How to Render LaTeX in Java
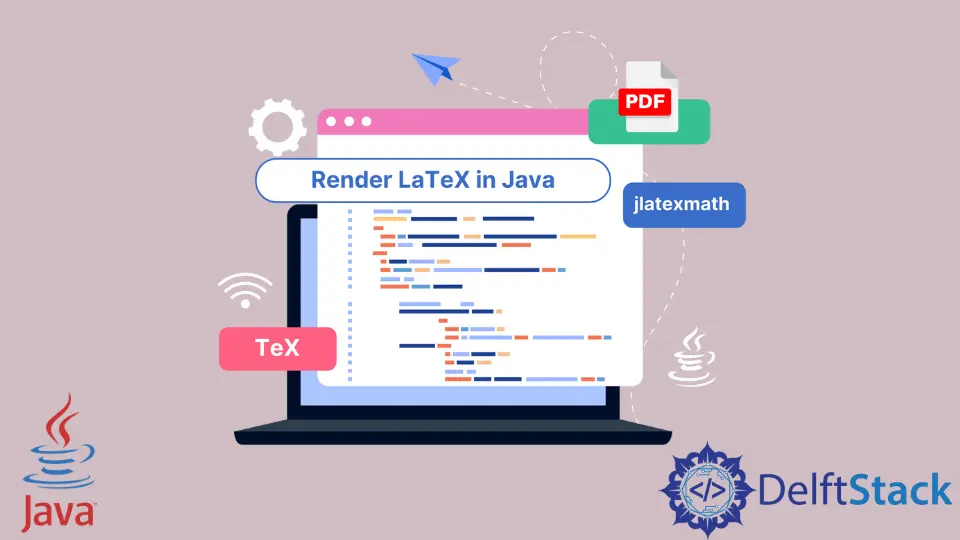
This tutorial will demonstrate how to execute TeX and get output in Java.
Use JLaTeXMath to Render LaTeX in Java
Import jlatexmath as a maven project in your eclipse or IDE, and use the examples and run your LaTeX in Java. Make sure the maven dependencies are already installed in your IDE.
Example:
package org.scilab.forge.jlatexmath.examples.export;
import java.io.IOException;
// This Class is used to render the latex
public class JAVA_TEX {
public static void main(String[] args) {
String demo_latex = "\\begin{array}{|c|l|||r|c|}";
demo_latex += "\\hline";
demo_latex +=
"\\text{Matrix}&\\multicolumn{2}{|c|}{\\text{Multicolumns}}&\\text{Font sizes commands}\\cr";
demo_latex += "\\hline";
demo_latex +=
"\\begin{pmatrix}\\alpha_{11}&\\cdots&\\alpha_{1n}\\cr\\hdotsfor{3}\\cr\\alpha_{n1}&\\cdots&\\alpha_{nn}\\end{pmatrix}&\\Large \\text{Large Right}&\\small \\text{small Left}&\\tiny \\text{tiny Tiny}\\cr";
demo_latex += "\\hline";
demo_latex += "\\multicolumn{4}{|c|}{\\Huge \\text{Huge Multicolumns}}\\cr";
demo_latex += "\\hline";
demo_latex += "\\end{array}";
try {
Convert.toSVG(demo_latex, "demo_latex.svg", false);
Convert.toSVG(demo_latex, "demo_latex_shaped.svg", true);
Convert.SVGTo("demo_latex.svg", "demo_latex.pdf", Convert.PDF);
Convert.SVGTo("demo_latex_shaped.svg", "demo_latex_shaped.pdf", Convert.PDF);
Convert.SVGTo("demo_latex.svg", "demo_latex.ps", Convert.PS);
Convert.SVGTo("demo_latex.svg", "demo_latex.eps", Convert.EPS);
} catch (IOException exception) {
throw new RuntimeException(exception);
}
}
}
Console output:
transcoding target/demo_latex.svg
Mar 08, 2022 12:36:46 PM org.apache.fop.fonts.truetype.TTFFile guessVerticalMetricsFromGlyphBBox
WARNING: capHeight value could not be determined. The font may not work as expected.
Mar 08, 2022 12:36:46 PM org.apache.fop.fonts.truetype.TTFFile guessVerticalMetricsFromGlyphBBox
WARNING: xHeight value could not be determined. The font may not work as expected.
transcoding target/demo_latex_shaped.svg
transcoding target/demo_latex.svg
transcoding target/demo_latex.svg
The code above will execute the LaTeX array syntax and generate output in .ps
, .svg
, and .pdf
.
Some warnings may occur, but that can be ignored.
Files output:
The .ps
, .svg
, and .pdf
files have the same written output below.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook