Color Textfield Text in JavaFX
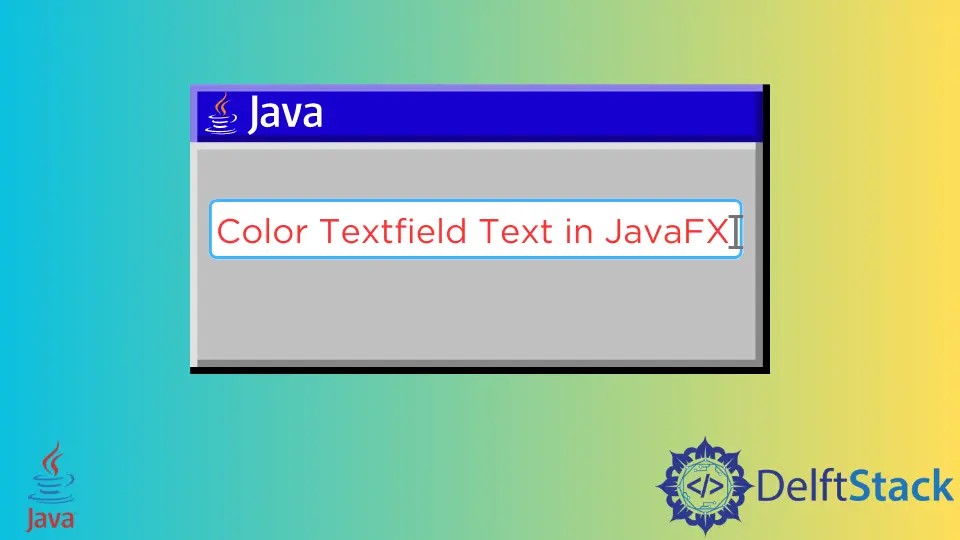
Textfield
is a basic element of the JavaFX UI component. Through this component, we can take user input to the system.
We can style this Textfield
by using a direct implementation of CSS property using the setStyle()
method. You can also include these CSS properties by providing an external CSS file.
In this article, we will see how we can create a Textfield
in JavaFX and provide color to the text of this Textfield
. We also see an example with explanations regarding this topic to make it easier to understand.
Color Textfield
Text in JavaFX
In our below example, we will create a Textfield
and set the color of its text to red. The code for our example will be like the below.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class FXTextfield extends Application {
public void start(Stage PrimaryStage) {
PrimaryStage.setTitle("TextField text color"); // Providing a title to our application
TextField txt = new TextField(); // Creating a text field
StackPane stkpn = new StackPane(); // Creating a stackpane
stkpn.getChildren().add(txt); // Adding text field to the stackpane
txt.setStyle("-fx-text-fill: red;"); // Setting the text color to red
Scene scene =
new Scene(stkpn, 200, 100); // Creating a scene with stackpane and dimention of 200x100
PrimaryStage.setScene(scene); // Creating the stage with the scene
PrimaryStage.show(); // Visualizing the stage
}
public static void main(String args[]) {
launch(args); // Launching the application
}
}
We have already commented on the purpose of each line. Now we discuss the core part of the topic here.
Through the line txt.setStyle("-fx-text-fill: red;");
, we set the CSS property -fx-text-fill
to be red
. We used the method setStyle()
.
After compiling the above example code and running it in your environment, you will get the below output.
Alternative Way to Change the Textfield
Text Color
JavaFX supports CSS that works with FXML. Now, when designing the User Interface with JavaFX GUI building tool like Scene Builder
provided by Oracle, you can easily define the text color with the CSS property while developing the UI.
Also, you can add a CSS file on which you can add the below properties.
-fx-text-fill: red;
You can use the code below to include your CSS file in your code directly.
scene.getStylesheets().add("YourCSS.css")
Remember, if your IDE doesn’t support the automatic inclusion of Libraries and Packages. Then you may need to manually include these necessary Libraries and Packages before compiling.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn