the test
method. If either one of the methods contains a primitive datatype, the compiler would have passed the null to the non-primitive one.
The program below demonstrates how the error is caused when methods are overloaded.
public class check {
public void method(Integer x) {
System.out.println("checking int");
}
public void method(String soap) {
System.out.println("checking if string");
}
// Main class
public static void main(String[] args) {
check obj = new check();
obj.method(null);
}
}
Output:
obj.method(null);
^
both method method(Integer) in check and method method(String) in check match
1 error
The null value must be explicitly supplied to a variable with a non-primitive datatype to remedy this problem. This way, the compiler can be directed to use a particular method.
The code below is almost similar to the above, with slight changes in the main function. The null value is passed to a String variable mv
, and object obj
is created.
At last, this object is passed to the method test
using the variable mv
. This variable helps the compiler in finding the correct reference datatype in it.
public class findthenull {
public void test(Integer x) {
System.out.println("test(Integer )");
}
public void test(String str) {
System.out.println("test(String )");
}
// Main class
public static void main(String[] args) {
String mv = null;
findthenull obj = new findthenull();
obj.test(mv);
}
}
Output:
test(String )
Undefined in Java Generics
As stated at the start of the article, undefined means not a defined data type. Java generics will be employed in the examples below as they can be used to define an object without needing to state its datatype.
Java generics are created to provide safety to parameterized methods. It aids in making classes where a gatekeeper can be placed so that it becomes parameterized.
While generic methods are like normal methods, the main difference is - generic methods are parameterized. Normal functions accept any datatype value, but in cases of incompatible datatypes, the program throws errors or crashes during run time.
Parameters can be put to methods using generics so that the method only accepts values of a specific data type while discards other data types’ values during compilation.
This provides programmers with greater flexibility to problems of typecasting and issues of incompatible data types.
Let’s understand how generics can be used.
- The class
genrics
is defined in the code below. - The method
check
is parameterized, having an objectT
. This objectT
is of an undefined type; thus, its datatype is undefined too, and we use the variabledata
to pass the arguments. - The method checks the value’s data type passed using
instanceof
syntax. If the value passed matches the datatype, we would print that value stating its datatype. - For incompatible data types, we used an
if-else
method to discard them.
public class genrics {
static <T> void check(T data) {
if (data instanceof String)
System.out.println("\nDatatype String has:" + data);
else if (data instanceof Integer)
System.out.println("\nDatatype Integer has:" + data);
else
System.out.println("\nThe datatype used is unsupported:" + data.getClass());
}
public static void main(String[] args) {
check("This is an example");
check(15);
check(5.0);
}
}
Output:
Datatype String has: This is an example
Datatype Integer has:15
The datatype used is unsupported: class java.lang.Double
Conclusion
This article aims at removing confusion associated with an undefined datatype and null objects. It is observed that an undefined data type does not exist in Java, and a null object is used to fulfill that need.
Java generics helped to understand that functions can be defined and called without defining data types of variables. Correct datatypes can be referenced anywhere in the code without striking an error.