Java - Use Nullable Annotation in Method Argument
- Understanding the @Nullable Annotation
- Applying @Nullable in Method Arguments
- Benefits of Using @Nullable
- Conclusion
- FAQ
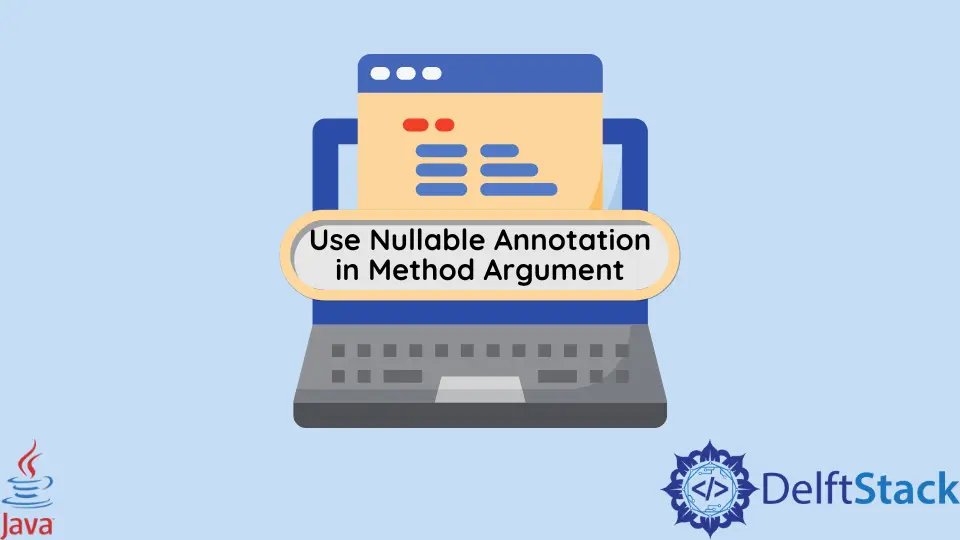
In the world of software development, ensuring code quality and preventing errors is paramount. One effective way to achieve this in Java is by using the @Nullable
annotation. This annotation is a powerful tool that helps developers indicate that a method argument can accept a null value.
In this tutorial, we will delve into the significance of the @Nullable
annotation, how it can be applied to method arguments, and the benefits it brings to code readability and maintainability. Whether you’re a seasoned Java developer or just starting out, understanding how to use this annotation effectively can enhance your coding practices and reduce the likelihood of encountering null pointer exceptions.
Understanding the @Nullable Annotation
The @Nullable
annotation is part of the Java ecosystem, often provided by libraries like JetBrains’ annotations or the Javax annotations package. By marking a method parameter or return type with @Nullable
, you are explicitly stating that the value can be null. This provides valuable information to both the compiler and other developers who may work with your code later.
Using @Nullable
can lead to better code practices, as it encourages developers to think critically about null values. It also aids in static analysis, allowing tools to catch potential null-related errors before runtime. In this section, we will explore how to effectively use the @Nullable
annotation within method arguments.
Applying @Nullable in Method Arguments
To utilize the @Nullable
annotation in method arguments, you must first ensure that you have the necessary library in your project. If you’re using Maven, you can add the JetBrains annotations dependency to your pom.xml
:
<dependency>
<groupId>org.jetbrains</groupId>
<artifactId>annotations</artifactId>
<version>23.0.0</version>
</dependency>
Once you have the annotation library set up, you can start applying the @Nullable
annotation to your method arguments. Here’s a simple example:
import org.jetbrains.annotations.Nullable;
public class User {
private String name;
public User(@Nullable String name) {
this.name = name;
}
public void printName() {
if (name != null) {
System.out.println("User name: " + name);
} else {
System.out.println("User name is not provided.");
}
}
}
In the code above, the constructor of the User
class accepts a String
that can be null. The printName
method checks for null before attempting to print the name. This simple check prevents potential null pointer exceptions and makes the code more robust.
Output:
User name is not provided.
By using the @Nullable
annotation, you clearly communicate to anyone reading the code that the name
parameter can be null. This encourages defensive programming practices, as developers are more likely to handle null cases appropriately.
Benefits of Using @Nullable
Implementing the @Nullable
annotation comes with several advantages. First and foremost, it improves code readability. When other developers see the annotation, they immediately understand that null values are acceptable. This clarity helps in maintaining the codebase over time.
Moreover, the @Nullable
annotation enhances static analysis capabilities. Tools like IntelliJ IDEA can provide warnings or suggestions based on the annotation, helping to catch potential null pointer exceptions during development rather than at runtime. This proactive approach significantly reduces debugging time and enhances overall code quality.
Additionally, using @Nullable
promotes better API design. When creating libraries or frameworks, clear documentation regarding which parameters can be null helps users of your API to use it correctly without running into unexpected null-related issues.
In summary, the @Nullable
annotation not only aids in preventing runtime errors but also fosters better coding practices and clearer communication among developers.
Conclusion
Incorporating the @Nullable
annotation into your Java methods is a straightforward yet powerful practice that can significantly improve your code quality. By clearly indicating which method arguments can accept null values, you enhance code readability, facilitate better static analysis, and ultimately reduce the chances of runtime errors. As you continue to develop your Java skills, remember the importance of using annotations like @Nullable
to write clearer, more maintainable code. Embrace this practice, and you’ll find that your coding experience becomes smoother and more efficient.
FAQ
-
What is the purpose of the @Nullable annotation?
The @Nullable annotation indicates that a method argument or return type can accept a null value, promoting better code practices and preventing null pointer exceptions. -
How do I use the @Nullable annotation in my Java project?
You can use the @Nullable annotation by including a library like JetBrains annotations in your project and applying the annotation to your method parameters or return types. -
What are the benefits of using @Nullable in Java?
The benefits include improved code readability, enhanced static analysis, better API design, and reduced chances of encountering null pointer exceptions. -
Can I use @Nullable with other annotations?
Yes, the @Nullable annotation can be used alongside other annotations, such as @NotNull, to provide clear documentation on the expected behavior of method parameters and return values. -
Are there any alternatives to the @Nullable annotation?
Alternatives include using Optionalfrom Java 8, which allows for a more explicit handling of optional values without relying on nulls.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn