Sentinel Value in Java
- Introduction to Sentinel Values
- Common Use Cases for Sentinel Values
- Implementation of Sentinel Values in Different Kinds of Loops
- Best Practices in Using Sentinel Values
- Conclusion
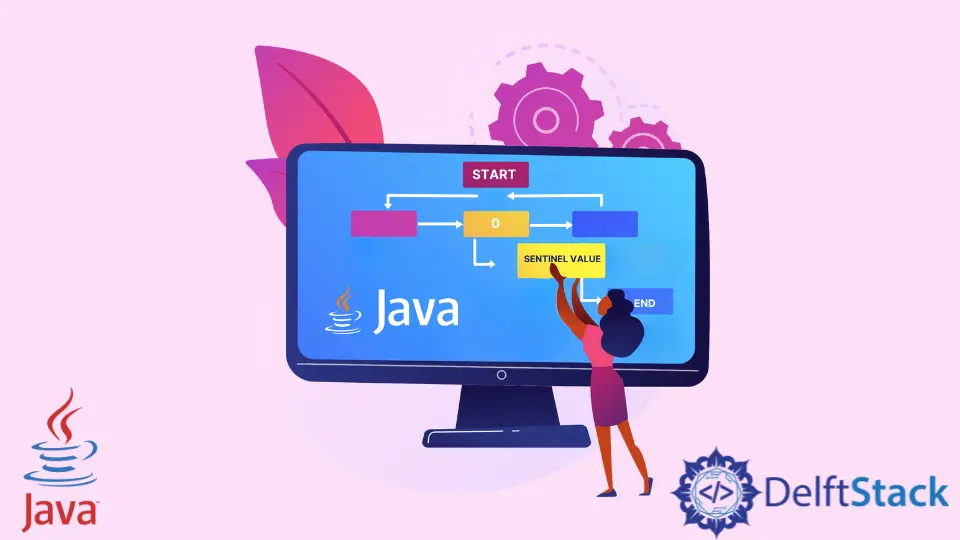
In a programming context, sentinel is a specific value used to terminate a condition in a recursive or looping algorithm. Sentinel value is used in many ways, such as dummy data, flag data, rouge value, or signal value.
Introduction to Sentinel Values
Sentinel values are special markers in programming used to signal the end of data input or termination of a loop. Typically distinct from regular data, they help control program flow.
For instance, in a user input scenario, a sentinel value like -1 might signify the end of input. These values simplify handling variable input lengths and enhance code readability.
Common Use Cases for Sentinel Values
Sentinel values find common use in programming scenarios where the exact number of inputs is unknown and a clear indication of input termination is required. One prevalent use case is in input validation, especially when receiving user inputs.
For instance, a program may prompt users to enter a series of integers until they input a specific sentinel value, signifying the end of their input. Sentinel values are also frequently employed in loops to control their execution, allowing the program to exit when a predetermined condition, signaled by the sentinel value, is met.
This flexibility makes sentinel values a valuable tool for handling variable input lengths and improving the overall flow and logic of programs.
Implementation of Sentinel Values in Different Kinds of Loops
In Java programming, the use of sentinel values is a powerful technique for managing input, loop termination, and overall program flow. A sentinel value acts as a marker, often a special numeric or string value, to signal specific conditions like the end of user input or loop termination.
In this section, we’ll explore the implementation of sentinel values using various methods, including special numeric values, specific strings, constants, enumerations, boolean
flags, exceptions, and break statements in different loop structures like while
, do-while
, and for
.
Let’s consider a scenario where we prompt a user to enter a series of positive integers until they input a sentinel value, such as -1, to indicate the end of their input. We will explore how sentinel values can be applied to achieve this in different loop structures.
import java.util.Scanner;
public class SentinelValueExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Using a sentinel value in a while loop
int userInput;
System.out.println("Enter positive integers (input -1 to stop):");
while ((userInput = scanner.nextInt()) != -1) {
System.out.println("Processing input: " + userInput);
}
// Using a sentinel value in a do-while loop
System.out.println("Enter positive integers (input -1 to stop):");
do {
userInput = scanner.nextInt();
System.out.println("Processing input: " + userInput);
} while (userInput != -1);
// Using a sentinel value in a for loop
System.out.println("Enter positive integers (input -1 to stop):");
for (;;) {
userInput = scanner.nextInt();
if (userInput == -1) {
break;
}
System.out.println("Processing input: " + userInput);
}
scanner.close();
}
}
In this Java example, a Scanner
is utilized to gather user input. The program prompts users to input positive integers until the sentinel value of -1 is entered.
The while
loop checks if the user input is not equal to -1.
If true, the loop continues processing the input and prompting for the next one. The loop terminates when the user inputs -1.
The do-while
loop ensures the code block is executed at least once, as it checks the condition after the block’s execution. Similar to the while
loop, it processes input and continues until the sentinel value is entered.
The for
loop is set up as an infinite loop (for (; ; )
). It relies on the break
statement to exit the loop when the sentinel value is encountered.
This approach provides flexibility in handling user input scenarios and demonstrates the versatility of sentinel values in different loop structures.
Output:
The program effectively processes the user inputs until the sentinel value (-1) is encountered in each loop structure.
Sentinel values are versatile and crucial for handling variable input scenarios, ensuring efficient program execution and user interaction. By understanding their implementation in different loop structures, Java developers can enhance the flexibility and readability of their code.
Best Practices in Using Sentinel Values
Clear and Meaningful Naming
Choose sentinel values with names that clearly convey their purpose, making code more readable and understandable.
Avoiding Ambiguity
Select values that are unlikely to be mistaken for valid data, preventing confusion and unintended program behavior.
Documentation
Clearly document the use of sentinel values in comments or documentation, providing context and guidance for future developers.
Consistency Across Codebase
Maintain uniformity in the choice and usage of sentinel values throughout your code to enhance code coherence and maintainability.
Range Considerations
When using numeric sentinel values, be mindful of the valid data range to avoid conflicts with actual inputs or potential overflow issues.
Handling User Input
When involving user input, design user-friendly prompts or messages to inform users about the expected sentinel values and input termination.
Exceptional Handling (Optional)
Consider using exception handling mechanisms for sentinel values in situations where exceptions provide a cleaner and more structured exit strategy.
Conclusion
Sentinel values serve as crucial markers in programming, particularly in the context of loop structures. This article explored the fundamental concept of sentinel values and provided practical insights into their implementation across different types of loops, including while
, do-while
, and for
loops in Java.
By adopting sentinel values, developers can efficiently manage user input and loop termination conditions, enhancing code flexibility. The article also highlighted best practices, emphasizing clear naming, avoiding ambiguity, and consistent usage.
Incorporating these practices ensures that sentinel values contribute to code clarity and maintainability, offering a valuable tool for effective programming.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn