@Override in Java
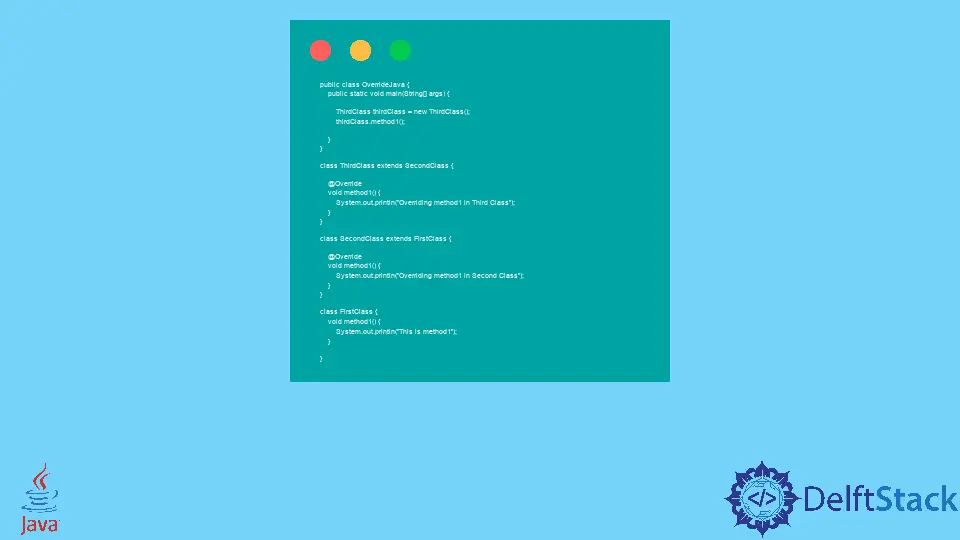
In this guide, we’ll have a deep dive into the topic of overriding and the @override
annotation in Java. Overriding is the concept where a child class has the same method as in its parent class. This concept is an example of runtime polymorphism.
Difference Between Overriding and Overloading in Java
Overriding is often confused with another similar term called overloading. Before going further, let us see the significant differences between them in the following table.
Method Overriding | Method Overloading |
---|---|
Methods should have the same name and signature | Methods must have the same name but different signature |
It is an example of runtime polymorphism | It is an example of compile-time polymorphism |
Return type of all the methods must be the same | Return type may or may not be the same |
Overriding happens between two or more classes | Overloading happens in the same class |
The parameters should be the same | The parameters should be different |
the @Override
Annotation in Java
The @Override
annotation tells the Java compiler that we want to override a method from the superclass. Although it is unnecessary to use @Override
whenever we want to implement it to a process, we recommend using it because we can make mistakes when creating the methods. For example, we might provide different parameters in the child class, which makes it overloading instead of overriding.
To overcome the mistake, we use @Override
above the method name in the child classes that tells the compiler that we want to override the method. If we make any mistake, the compiler will throw an error.
In the example below, we have four classes. The OverrideJava
class is where the main()
method is and where the methods of other classes will be called using their objects. Then we have three other classes in which the FirstClass
has a method called method1()
with a print message inside. Finally, the SecondClass
class extends the FirstClass
that uses the concept of inheritance. Here, we use the same method name and signature method1()
as its parent class like FirstClass
.
Notice that in the SecondClass
class, we use the @Override
annotation. Next, we extend the SecondClass
in the ThirdClass
class and use the method1()
method with the annotation. To check which method gets called, we create an object of ThirdClass
, which is the child class, and call method1()
. In the output, we observe that the method inside ThirdClass
is called because the child changed the inner implementation after the parent.
public class OverrideJava {
public static void main(String[] args) {
ThirdClass thirdClass = new ThirdClass();
thirdClass.method1();
}
}
class ThirdClass extends SecondClass {
@Override
void method1() {
System.out.println("Overriding method1 in Third Class");
}
}
class SecondClass extends FirstClass {
@Override
void method1() {
System.out.println("Overriding method1 in Second Class");
}
}
class FirstClass {
void method1() {
System.out.println("This is method1");
}
}
Output:
Overriding method1 in Third Class
If we want to call the parent class’s method1()
instead of the child class, we can use super.method1()
that calls its super class’s method1()
. The output shows that inside ThirdClass
first, its parent class that’s the SecondClass's
method is called, then its own implementation is called.
public class OverrideJava {
public static void main(String[] args) {
ThirdClass thirdClass = new ThirdClass();
thirdClass.method1();
}
}
class ThirdClass extends SecondClass {
@Override
void method1() {
super.method1();
System.out.println("Overriding method1 in Third Class");
}
}
class SecondClass extends FirstClass {
@Override
void method1() {
System.out.println("Overriding method1 in Second Class");
}
}
class FirstClass {
void method1() {
System.out.println("This is method1");
}
}
Output:
Overriding method1 in Second Class
Overriding method1 in Third Class
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn