How to Use Java Synchronized Block for Class
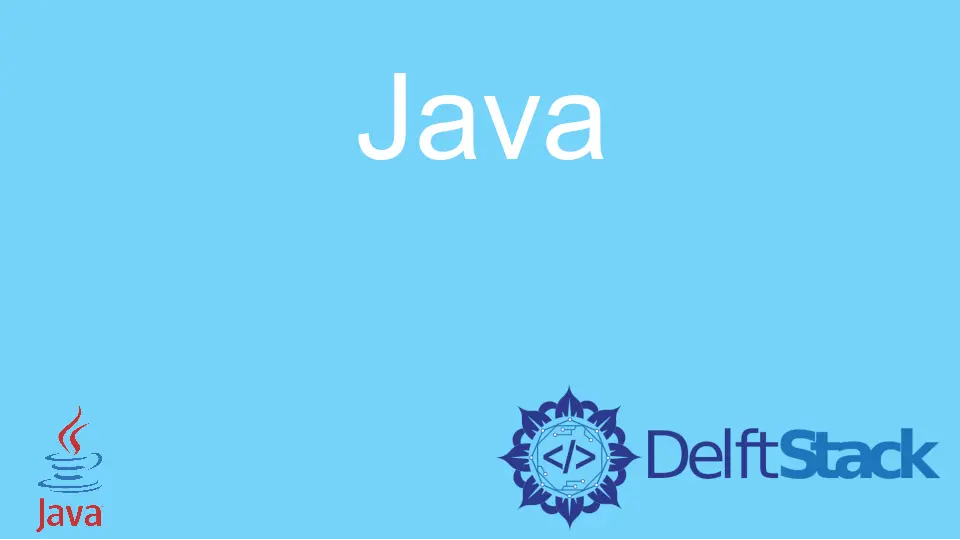
In Java, synchronization is managing multiple threads accessing resources that could be shared. Synchronization in Java is used so that threads can reliably communicate with each other, and it is also considered the best option for a single thread to access shared resources.
The synchronized
keyword is used to mark synchronized blocks. On some objects, Java synchronization block is applicable.
Use Java Synchronized Block for Class
If a synchronized block for the same objects has multiple threads, it would only allow one thread to enter the block and execute only one thread at a time. All other threads would remain blocked.
Let’s explain the Java synchronization block for class, along with examples.
Example Code:
class Block {
// synchronized block starts from here
void printBlock(int b) {
synchronized (this) {
System.out.println("The block output is: ");
for (int j = 1; j <= 4; j++) {
System.out.println(b * j);
// handles exception
try {
Thread.sleep(500);
} catch (Exception e) {
System.out.println(e);
}
}
}
} // ending
}
class S1 extends Thread {
Block s;
S1(Block s) {
this.s = s;
}
public void run() {
s.printBlock(10);
}
}
class S2 extends Thread {
Block s;
S2(Block s) {
this.s = s;
}
public void run() {
s.printBlock(200);
}
}
class SyncBlock {
// main method
public static void main(String args[]) {
// object creation
Block obj = new Block();
S1 s1 = new S1(obj);
S2 s2 = new S2(obj);
// run the threads s1 and s2
s1.run();
s2.run();
}
}
Output:
The block output is:
10
20
30
40
The block output is:
200
400
600
800
In the above example, we have two threads, S1
and S2
. Both threads have a printBlock
method that helps them call the synchronized
methods.
The S1
thread input value is 10, and for S2
, it is 200. Then, the block output prints between the S1
and S2
threads output.
Example Code:
import java.util.*;
class synchronize {
String title = "";
public int c = 0;
public void result(String s, List<String> li) {
// only one thread is allowed to change the title simultaneously.
synchronized (this) {
title = s;
c++;
}
li.add(s);
}
}
class SyncBlock {
// main method
public static void main(String[] args) {
// for synchronize class, we have created an object
synchronize ob = new synchronize();
// we have created a list here
List<String> li = new ArrayList<String>();
// for calling the method, a string is passed to it
ob.result("Sprint Planning", li);
System.out.println(ob.title);
}
}
Output:
Sprint Planning
In the above example, a class synchronization has been created. In this class, we’ve used the synchronized
method.
A string is passed as an input value for calling the method. i.e., Sprint Planning
.
When we run the above program, we get Sprint Planning
as an output.
Conclusion
There are many ways to discuss synchronization blocks in Java, but in this article, we have explained synchronization, synchronization blocks, and how it works.
If we have multiple threads
for an object at a time, using a synchronization block, we can only execute one thread at a time. The other threads wouldn’t be allowed to enter the block until the thread inside the block is executed successfully.
So, the synchronized block class in Java ensures that, for instance, there should be only one thread
in the block at a time.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn