How to Start a new thread in Java
-
Create a thread by extending the
Thread
class in Java -
Create thread by implementing
Runnable
interface in Java -
Create thread by the
Thread
Object in Java - Create thread using the anonymous class in Java
- Create thread using the lambda function in Java
-
Create thread using the
Executors
class in Java
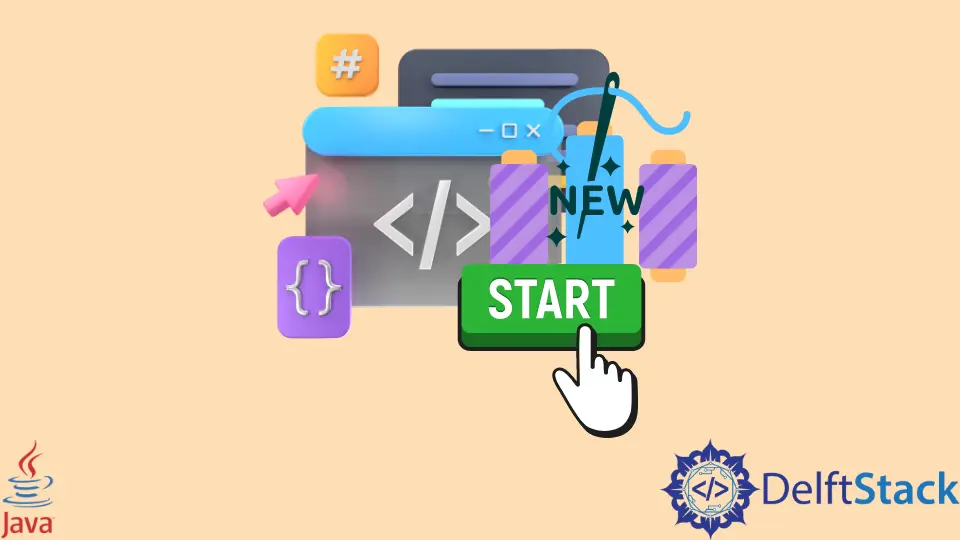
This tutorial introduces how to start a new thread in Java.
A thread is the smallest unit of a program/process that executes in real-time and maximizes CPU utilization.
Java supports the thread concept and allows to create a thread to create multithreading applications. In this article, we will learn to create a new thread in Java.
To create a new thread in Java, we can use either the Thread
class or the Runnable
interface. Let’s see some examples.
Create a thread by extending the Thread
class in Java
In Java, Thread
is a class used to create a new thread and provides several utility methods. In this example, we used the Thread
class by extending it and then starting by using the start()
method. The start()
method starts a new thread. The run()
method is used to perform the task by the newly created thread. The thread automatically runs this method. See the example below.
public class SimpleTesting extends Thread {
public static void main(String[] args) {
SimpleTesting st = new SimpleTesting();
st.start();
}
public void run() {
try {
System.out.println("New Thread Start");
Thread.sleep(1000);
System.out.println("Thread sleep for 1 second.");
} catch (InterruptedException e) {
System.out.println(e);
}
}
}
Output:
New Thread Start
Thread sleep for 1 second.
Create thread by implementing Runnable
interface in Java
The Runnable
interface can be used to create a new thread, but it does not provide such features as provided by the Thread class. This interface provides a run() method that can be used to perform tasks. See the example below.
public class SimpleTesting implements Runnable {
public static void main(String[] args) {
SimpleTesting st = new SimpleTesting();
Thread one = new Thread(st);
one.start();
}
public void run() {
try {
System.out.println("New Thread Start");
Thread.sleep(1000);
System.out.println("Thread sleep for 1 second.");
} catch (InterruptedException e) {
System.out.println(e);
}
}
}
Output:
New Thread Start
Thread sleep for 1 second.
Create thread by the Thread
Object in Java
We can directly create a new thread using the Thread
object and start()
method, but this thread does not perform any task since we did not provide the run()
method implementation. We can get thread information by using its built-in methods such as getState()
, getName()
, etc. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
Thread one = new Thread("New Thread");
System.out.println("Thread state : " + one.getState());
one.start();
System.out.println("Thread state : " + one.getState());
System.out.println("Thread Name : " + one.getName());
System.out.println("Thread state : " + one.getState());
}
}
Output:
Thread state : NEW
Thread state : RUNNABLE
Thread Name : New Thread
Thread state : TERMINATED
Create thread using the anonymous class in Java
This is not a new way to create a new thread; we just used an anonymous class by using the Runnable
class, passed it to the Thread
constructor, and started the thread. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
Thread t1 = new Thread(new Runnable() {
public void run() {
try {
System.out.println("New Thread Start");
Thread.sleep(1000);
System.out.println("Thread sleep for 1 second.");
} catch (InterruptedException e) {
System.out.println(e);
}
}
});
t1.start();
}
}
Output:
New Thread Start
Thread sleep for 1 second.
Create thread using the lambda function in Java
Lambda function feature was added to Java 8 version that can be used to substitute anonymous class in Java. Here, we used the lambda function to create a new thread. This is a compact and short way to create a thread. See the example below.
public class SimpleTesting {
public static void main(String[] args) throws InterruptedException {
new Thread(() -> {
try {
System.out.println("New Thread Start");
Thread.sleep(1000);
System.out.println("Thread sleep for 1 second.");
} catch (InterruptedException e) {
System.out.println(e);
}
}) {
{ start(); }
}.join();
}
}
Output:
New Thread Start
Thread sleep for 1 second.
Create thread using the Executors
class in Java
The Executors
is a class that belongs to the Java concurrency package and can be used to create multithreading applications. So, we can use it to create a new thread also. The newSingleThreadExecutor()
method initializes a new thread and is then executed by the submit()
function. See the example below.
import java.util.concurrent.Executors;
public class SimpleTesting {
public static void main(String[] args) throws InterruptedException {
Executors.newSingleThreadExecutor().submit(() -> {
try {
System.out.println("New Thread Start");
Thread.sleep(1000);
System.out.println("Thread sleep for 1 second.");
} catch (InterruptedException e) {
System.out.println(e);
}
});
}
}
Output:
New Thread Start
Thread sleep for 1 second.