What is Reflection in Java
- Class Object
-
Class
forName()
Method in Java -
Class
getClass()
Method in Java - Useful Methods for Reflection in Java
- Useful Methods for Reflection Upon Methods
- Useful Methods for Reflection Upon Fields
- Conclusion
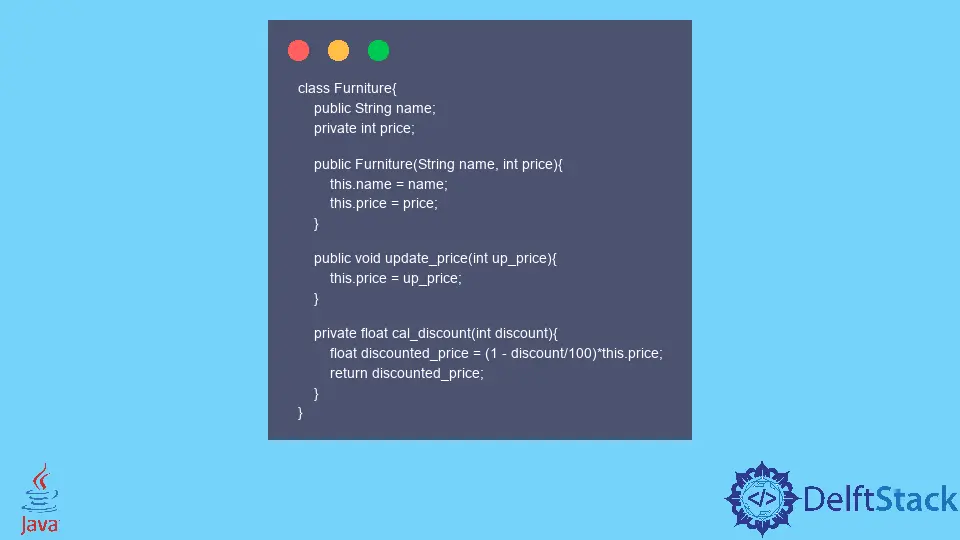
This tutorial introduces the reflection and how to use it in Java. Java has a reflection API feature that allows us to inspect and modify classes, interfaces, etc.
In this tutorial, we learn what reflection API is and its uses. Reflection in Java allows us to observe and alter classes, interfaces, constructors, methods, and fields during the runtime, even if we do not know the class name during the compile time.
Creating new objects, invoking methods, and getting/setting field values may all be done with reflection. Let’s understand with some examples.
We first need to import the following package to use the reflection API.
java.lang.reflect.*
It imports all the classes contained in the reflection API.
Class Object
After importing the package, we need to create a class object to use the reflection API methods.
Class exists in Java since it keeps track of all data about objects and classes during runtime. Reflection can be executed on the Class object.
A class object can be created in three ways. We will look into each method one by one.
But before that, let’s create a class that we will use throughout this tutorial.
class Furniture {
public String name;
private int price;
public Furniture(String name, int price) {
this.name = name;
this.price = price;
}
public void update_price(int up_price) {
this.price = up_price;
}
private float cal_discount(int discount) {
float discounted_price = (1 - discount / 100) * this.price;
return discounted_price;
}
}
Now, let’s create an object of class Class of the furniture class.
Class forName()
Method in Java
Class class provides a method forName()
to load object.
To use this method, we must know the name of the class upon which we want to reflect. We then pass the class name as the argument to the forName()
method.
Look at the code below:
Class class
= Class.forName("Furniture");
This method throws a ClassNotFoundException
if Java cannot find the class.
Class getClass()
Method in Java
We call this method on the object of the class upon which we want to reflect. This method returns the object’s class.
Look at the code below:
Furniture furniture = new Furniture("Chair", 8565);
Class f = furniture.getClass();
We can also use the .class
feature to get class objects for reflection.
// create a class object to relect on furniture
Class f = Furniture.class;
Useful Methods for Reflection in Java
The class object has the following three methods, which can be used to reflect upon the class.
getName()
: This method returns the name of the class.getModifiers()
: This method returns an integer representing the access modifier of the class. We can then convert this integer to String using theModifier.toString()
method.getSuperclass()
: This method returns the Superclass of the method.
Let’s see an example to understand these methods.
import java.lang.reflect.Modifier;
public class SimpleTesting {
public static void main(String[] args) {
// create an object of furniture class
Furniture furniture = new Furniture("Chair", 8565);
// create a class object to relect on furniture
Class f = furniture.getClass();
// get the name of the class
String class_name = f.getName();
System.out.println("The class name is " + class_name);
// get the class modifier
int f_modifier = f.getModifiers();
String f_mod = Modifier.toString(f_modifier);
System.out.println("The modifier of the class is " + f_mod);
// get the superclass of the class
Class f_superclass = f.getSuperclass();
System.out.println("The Superclass of the class is " + f_superclass.getName());
}
}
Output:
The class name is Furniture
The modifier of the class is
The Superclass of the class is java.lang.Object
Useful Methods for Reflection Upon Methods
Java provides a class called Method that deals with methods during reflection.
A method called getDeclaredMethods()
returns an array of all the methods defined in the class. The methods returned belong to the class Method.
The Method class contains several methods for retrieving information about the methods in a class. We will use the following methods of the method class:
Method.getName()
: This method returns the method’s name.Method.getModifiers()
: This method returns an integer representing the method’s access modifier.Method.getReturnType()
: This method returns the return type of the method.
Let’s see an example to understand these methods.
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
public class SimpleTesting {
public static void main(String[] args) {
// create an object of furniture class
Furniture furniture = new Furniture("Chair", 8565);
// create a class object to relect on furniture
Class f = furniture.getClass();
// using object f of Class to
// get all the declared methods of Furniture
Method[] f_methods = f.getDeclaredMethods();
for (Method f_method : f_methods) {
// get the name of the method
System.out.println("Method Name: " + f_method.getName());
// get the access modifier of methods
int f_modifier = f_method.getModifiers();
System.out.println("Modifier: " + Modifier.toString(f_modifier));
// get the return type of the methods
System.out.println("Return Types: " + f_method.getReturnType());
System.out.println(" ");
}
}
}
Output:
Method Name: update_price
Modifier: public
Return Types: void
Method Name: cal_discount
Modifier: private
Return Types: float
In the above example, we are trying to know about the methods defined in the Furniture class. We first have to create a Class object using the getClass()
method.
Useful Methods for Reflection Upon Fields
We can also inspect and change different fields of a class using the methods of the Field class. There is a method called.
class.getField
(<field name>)
This method takes one argument: the field’s name that we want to access and returns a Field object of the passed field. We can then use the Field class object to call various methods.
We will use the following methods of the Field class.
Field.set()
: This method sets the field’s value.Field.get()
: This method returns the value stored in the field.Field.getModifier()
: This method returns an integer representing the access modifier of the field.
Let’s see an example to understand these methods.
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
public class SimpleTesting {
public static void main(String[] args) throws Exception {
// create object of the furniture class
Furniture furniture = new Furniture("Chair", 8565);
// create a class object to relect on furniture
Class f = furniture.getClass();
// using object f of Class to
// acces the name field of Furniture
Field f_name = f.getField("name");
// get the value of the field
String f_name_value = (String) f_name.get(furniture);
System.out.println("Field value before changing: " + f_name_value);
// changing the field value
f_name.set(furniture, "Table");
// get the value of the field
f_name_value = (String) f_name.get(furniture);
System.out.println("Field value after changing: " + f_name_value);
// get the access modifier
int f_name_mod = f_name.getModifiers();
// convert the access modifier to String form
String f_name_modifier = Modifier.toString(f_name_mod);
System.out.println("Modifier: " + f_name_modifier);
System.out.println(" ");
}
}
Output:
Field value before changing: Chair
Field value after changing: Table
Modifier: public
Accessing a private field is similar except that we have to modify the accessibility of the private field by using the method setAccessible()
and passing true as its argument.
Look at the example code below; we are trying to access and modify the private field price of the Furniture class.
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
public class SimpleTesting {
public static void main(String[] args) throws Exception {
// create object of the furniture class
Furniture furniture = new Furniture("Chair", 8565);
// create a class object to reflect on furniture
Class f = furniture.getClass();
// using object f of Class to
// access the price field of Furniture
Field f_price = f.getDeclaredField("price");
// modifying the accessibility
f_price.setAccessible(true);
// get the value of the field
int f_price_value = (Integer) f_price.get(furniture);
System.out.println("Field value before changing: " + f_price_value);
// changing the field value
f_price.set(furniture, 453);
// get the value of the field
f_price_value = (Integer) f_price.get(furniture);
System.out.println("Field value after changing: " + f_price_value);
// get the access modifier
int f_price_mod = f_price.getModifiers();
// convert the access modifier to String form
String f_price_modifier = Modifier.toString(f_price_mod);
System.out.println("Modifier: " + f_price_modifier);
System.out.println(" ");
}
}
Output:
Field value before changing: 8565
Field value after changing: 453
Modifier: private
Conclusion
We have a sufficient understanding of reflection in Java after reading this article.
We also studied the classes and methods in Java utilized for reflection. We learned how to get the name of the class and its methods and fields.
Using the reflection API, we can quickly get information about the class.