How to Unzip Files in Java
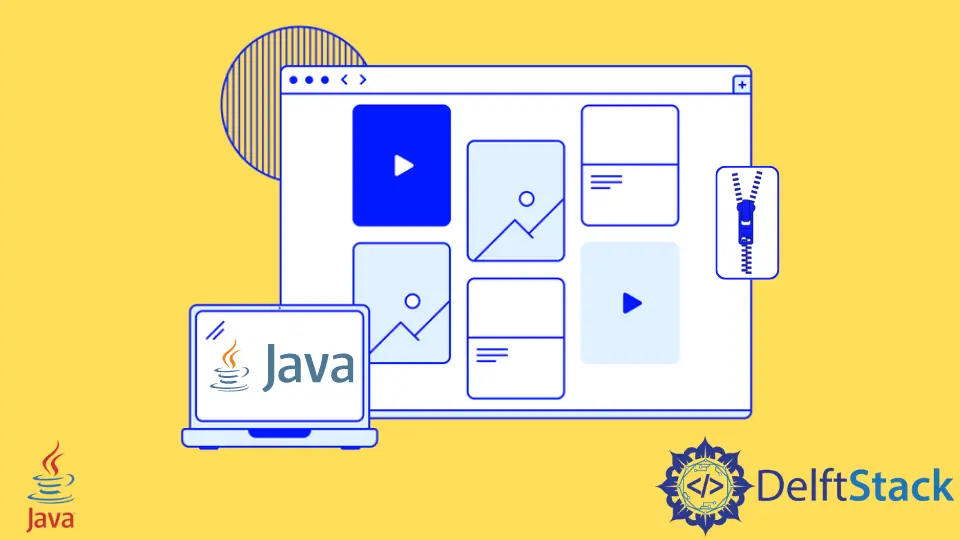
We can use the built-in Zip
API in Java to extract a zip file.
This tutorial demonstrates how to extract a zip file in Java.
Unzip Files in Java
The java.util.zip
is used to unzip the zip files in Java. The ZipInputStream
is the main class used to read the zip files and extract them.
Follow the steps below to extract zip files in Java.
-
Read the zip file using
ZipInputStream
andFileInputStream
. -
Read the entries using
getNextEntry()
method. -
Now read the binary data using the
read()
method with bytes. -
Close the entry using
closeEntry()
method. -
Finally, close the zip file.
We created a function to take the input and destination path and extract the files to implement these steps. The zip file is below.
Let’s implement the above method in Java to extract the zip file shown in the picture.
package delftstack;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class Java_Unzip {
private static final int BUFFER_SIZE = 4096;
public static void unzip(String ZipFilePath, String DestFilePath) throws IOException {
File Destination_Directory = new File(DestFilePath);
if (!Destination_Directory.exists()) {
Destination_Directory.mkdir();
}
ZipInputStream Zip_Input_Stream = new ZipInputStream(new FileInputStream(ZipFilePath));
ZipEntry Zip_Entry = Zip_Input_Stream.getNextEntry();
while (Zip_Entry != null) {
String File_Path = DestFilePath + File.separator + Zip_Entry.getName();
if (!Zip_Entry.isDirectory()) {
extractFile(Zip_Input_Stream, File_Path);
} else {
File directory = new File(File_Path);
directory.mkdirs();
}
Zip_Input_Stream.closeEntry();
Zip_Entry = Zip_Input_Stream.getNextEntry();
}
Zip_Input_Stream.close();
}
private static void extractFile(ZipInputStream Zip_Input_Stream, String File_Path)
throws IOException {
BufferedOutputStream Buffered_Output_Stream =
new BufferedOutputStream(new FileOutputStream(File_Path));
byte[] Bytes = new byte[BUFFER_SIZE];
int Read_Byte = 0;
while ((Read_Byte = Zip_Input_Stream.read(Bytes)) != -1) {
Buffered_Output_Stream.write(Bytes, 0, Read_Byte);
}
Buffered_Output_Stream.close();
}
public static void main(String[] args) throws IOException {
String ZipFilePath = "delftstack.zip";
String DestFilePath = "C:\\Users\\Sheeraz\\eclipse-workspace\\Demos";
unzip(ZipFilePath, DestFilePath);
System.out.println("Zip File extracted Successfully");
}
}
The output for the code above is below.
Zip File extracted Successfully
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook