Weak Reference Class in Java
Sheeraz Gul
Oct 12, 2023
Java
Java Reference
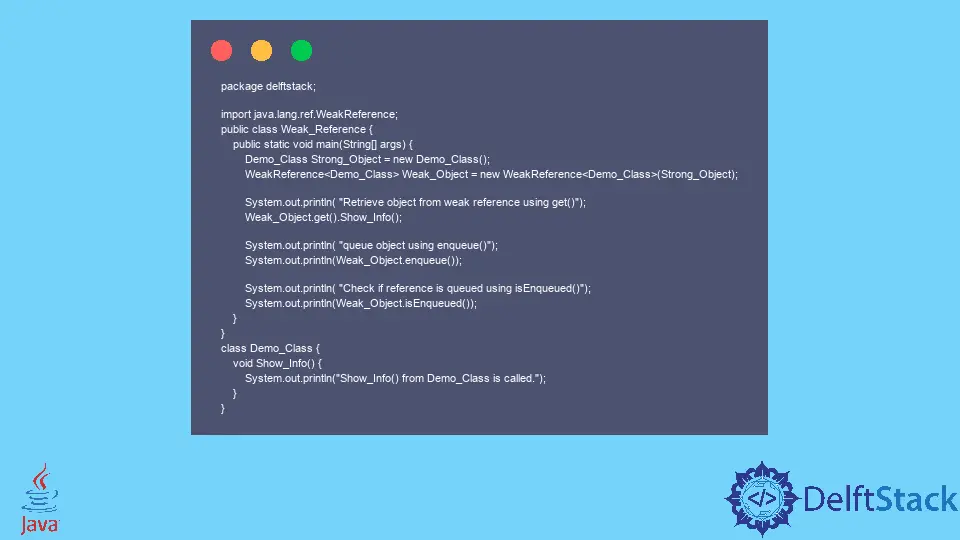
A weak reference is a reference that’s not strong enough to make an object remain in memory.
This tutorial demonstrates the use of weak references in Java.
Weak Reference Class in Java
In Java, the java.lang.ref.WeakReference
class leverages the garbage collectors to determine the reachability, so we don’t need to do it ourselves. The weak reference class in Java has the following methods.
Method | Description |
---|---|
get() |
This method returns the reference object’s referent. |
clear() |
This method is used to clear the reference object. |
enqueue() |
This method is used to add the reference object to the queue; the object needs to be registered with the queue. |
isEnqueued() |
This method tells if the reference object has been enqueued; it can be by the garbage collector or the program itself. |
First, let’s try a simple example to implement the Java weak reference class.
package delftstack;
import java.lang.ref.WeakReference;
public class Weak_Reference {
public static void main(String[] args) {
// creating a strong object
Demo_Class Strong_Object = new Demo_Class();
// creating a weak reference of demo_class
WeakReference<Demo_Class> Weak_Object = new WeakReference<>(Strong_Object);
System.out.println("Call the show info function using the strong object");
Strong_Object.Show_Info();
System.out.println("Setting the object to null");
Strong_Object = null;
Strong_Object = Weak_Object.get();
System.out.println("Call the show info function using the weak object");
Strong_Object.Show_Info();
}
}
class Demo_Class {
void Show_Info() {
System.out.println(">>This is the info from demo class<<");
}
}
The code above creates a strong object of Demo_Class
and a weak object of the same class and calls the Show_Info
function. See output:
Call the show info function using the strong object
>>This is the info from demo class<<
Setting the object to null
Call the show info function using the weak object
>>This is the info from demo class<<
Now let’s try an example with the above methods.
package delftstack;
import java.lang.ref.WeakReference;
public class Weak_Reference {
public static void main(String[] args) {
Demo_Class Strong_Object = new Demo_Class();
WeakReference<Demo_Class> Weak_Object = new WeakReference<Demo_Class>(Strong_Object);
System.out.println("Retrieve object from weak reference using get()");
Weak_Object.get().Show_Info();
System.out.println("queue object using enqueue()");
System.out.println(Weak_Object.enqueue());
System.out.println("Check if reference is queued using isEnqueued()");
System.out.println(Weak_Object.isEnqueued());
}
}
class Demo_Class {
void Show_Info() {
System.out.println("Show_Info() from Demo_Class is called.");
}
}