Servlet in Java
- What Is Servlet
- Advantages of Using Servlet
- How to Create a Servlet in Java
- Important Packages for Servlet
- Servlet Classes
- Servlet Interfaces
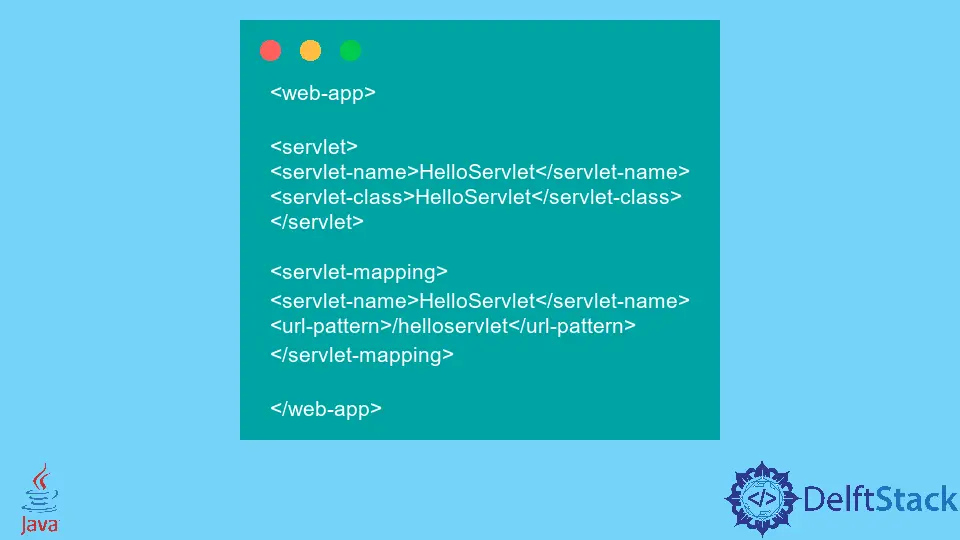
This tutorial introduces what servlet is and how to create a servlet in Java.
What Is Servlet
Servlet is a server-side technology that is used to create web applications in Java. It creates dynamic web applications and provides an API consisting of several classes and interfaces to manage the application.
Advantages of Using Servlet
Before servlet, a CGI (Common Gateway Interface
) program was used to create server-side programs that had several performance issues. Servlet has the edge over CGI and provides the following advantages:
- Higher Performance due to multithreading concept
- No platform dependency
- Portable
- Secure and safe due to Java byte code.
How to Create a Servlet in Java
There are several ways to create a servlet application, such as using any of the following classes or interfaces:
- Implement
Servlet
Interface - Inherit
GenericServlet
Class - Inherit
HttpServlet
Class
These are some classes and interfaces that are used to create a servlet application. They contain methods such as doPost()
and doGet()
to handle HTTP requests.
A servlet application follows a specific directory/project structure and consists of some required steps such as:
- Create a servlet
- Create a deployment descriptor
- Deploy servlet application to a server (apache tomcat).
As we stated earlier, to create a servlet, we need to extend the HttpServlet
class and override its methods. Here, we are using the doGet()
method to handle the Get requests.
The below is a basic servlet code.
Create a Servlet
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
// Extending HttpServlet class
public class HelloServlet extends HttpServlet {
private String msg;
public void init() throws ServletException {
// Do required initialization
msg = "Hello Servlet";
}
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Setting content type for response
response.setContentType("text/html");
// printing msg to browser in h1 heading
PrintWriter out = response.getWriter();
out.println("<h1>" + msg + "</>");
}
public void destroy() {
// code to execute while destroy servlet.
}
}
Output:
This servlet code will print the below message to the browser.
Create the Deployment Descriptor
This is an XML file that contains information on the servlet, and the web container gets all the information from this file. This file uses several tags such as <web-app>
, <servlet>
, <servlet-mapping>
, etc.
They all are used to set information for the container. The container uses a parser to extract this information.
See a servlet descriptor (web.xml) for our HelloServlet class.
<web-app>
<servlet>
<servlet-name>HelloServlet</servlet-name>
<servlet-class>HelloServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>HelloServlet</servlet-name>
<url-pattern>/helloservlet</url-pattern>
</servlet-mapping>
</web-app>
After creating this XML file, compile the servlet file and deploy the complete project to a web server such as apache tomcat. This is a web application, so it requires a server to run.
After deploying the project, start the server and access the servlet by entering the browser’s mapping URL helloservlet
.
Important Packages for Servlet
Servlet API contains two main packages that contain all the classes and interfaces.
javax.servlet
javax.servlet.http
Servlet Classes
These are some frequently used servlet classes:
GenericServlet
ServletInputStream
ServletOutputStream
ServletRequestWrapper
ServletResponseWrapper
ServletRequestEvent
ServletContextEvent
HttpServlet
Cookie
HttpServletRequestWrapper
HttpServletResponseWrapper
HttpSessionEvent
Servlet Interfaces
These are some frequently used servlet interfaces:
Servlet
ServletRequest
ServletResponse
RequestDispatcher
ServletConfig
ServletContext
Filter
FilterConfig
FilterChain
ServletRequestListener
HttpServletRequest
HttpServletResponse
HttpSession
HttpSessionListener