The Difference Between Size and Length in Java
-
the
length
Property of Arrays in Java -
Example of
.length
in an Array in Java -
Example of the
size()
Method in an Array in Java -
Find the Length Using the
length()
Method in Java -
Java Collections
size()
Method - the Difference Between Size and Length in Java
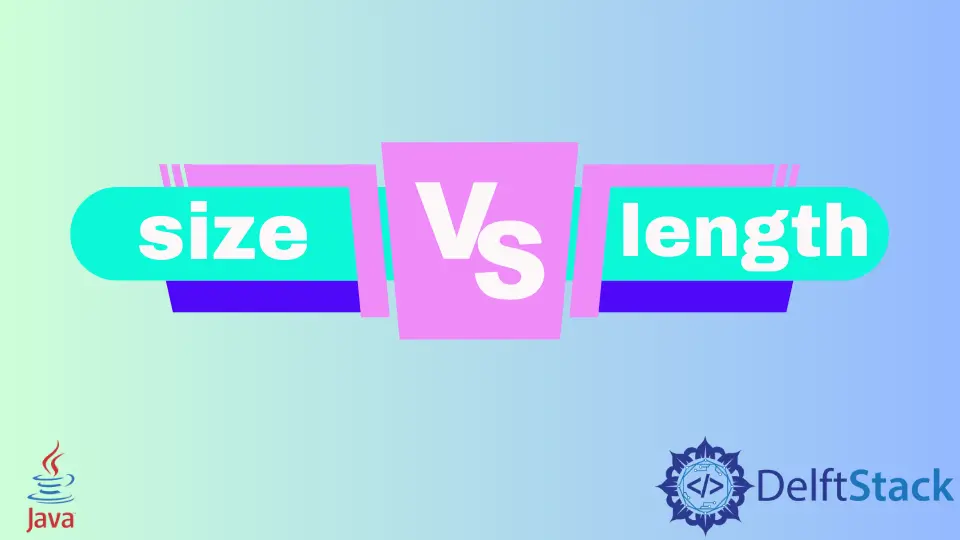
This tutorial introduces the difference between size and length in Java. We also listed some example codes to help you understand the topic.
Java has a size()
method and a length
property. A beginner may think these are interchangeable and perform the same task because they sound somewhat the same. In Java, size and length are two different things. Here, we will learn the differences between the two.
the length
Property of Arrays in Java
Arrays store a fixed number of data of the same type in an ordered fashion. All arrays in Java have a length field that stores the space allocated for the elements of that array. It is a constant value used to find out the maximum capacity of the array.
- Remember that this field will not give us the number of elements present in the array but the maximum number of elements that can be stored (irrespective of whether elements are present or not).
Example of .length
in an Array in Java
In the code below, we first initialize an array of length 7
. The length field of this array shows 7
even though we did not add any elements. This 7
just denotes the maximum capacity.
public class Main {
public static void main(String[] args) {
int[] intArr = new int[7];
System.out.print("Length of the Array is: " + intArr.length);
}
}
Output:
Length of the Array is: 7
Now, let’s add 3 elements to the array using their index and then print the length field. It still shows 7.
public class Main {
public static void main(String[] args) {
int[] intArr = new int[7];
intArr[0] = 20;
intArr[1] = 25;
intArr[2] = 30;
System.out.print("Length of the Array is: " + intArr.length);
}
}
Output:
Length of the Array is: 7
The length field is constant because arrays are fixed in size. We must define the maximum number of elements we will store in the array (the array’s capacity) during initialization, and we cannot exceed this limit.
Example of the size()
Method in an Array in Java
There is no size()
method for arrays; it will return a compilation error. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int[] intArr = new int[7];
System.out.print("Length of the Array is: " + intArr.size());
}
}
Output:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Cannot invoke size() on the array type int[]
at SimpleTesting.main(SimpleTesting.java:7)
Find the Length Using the length()
Method in Java
Java strings are simply an ordered collection of characters, and unlike arrays, they have a length()
method and not a length
field. This method returns the number of characters present in the string.
See the example below.
public class Main {
public static void main(String[] args) {
String str1 = "This is a string";
String str2 = "Another String";
System.out.println("The String is: " + str1);
System.out.println("The length of the string is: " + str1.length());
System.out.println("\nThe String is: " + str2);
System.out.println("The length of the string is: " + str2.length());
}
}
Output:
The String is: This is a string
The length of the string is: 16
The String is: Another String
The length of the string is: 14
Note that we cannot use the length
property on strings and the length()
method is not applicable on arrays. The following code shows the errors if we misuse them.
public class SimpleTesting {
public static void main(String[] args) {
String str1 = "This is a string";
System.out.println("The length of the string is: " + str1.length);
}
}
Output:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
length cannot be resolved or is not a field
at SimpleTesting.main(SimpleTesting.java:7)
Similarly, we cannot use the string length()
method on arrays.
public class SimpleTesting {
public static void main(String[] args) {
int[] intArray = {1, 2, 3};
System.out.println("The length of the string is: " + intArray.length());
}
}
Output:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Cannot invoke length() on the array type int[]
at SimpleTesting.main(SimpleTesting.java:7)
Java Collections size()
Method
size()
is a method of the java.util.Collections
class. The Collections
class is used by a lot of different collections (or data structures) like ArrayList
, LinkedList
, HashSet
, and HashMap
.
The size()
method returns the number of elements currently present in the collection. Unlike the length
property of arrays, the value returned by the size()
method is not constant and changes according to the number of elements.
All the collections of the Collection Framework
in Java are dynamically allocated, so the number of elements can vary. The size()
method is used to keep track of the number of elements.
In the code below, it is clear that when we create a new ArrayList
without any elements in it, the size()
method returns 0.
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> list = new ArrayList<Integer>();
System.out.println("The ArrayList is: " + list);
System.out.println("The size of the ArrayList is: " + list.size());
}
}
Output:
The ArrayList is: []
The size of the ArrayList is: 0
But this value changes as we add or remove elements. After adding three elements, the size increases to 3. Next, we removed two elements, and the size of the list becomes 1.
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> list = new ArrayList<Integer>();
System.out.println("The ArrayList is: " + list);
System.out.println("The size of the ArrayList is: " + list.size());
list.add(20);
list.add(40);
list.add(60);
System.out.println("\nAfter adding three new elements:");
System.out.println("The ArrayList is: " + list);
System.out.println("The size of the ArrayList is: " + list.size());
list.remove(0);
list.remove(1);
System.out.println("\nAfter removing two elements:");
System.out.println("The ArrayList is: " + list);
System.out.println("The size of the ArrayList is: " + list.size());
}
}