Transient in Java
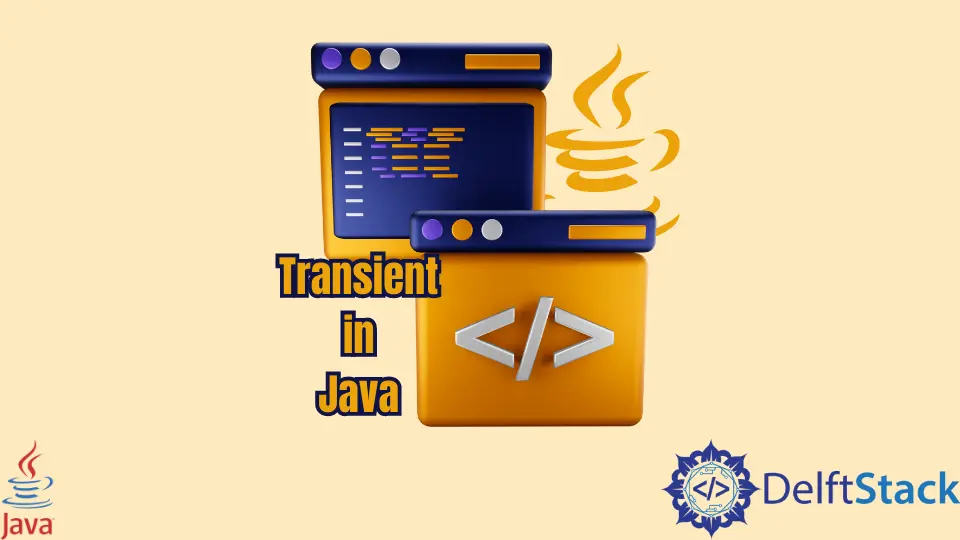
This tutorial introduces what the transient keyword is and how to use it in applications in Java.
Transient is a keyword in Java that is used to mark a variable as non-serializable. It is used when we are serializing an object.
Serialization is the process of transforming a Java object into a stream of bytes. So, it can be persisted to a file, and when we want to avoid any field persisting into a file, we mark that transient.
We cannot mark transient any method, but fields only. Let’s understand with some examples.
To make a class serializable, we need first to implement the Seriablizable
interface and then use the transient keyword with variables. Below is a serializable class example that implements a Serializable
interface. However, it does not mark any field transient.
class Student implements Serializable {
/*
* non- transient fields
*/
int id;
String name;
String email;
}
Make Transient Variable in Java
In this example, we have a class Student
that implements the Serializable
interface and marks the email as transient. So, when we write its states to a file, only name
and id
are written to the file. The email
data is not written. You can verify that by reading the same file and displaying the data to the console. See the example below.
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
public class SimpleTesting {
public static void main(String[] args) throws IOException, ClassNotFoundException {
Student student = new Student();
student.setId(10);
student.setName("Rohan");
student.setEmail("rohan@gmail.com");
// Writing to a file
FileOutputStream fileStream = new FileOutputStream("student.txt");
ObjectOutputStream outStream = new ObjectOutputStream(fileStream);
outStream.writeObject(student);
outStream.flush();
outStream.close();
fileStream.close();
// Reading file
ObjectInputStream inputStream = new ObjectInputStream(new FileInputStream("student.txt"));
student = (Student) inputStream.readObject();
System.out.println(student.id + " " + student.name + " " + student.email);
System.out.println(Student.getSerialversionuid());
inputStream.close();
}
}
class Student implements Serializable {
private static final long serialVersionUID = 1L;
int id;
String name;
transient String email;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public static long getSerialversionuid() {
return serialVersionUID;
}
}
Output:
10 Rohan null 1
You can notice in the output that the email is null.
Make Transient Variable in Hibernate Entity Class in Java
If you are working with hibernate and want to make some variables/fields transient, use the @Transient
modifier. It will skip writing data to the database table. This annotation is helpful only when you are working with hibernate, and since this is an annotation, you must import all the required JARs to your project. See the example below.
@Entity
class Student implements Serializable {
@Id int id;
String name;
@Transient String email; // transient
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}