How to Override the toString Method in Java
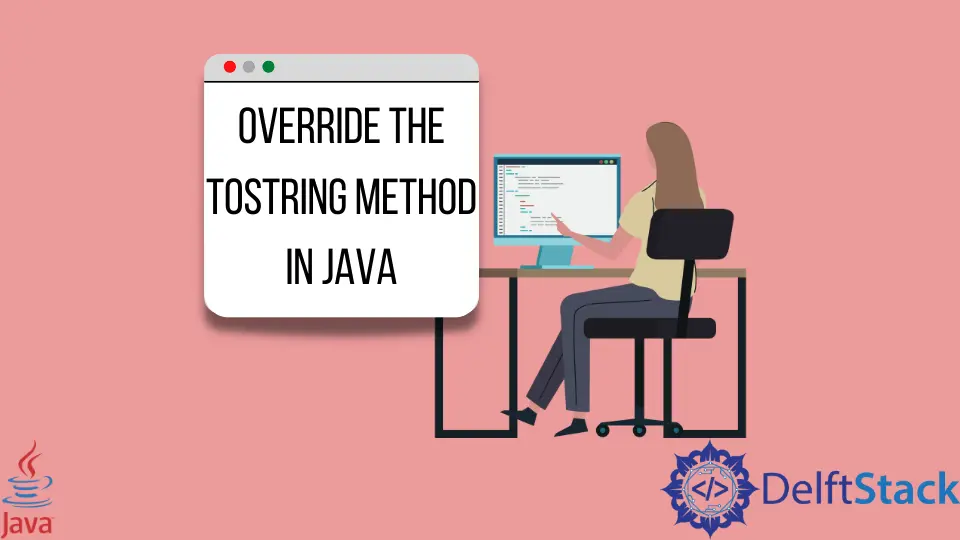
We often use strings in Java as they are an essential part of every program.
In this tutorial, we will see how we can override the tostring()
method in Java using an easy example to understand the concept better.
Override the toString()
Method Using the @Override
Annotation in Java
The toString()
method is a part of the Object
class which is a superclass of every class in Java. It means that every class has its own toString()
method. But we don’t get the desired output from the default toString()
method every time. It is when we have to override the method and customize it according to our needs.
To demonstrate how to override the toString()
method, we will see two examples, one without overriding the toString()
method and another that has the method overridden.
In the below example, we have a class ExampleClass
with three variables that are initialized in a constructor. When we create an object of the ExampleClass
class with the arguments passed and then print the object, we get the object that is not meaningful and not readable for humans. It is because, when print exampleClass
, its default toString()
is called. But we want to print the values of the variables as a string. To do this, we will override the toString()
method in the next example.
public class OverrideToString {
public static void main(String[] args) {
ExampleClass exampleClass = new ExampleClass("Alex", "Novel", 24);
System.out.println(exampleClass);
}
}
class ExampleClass {
String firstName;
String lastName;
int age;
ExampleClass(String firstName, String lastName, int age) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
}
}
Output:
ExampleClass@37bba400
In the following code, we take the same example as before, but this time we override the toString()
method using the @Override
annotation and write the method name as toString()
with a return type of String
. We can do this manually or we can use shortcuts that are included in many IDEs.
As the method returns a String, we have to construct it. We concatenate the variables with String to make a single String. At last, when we create an object of exampleClass
and print it, we get the output as a String with the values of the variables passed. It happens because we override the toString()
method to specify our own implementation.
public class OverrideToString {
public static void main(String[] args) {
ExampleClass exampleClass = new ExampleClass("Alex", "Novel", 24);
System.out.println(exampleClass);
}
}
class ExampleClass {
String firstName;
String lastName;
int age;
ExampleClass(String firstName, String lastName, int age) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
}
@Override
public String toString() {
return "firstName='" + firstName + '\'' + ", lastName='" + lastName + '\'' + ", age=" + age;
}
}
Output:
firstName='Alex', lastName='Novel', age=24
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn