Unchecked Cast in Java
-
What Is the
Unchecked Cast
Warning in Java -
Understanding
Unchecked Cast
Warnings in Java -
Preventing
Unchecked Cast
Warnings -
Best Practices for Preventing
Unchecked Cast
Warnings in Java - Conclusion
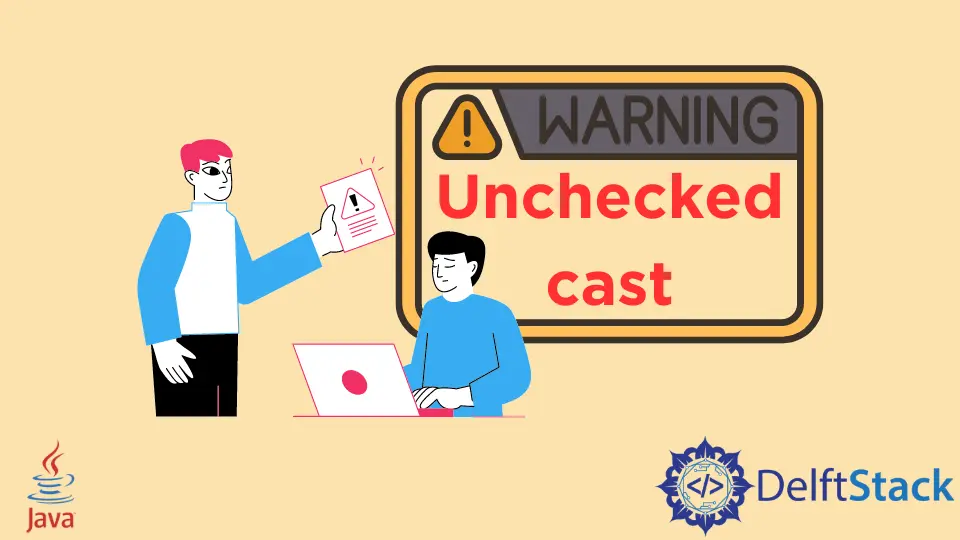
Java is a programming language that enforces type safety, which means that we should always specify the type of data that we are going to store or use and cannot store incompatible types in them.
Discover how to prevent unchecked cast
warnings in Java. Explore the causes, solutions, and best practices for ensuring type safety and reliability in your code.
What Is the Unchecked Cast
Warning in Java
An unchecked cast
warning in Java occurs when the compiler cannot ensure type safety during a casting operation. It warns the developer about potential runtime errors, such as ClassCastException
, that may occur due to type mismatches.
Unchecked cast
warnings typically arise when casting from a generic type to a specific type or when casting to a parameterized type without proper type checking. Addressing these warnings is crucial to ensure code reliability and prevent unexpected runtime errors.
Understanding Unchecked Cast
Warnings in Java
In Java programming, unchecked cast
warnings are common occurrences that indicate potential type safety issues in your code. Let’s delve into two significant causes of unchecked cast
warnings:
Cause | Description |
---|---|
Direct Casting from Raw Types | When you directly cast from raw types to parameterized types, it can lead to unchecked cast warnings. This occurs when the compiler cannot ensure type safety during the cast operation. |
Casting Without Type Checking | Performing unchecked casts without proper type checking can also trigger unchecked cast warnings. This happens when you attempt to cast an object to a parameterized type without verifying its actual type, potentially leading to runtime errors. |
Understanding these causes is crucial for maintaining code quality and preventing unexpected runtime errors. Let’s explore solutions to address unchecked cast
warnings in Java code.
Preventing Unchecked Cast
Warnings
In Java programming, unchecked cast
warnings signify potential type safety issues that can lead to runtime errors if not addressed properly. Direct casting from raw types and casting without type checking are common scenarios where unchecked cast
warnings occur.
Understanding how to prevent these warnings is crucial for maintaining code integrity and preventing unexpected runtime errors. In this section, we’ll explore effective techniques to prevent unchecked cast
warnings in Java by addressing direct casting from raw types and casting without proper type checking.
Code Example:
import java.util.ArrayList;
import java.util.List;
public class UncheckedCastExample {
public static void main(String[] args) {
// Direct casting from raw types
List rawList = new ArrayList();
rawList.add("Hello");
// List<String> stringList1 = (List<String>) rawList; // Direct cast from raw type
List<String> stringList1 = new ArrayList<>(rawList); // Corrected code: Create a new ArrayList
// Casting without type checking
Object obj = "World";
// List<String> stringList2 = (List<String>) obj; // Casting without type checking
List<String> stringList2 = new ArrayList<>(); // Create a new ArrayList
stringList2.add((String) obj); // Type check and cast before adding to the list
// Output for direct casting from raw types
System.out.println("Output for direct casting from raw types: " + stringList1);
// Output for casting without type checking
System.out.println("Output for casting without type checking: " + stringList2);
}
}
The code example provided illustrates two common scenarios in Java where unchecked cast
warnings can arise: direct casting from raw types and casting without proper type checking.
In the first scenario, a raw ArrayList
named rawList
is instantiated, and a String
(Hello
) is added to it. Initially, an attempt is made to directly cast rawList
to a parameterized type List<String>
.
Such direct casting from a raw type can trigger unchecked cast
warnings as it bypasses type safety checks. To address this, we adopt a safer approach by creating a new ArrayList
, stringList1
.
By passing rawList
as a parameter to its constructor, we ensure that stringList1
maintains the correct generic type. This action effectively prevents unchecked cast
warnings, ensuring type safety throughout the code.
In the second scenario, an Object
(obj
) is assigned the value World
. There is an initial attempt to cast obj
directly to List<String>
without performing proper type checking.
Such casting without type checking can lead to unchecked cast
warnings as it lacks verification of type compatibility. To mitigate this risk, we instantiate a new ArrayList
(stringList2
) and add obj
after performing necessary type checking and casting.
By ensuring that the object being added to stringList2
is indeed a String
type, we maintain type safety and avoid unchecked cast
warnings.
By employing these techniques, developers can effectively prevent unchecked cast
warnings in their Java code, thereby enhancing type safety and reducing the likelihood of unexpected runtime errors.
The code will produce the following output:
By following the demonstrated techniques, developers can effectively prevent unchecked cast
warnings in Java code, ensuring type safety and reducing the risk of unexpected runtime errors. Understanding and implementing these practices are essential for maintaining code reliability and integrity in Java applications.
Best Practices for Preventing Unchecked Cast
Warnings in Java
Use Generics Consistently
Utilize parameterized types (generics) consistently throughout your code to ensure type safety and prevent unchecked cast
warnings.
Perform Type Checking Before Casting
Always perform proper type checking before casting objects to parameterized types to ensure compatibility and prevent unchecked cast
warnings.
Avoid Raw Types
Minimize the use of raw types and prefer parameterized types whenever possible to maintain type safety and prevent unchecked cast
warnings.
Consider Type Inference
Leverage type inference where applicable to automatically determine generic types and reduce the likelihood of unchecked cast
warnings.
Review and Test Code
Regularly review and test your code to identify and address any instances of unchecked cast
warnings, ensuring robustness and reliability.
Conclusion
Unchecked cast
warnings in Java signal potential type safety issues arising from direct casting from raw types and casting without proper type checking. The causes include direct casting from raw types and casting without type checking.
Solutions involve creating new parameterized types instead of directly casting from raw types and performing type checking before casting. Best practices include using generics consistently, avoiding raw types, considering type inference, and regularly reviewing and testing code.
By implementing these strategies, developers can ensure type safety and prevent unchecked cast
warnings in Java programs.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn