How to Merge PDF in Java
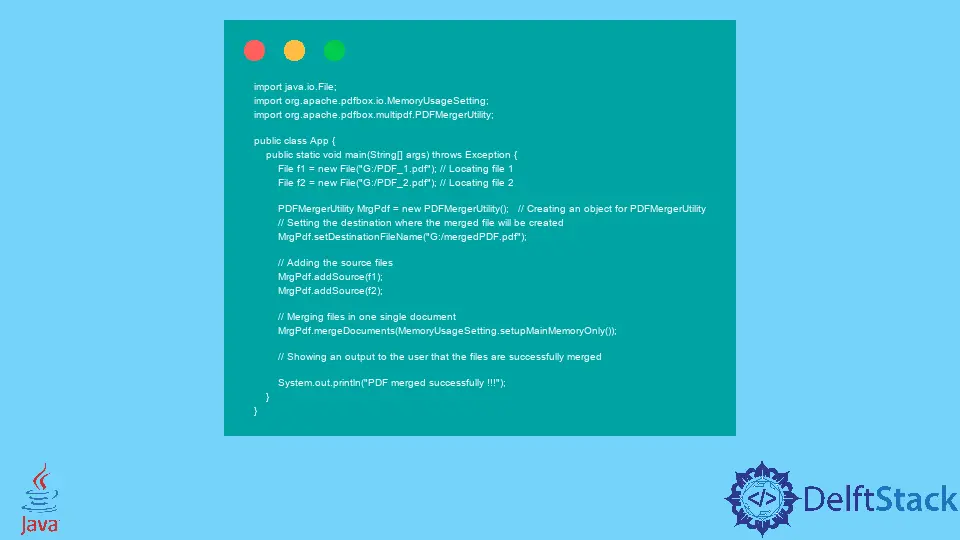
Merging PDF files in Java can be a straightforward task if you have the right tools and libraries at your disposal. Whether you’re working on a project that requires combining multiple documents into one or simply need to organize your PDF files, knowing how to merge PDFs programmatically can save you time and effort.
In this tutorial, we will explore various methods to merge PDF files using Java, focusing on popular libraries like Apache PDFBox and iText. By the end of this guide, you’ll have a clear understanding of how to efficiently combine PDFs in your Java applications.
Merging PDFs Using Apache PDFBox
Apache PDFBox is a powerful Java library that allows you to create, manipulate, and extract data from PDF documents. It’s open-source and widely used for PDF-related tasks. Merging PDFs with PDFBox is quite straightforward and requires just a few lines of code.
Here’s a simple example of how to merge PDF files using PDFBox:
import org.apache.pdfbox.multipdf.PDFMergerUtility;
import java.io.File;
public class MergePDFs {
public static void main(String[] args) {
PDFMergerUtility merger = new PDFMergerUtility();
merger.setDestinationFileName("merged.pdf");
File file1 = new File("file1.pdf");
File file2 = new File("file2.pdf");
merger.addSource(file1);
merger.addSource(file2);
try {
merger.mergeDocuments(null);
System.out.println("PDFs merged successfully!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
PDFs merged successfully!
In this code snippet, we first import the necessary classes from the PDFBox library. We create an instance of PDFMergerUtility
and set the destination file name for the merged PDF. Next, we specify the source PDF files we want to merge using the addSource
method. Finally, we call the mergeDocuments
method to perform the merge. Handling exceptions is also essential to catch any issues during the merging process.
Merging PDFs Using iText
iText is another robust library for PDF manipulation in Java. It is particularly known for its flexibility and powerful features. While iText is free for personal use, it has licensing requirements for commercial applications.
Here’s how you can merge PDF files using iText:
import com.itextpdf.kernel.pdf.PdfDocument;
import com.itextpdf.kernel.pdf.PdfReader;
import com.itextpdf.kernel.pdf.PdfWriter;
import java.io.File;
public class MergePDFs {
public static void main(String[] args) {
String dest = "merged.pdf";
try {
PdfDocument pdfDoc = new PdfDocument(new PdfWriter(dest));
for (String src : new String[]{"file1.pdf", "file2.pdf"}) {
PdfDocument srcDoc = new PdfDocument(new PdfReader(src));
srcDoc.copyPagesTo(1, srcDoc.getNumberOfPages(), pdfDoc);
srcDoc.close();
}
pdfDoc.close();
System.out.println("PDFs merged successfully!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
PDFs merged successfully!
In this example, we import the necessary iText classes and create a new PdfDocument
for the merged output. We loop through the source files, creating a PdfDocument
for each one and copying its pages into the destination document. Finally, we close both the source and destination documents to ensure that all resources are released. Exception handling is also included to manage any potential errors.
Conclusion
Merging PDF files in Java is a task that can be accomplished easily with the right libraries. Whether you choose Apache PDFBox or iText, both libraries offer reliable methods to combine multiple PDF documents into one. By following the examples provided in this tutorial, you can effectively integrate PDF merging capabilities into your Java applications, enhancing their functionality and user experience. As you continue to work with PDFs, exploring the full range of features these libraries offer will allow you to handle various PDF-related tasks with ease.
FAQ
-
What libraries can I use to merge PDFs in Java?
You can use libraries like Apache PDFBox and iText to merge PDF files in Java. -
Is iText free to use for commercial applications?
No, iText requires a commercial license for use in commercial applications, but it is free for personal use. -
Can I merge more than two PDF files at once?
Yes, both Apache PDFBox and iText allow you to merge multiple PDF files in a single operation. -
What is the output format after merging PDFs?
The output will be a single PDF file that contains all the pages from the source files. -
Do I need to handle exceptions while merging PDFs?
Yes, it is important to handle exceptions to manage any issues that may arise during the merging process.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn