Struct in Java
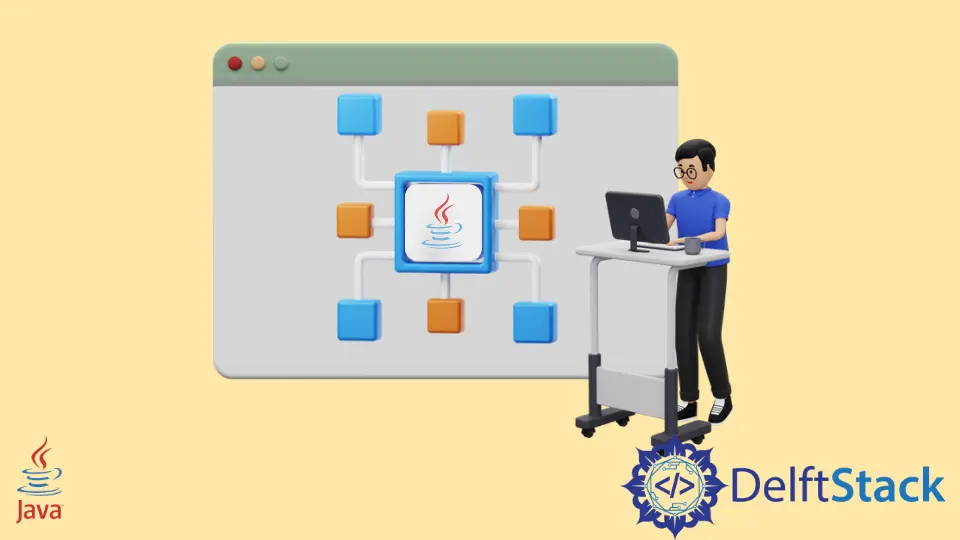
In programming, the struct is a keyword for creating a structure that contains variables, methods, different types of constructors, operators, etc. It is similar to classes that hold different types of data and has a value type. It creates objects which require less memory.
However, structs are not present in Java. We can modify some objects in Java to use them as a struct.
These methods are discussed below.
Use the Classes to Simulate a Struct in Java
We can make all the methods in a class public to simulate a struct. The main difference between a struct and a class is that the struct is by default public, and the class is private. So, if we create a class and change its methods and variables to public, it will work similarly to a struct.
We implement this logic in the following example.
class EmployeeExample {
private String emp_name;
private int emp_code;
// constructor
public Employee(String emp_name, int emp_code) {
this.emp_name = emp_name;
this.emp_code = emp_code;
}
// getter
public String getName() {
return emp_name;
}
public int getCode() {
return emp_code;
}
public static void main(String[] args) {
EmployeeExample[] array = new EmployeeExample[2]; // new stands for create an array object
array[0] = new EmployeeExample("Ram", 1); // new stands for create an employee object
array[1] = new EmployeeExample("Shyaam", 2);
for (int i = 0; i < array.length; i++) {
System.out.println(array[i].getName() + " " + array[i].getCode());
}
}
}
Output:
Ram 1
Shyaam 2
In the above example, we have created the constructors and getName()
and getCode()
methods as public. Then, the code takes employee name and employee code input and stores the values in emp_name
and emp_code
, respectively, in the class EmployeeExample
.
Use the JUnion Plugin to Simulate a Struct in Java
We can also use the Project JUnion plugin. Here we get software that helps us to create struct by using @Struct
annotation. We have to install the plugin from the site github.io/junion and place it in the maven dependency. Also, we have to add the jar file name junion.jar
, which can be downloaded from the same website.
The @Struct
annotation consists of methods that help us to create structure in Java.
The following example demonstrates the above method.
import theleo.jstruct.Struct;
public class Struct {
@Struct
public static class Emp_Name {
public String first_Name, middle_Name, last_Name;
}
public static void main(String[] args) {
Emp_Name[] array = new Emp_Name[2];
array[0].first_Name = "Ram";
array[0].middle_Name = "";
array[0].last_Name = "Sharma ";
System.out.println(
"Name : " + array[0].first_Name + " " + array[0].middle_Name + " " + array[0].last_Name);
}
}
Output:
Name : Ram Sharma
Here we make a static public class, Emp_Name
, which acts as a struct and consists of a few methods. Then in the main class, we created a new array of length two and then added data using the methods and store them in the array.