JavaTuples in Java
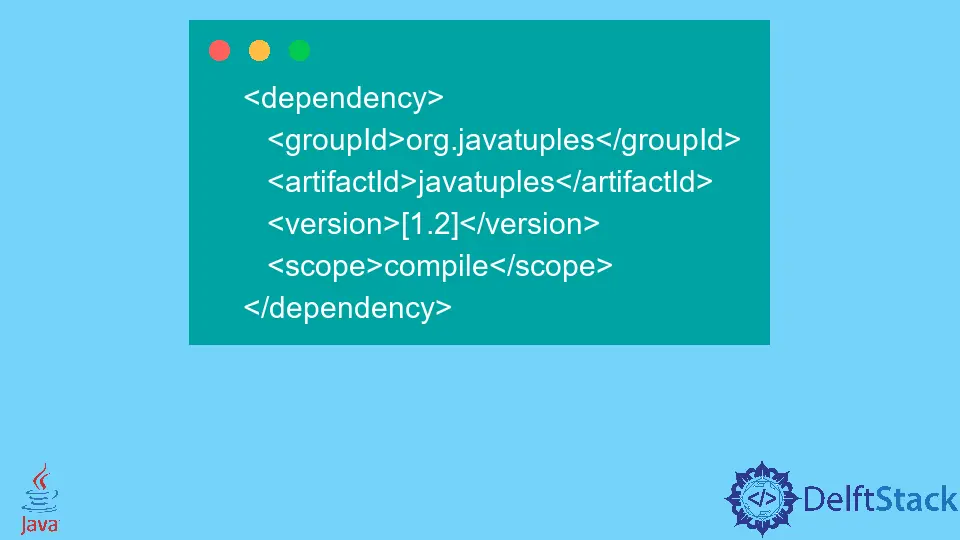
A tuple is a data structure that can store objects without requiring any relation between them but have a mutual motive. Java does not support the tuple data structure, but we can use a JavaTuples
library.
Features of JavaTuples
- The Tuples are Typesafe
- They are immutable
- We can iterate through tuple values
- They are serializable
- They implement
Comparable<Tuple>
- Implements methods like
equals()
,hashCode()
, andtoString()
Tuple Classes in JavaTuples
We can use up to ten elements in tuple classes. There are special subclasses for every number of elements in JavaTuples
which are mentioned in the table below:
No. of Elements (Tuple Size) | Class Name | Example |
---|---|---|
One | Unit |
Unit<1> |
Two | Pair |
Pair<1,2> |
Three | Triplet |
Triplet<1,2,3> |
Four | Quartet |
Quartet<1,2,3,4> |
Five | Quintet |
Quintet<1,2,3,4,5> |
Six | Sextet |
Sextet<1,2,3,4,5,6> |
Seven | Septet |
Septet<1,2,3,4,5,6,7> |
Eight | Octet |
Octet<1,2,3,4,5,6,7,8> |
Nine | Ennead |
Ennead<1,2,3,4,5,6,7,8,9> |
Ten | Decade |
Decade<1,2,3,4,5,6,7,8,9,10> |
Methods in JavaTuples
The Tuple
class provides various methods to perform operations.
Method | Description |
---|---|
equals() |
It is used to override the default implementation of the equals method of the Object class |
hashCode() |
Returns a hash code for each object. |
toString() |
Returns a string representation |
getSize() |
Returns the size of the tuples |
getValue() |
Returns a value at a specified position in a tuple |
indexOf() |
Returns the index of the asked sub string in a given string. |
contains() |
Check is a element exists in the tuple |
containsAll() |
Checks if all the elements of an list or array exists in the tuple or not |
iterator() |
Returns an iterator |
lastIndexOf() |
Returns the last index of the asked substring in a given string. |
toArray() |
Tuple to Array |
toList() |
Tuple to List |
Implement Tuple in Java
We import the following maven-based dependency to use the JavaTuples
library.
<dependency>
<groupId>org.javatuples</groupId>
<artifactId>javatuples</artifactId>
<version>[1.2]</version>
<scope>compile</scope>
</dependency>
In the example, we implement the various tuple classes in the above table and get their values by printing them. We provide different data types in the tuples like String
, Integer
, Boolean
, etc.
We also create a class DummyClass
and pass its object in the tuples if it throws an error.
import org.javatuples.*;
public class JavaTuplesExample {
public static void main(String[] args) {
Unit<String> unitTuple = new Unit<>("First Tuple Element");
Pair<String, Integer> pairTuple = new Pair<>("First Tuple Element", 2);
Triplet<String, Integer, Boolean> tripletTuple = new Triplet<>("First Tuple Element", 2, true);
Quartet<String, Integer, Boolean, String> quartetTuple =
new Quartet<>("First Tuple Element", 2, true, "Fourth Tuple Element");
Quintet<String, Integer, Boolean, String, Integer> quintetTuple =
new Quintet<>("First Tuple Element", 2, true, "Fourth Tuple Element", 5);
Sextet<String, Integer, Boolean, String, Integer, DummyClass> sextetTuple =
new Sextet<>("First Tuple Element", 2, true, "Fourth Tuple Element", 5, new DummyClass());
Septet<String, Integer, Boolean, String, Integer, DummyClass, Integer> septetTuple =
new Septet<>(
"First Tuple Element", 2, true, "Fourth Tuple Element", 5, new DummyClass(), 7);
Octet<String, Integer, Boolean, String, Integer, DummyClass, Integer, String> octetTuple =
new Octet<>("First Tuple Element", 2, true, "Fourth Tuple Element", 5, new DummyClass(), 7,
"Eight Tuple Element");
Ennead<String, Integer, Boolean, String, Integer, DummyClass, Integer, String, Integer>
enneadTuple = new Ennead<>("First Tuple Element", 2, true, "Fourth Tuple Element", 5,
new DummyClass(), 7, "Eight Tuple Element", 9);
Decade<String, Integer, Boolean, String, Integer, DummyClass, Integer, String, Integer, String>
decadeTuple = new Decade<>("First Tuple Element", 2, true, "Fourth Tuple Element", 5,
new DummyClass(), 7, "Eight Tuple Element", 9, "Tenth Tuple Element");
System.out.println(unitTuple);
System.out.println(pairTuple);
System.out.println(tripletTuple);
System.out.println(quartetTuple);
System.out.println(quintetTuple);
System.out.println(sextetTuple);
System.out.println(septetTuple);
System.out.println(octetTuple);
System.out.println(enneadTuple);
System.out.println(decadeTuple);
}
}
class DummyClass {}
Output:
[First Tuple Element]
[First Tuple Element, 2]
[First Tuple Element, 2, true]
[First Tuple Element, 2, true, Fourth Tuple Element]
[First Tuple Element, 2, true, Fourth Tuple Element, 5]
[First Tuple Element, 2, true, Fourth Tuple Element, 5, DummyClass@254989ff]
[First Tuple Element, 2, true, Fourth Tuple Element, 5, DummyClass@5d099f62, 7]
[First Tuple Element, 2, true, Fourth Tuple Element, 5, DummyClass@37f8bb67, 7, Eight Tuple Element]
[First Tuple Element, 2, true, Fourth Tuple Element, 5, DummyClass@49c2faae, 7, Eight Tuple Element, 9]
[First Tuple Element, 2, true, Fourth Tuple Element, 5, DummyClass@20ad9418, 7, Eight Tuple Element, 9, Tenth Tuple Element]
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn