Super Constructor in Java
-
Using the
super()
With No-Argument Constructor in Java -
Using the
super()
With Parameterized Constructor in Java
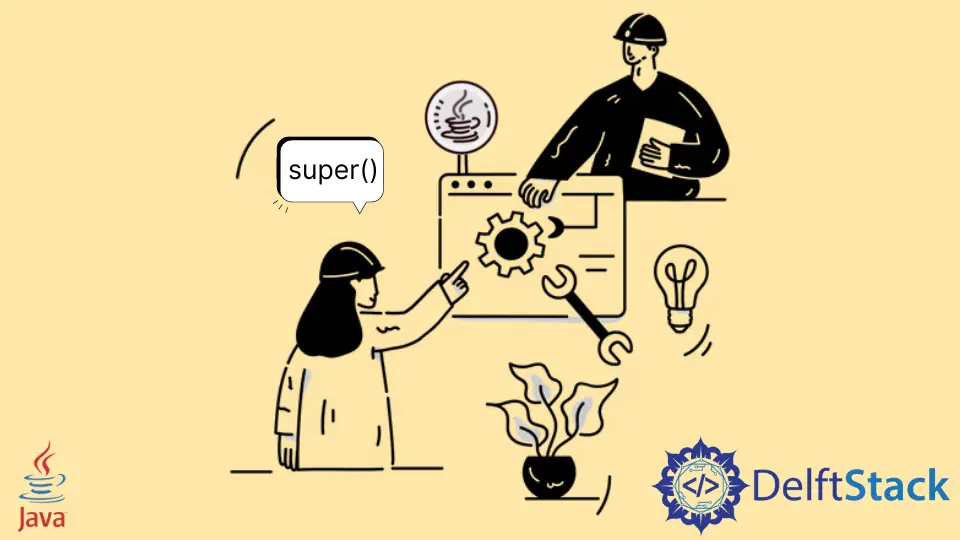
This tutorial will discuss the super
keyword to call the parent class’s variables, functions, and constructors from its subclasses.
The following sections show how to use the super()
to call the constructor of the sub-class parent.
Using the super()
With No-Argument Constructor in Java
The super
keyword comes into usage when we use the concept of inheritance in Java.
When we inherit a class using the keyword extends
, we get the inherited class: a parent class or a superclass, and the class that inherits the parent is called the child class or a subclass.
We use super()
to call the parent class’s constructor. Still, suppose we want to call the default constructor or the constructor without any arguments of the parent class.
In that case, we do not need to call super()
because it is called automatically when the constructor is created.
To understand it better, let us see two examples. In the first example below, we have three classes.
In the Vehicle
class, we print a message in its no-argument constructor. The Motorcycle
class inherits the Vehicle
using the extends
keyword, making Vehicle
a superclass and Motorcycle
a subclass.
We print a message like the Vehicle
constructor in the Motorcycle
class. When we create an object of Motorcycle
using the new
keyword, the class’ constructor is called.
Notice that the constructor of the Vehicle
class is also called the Motorcycle
constructor. It happens because there is a super()
attached to every no argument and default constructor call.
class Vehicle {
Vehicle() {
System.out.println("Vehicle Class Constructor Called");
}
}
class Motorcycle extends Vehicle {
Motorcycle() {
System.out.println("Motorcycle Class Constructor Called");
}
}
class ExampleClass1 {
public static void main(String[] args) {
new Motorcycle();
}
}
Output:
Vehicle Class Constructor Called
Motorcycle Class Constructor Called
Using the super()
With Parameterized Constructor in Java
Unlike the no-argument constructor automatically calls the super()
, the parameterized constructor does not call it, and we need to call it with the arguments.
In the example, we have the same classes as the above program, but the constructor of the Vehicle
class receives a parameter in this program.
Now, if we try to call the super()
from the constructor of Motorcycle
, we get an error because the Vehicle
constructor needs a parameter.
To resolve this, we need to pass an argument in the super()
that is passed in the constructor of the Motorcycle
when the object is created.
class Vehicle {
Vehicle(String str) {
System.out.println("Vehicle Class Constructor Called");
}
}
class Motorcycle extends Vehicle {
Motorcycle(String str) {
super(str);
System.out.println("Motorcycle Class Constructor Called");
}
}
class ExampleClass1 {
public static void main(String[] args) {
new Motorcycle("example string");
}
}
Output:
Vehicle Class Constructor Called
Motorcycle Class Constructor Called
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn