SMS API in Java
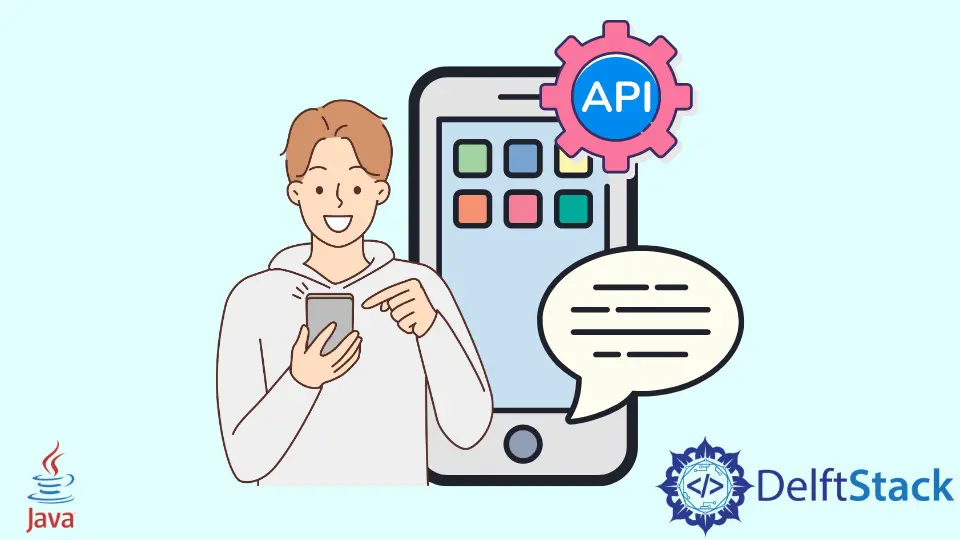
This tutorial demonstrates how to send SMS using an API in Java.
SMS API in Java
Several APIs are provided out there to send SMS using Java code. Some of these APIs are paid, and some are open source.
Text Magic
is one of the most popular APIs to send SMS using different programming languages. The Text Magic
API Java wrapper includes all necessary API tests and commands.
There are different ways to install the Text Magic
API in your IDE. The requirements for this API are:
- Apache Maven 2.0 or above
- Java SE6 or above
Use Eclipse to Install TextMagic
API
Follow the steps below to install the TextMagic
API using Eclipse IDE.
-
Go to
File
and selectImport
. -
Select
Git
>Projects from Git
. -
Select
Clone URI
. -
Paste
https://github.com/textmagic/textmagic-rest-java
in the URI. -
Keep pressing
Next
until you reach this page: -
Click
Finish
, and a new project from Git will be added to your Eclipse.
Use Maven to Quickly Install TextMagic
API
One of the easiest ways to install the TextMagic
API is to add the Dependency
to our existing maven project. The dependency is below with the newest version.
<dependency>
<groupId>com.textmagic.sdk</groupId>
<artifactId>textmagic-java-sdk</artifactId>
<version>1.2.0</version>
</dependency>
Use Git to Manually Install TextMagic
API
We can also install the TextMagic
using the Git command from the TextMagic
GitHub repository. Run the following commands.
-
To download the
textmagic
from Git:git clone git://github.com/textmagic/textmagic-rest-java.git
-
Set the directory to
textmagic
:cd textmagic-rest-java
-
Install
mvn
:mvn install
Generate API_V2
Key
To send an SMS through the TextMagic
API, you need to generate an API_V2
key for a username. Follow the steps below to obtain an API_V2
key for your username.
- First, we must log in to
TextMagic
. Start a free trial if you haven’t registered, as SMS APIs are mostly paid. - Go to the API settings page.
- Click the button
Add new API key
. - Pick any name to add it to
Enter an app name for this key
. - Now click
Generate New Key
. - Once the key is generated, it will show in the green bar above the page or table.
Once the API_V2
key is generated, it can be used along with the username to send SMS through the Java code.
Example of TextMagic
API in Java
Once the TextMagic
API is successfully installed and the key is generated, we can send SMS using Java. Here is an example.
package test.java.com.textmagic.sdk;
import com.textmagic.sdk.RestClient;
import com.textmagic.sdk.RestException;
import com.textmagic.sdk.resource.instance.*;
import java.util.*;
public class TextMagic_Demo {
public static void main(String... strings) throws RestException {
RestClient SMS_Client = new RestClient("User Name", "APIv2 Key");
TMNewMessage SMS_Message = SMS_Client.getResource(TMNewMessage.class);
SMS_Message.setText("Hello this is delftstack.com");
SMS_Message.setPhones(Arrays.asList(new String[] {"99900000"}));
try {
SMS_Message.send();
} catch (final RestException e) {
System.out.println(e.getErrors());
throw new RuntimeException(e);
}
System.out.println(SMS_Message.getId());
}
}
The code above will send an SMS with the given message and phone number. We also need to add the User Name
and APIv2 Key
generated from the above step.
Go to this link for info on the API.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook